First, we make sure that XAMPP Server is installed on our machine. Once it’s installed, let’s begin by first opening Xampp application. We have the following services running when we open Xampp application:
- MySQL Database
- Apache Web Server
Now we can navigate over to localhost/phpmyadmin and create a database and table.
Click on New and then type in the name of Database. We are going to name our database SampleDB. Once complete, click the Create button.
Next, we can create the table we want to use named SampleTable
. Make sure to set the number of columns (In this example we set number of columns to 5). Once complete, click the Create
button.
Now that our Database and Sample table is created, we can enter our columns and click on save.
Make sure enter the correct data type for each column.
- first_name
- last_name
- gender
- address
Create the html form
Now it’s time to create our HTML form. We will be using this form to send the data to our database.
Filename: index.php
<!DOCTYPE html>
<head>
<title>Sample Form</title>
</head>
<body>
<center>
<h1>Storing Form data in Database</h1>
<form action=”insert.php” method=”post”>
<p>
<label for=”firstName”>First Name:</label>
<input type=”text” name=”first_name” id=”firstName”>
</p>
<p>
<label for=”lastName”>Last Name:</label>
<input type=”text” name=”last_name” id=”lastName”>
</p>
<p>
<label for=”Gender”>Gender:</label>
<input type=”text” name=”gender” id=”Gender”>
</p>
<p>
<label for=”Address”>Address:</label>
<input type=”text” name=”address” id=”Address”>
</p>
<p>
<label for=”emailAddress”>Email Address:</label>
<input type=”text” name=”email” id=”emailAddress”>
</p>
<input type=”submit” value=”Submit”>
</form>
</center>
</body>
</html>
Create the php script
Now we need to create our PHP script. This is the script that will process our form data and insert it into our database.
Filename: insert.php
<!DOCTYPE html>
<html>
<head>
<title>Insert Page page</title>
</head>
<body>
<center>
<?php
// servername => localhost
// username => root
// password => empty
// database name => sampledb
$conn = mysqli_connect(“localhost”, “root”, “”, “sampledb”);
// Check connection
if($conn === false){
die(“ERROR: Could not connect. “
. mysqli_connect_error());
}
// Taking all 5 values from the form data(input)
$first_name = $_REQUEST[‘first_name’];
$last_name = $_REQUEST[‘last_name’];
$gender = $_REQUEST[‘gender’];
$address = $_REQUEST[‘address’];
$email = $_REQUEST[’email’];
// We are going to insert the data into our table, sampletable
$sql = “INSERT INTO sampletable VALUES (‘$first_name’,
‘$last_name’,’$gender’,’$address’,’$email’)”;
// Check if the query is successful
if(mysqli_query($conn, $sql)){
echo “<h3>data stored in a database successfully.”
. ” Please browse localhost\phpmyadmin”
. ” to view the updated data</h3>”;
echo nl2br(“\n$first_name\n $last_name\n “
. “$gender\n $address\n $email”);
} else{
echo “ERROR: Hush! Sorry $sql. “
. mysqli_error($conn);
}
// Close connection
mysqli_close($conn);
?>
</center>
</body>
</html>
Now we can submit form data to the database but first we need to save index.php
and insert.php
files inside of htdocs
folder located inside Xampp directory.
Now we can head over to localhost/index.php
to fill out the form submit it to our database.
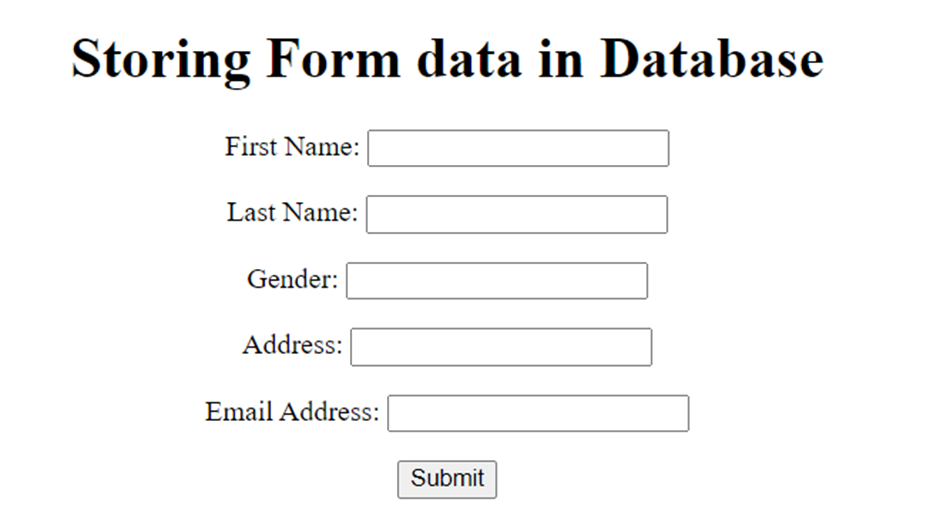
Fill out the form and then click submit
.
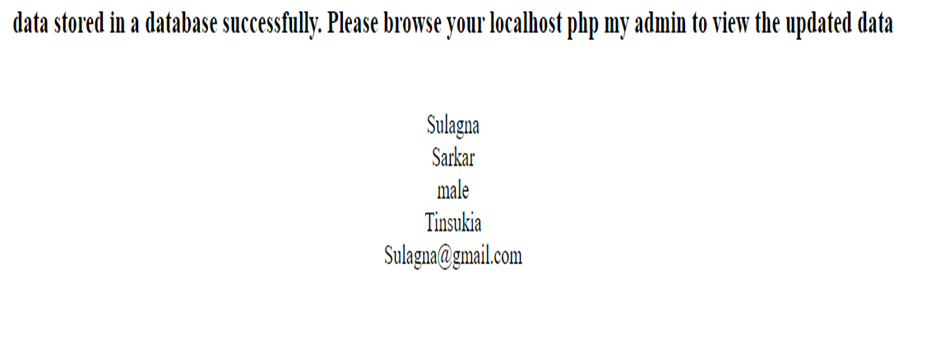
Go to localhost/phpmyadmin
 and select the database to ensure that data was successfully inserted.
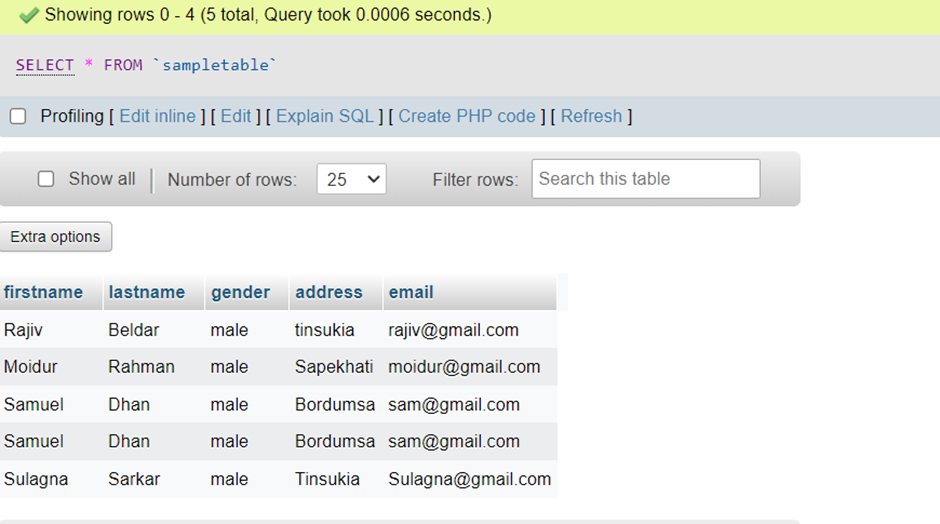
In the awesome design of things you actually get an A+ for effort. Where you actually confused us ended up being on all the specifics. You know, as the maxim goes, details make or break the argument.. And it could not be more true in this article. Having said that, allow me inform you what exactly did give good results. The writing is definitely rather powerful and that is possibly why I am making the effort in order to comment. I do not really make it a regular habit of doing that. Secondly, even though I can certainly notice a jumps in reason you make, I am definitely not certain of just how you seem to connect the details which produce the actual conclusion. For the moment I will yield to your point however hope in the near future you actually connect the dots much better.