1. Write a C++ program to check two numbers are equal or not.
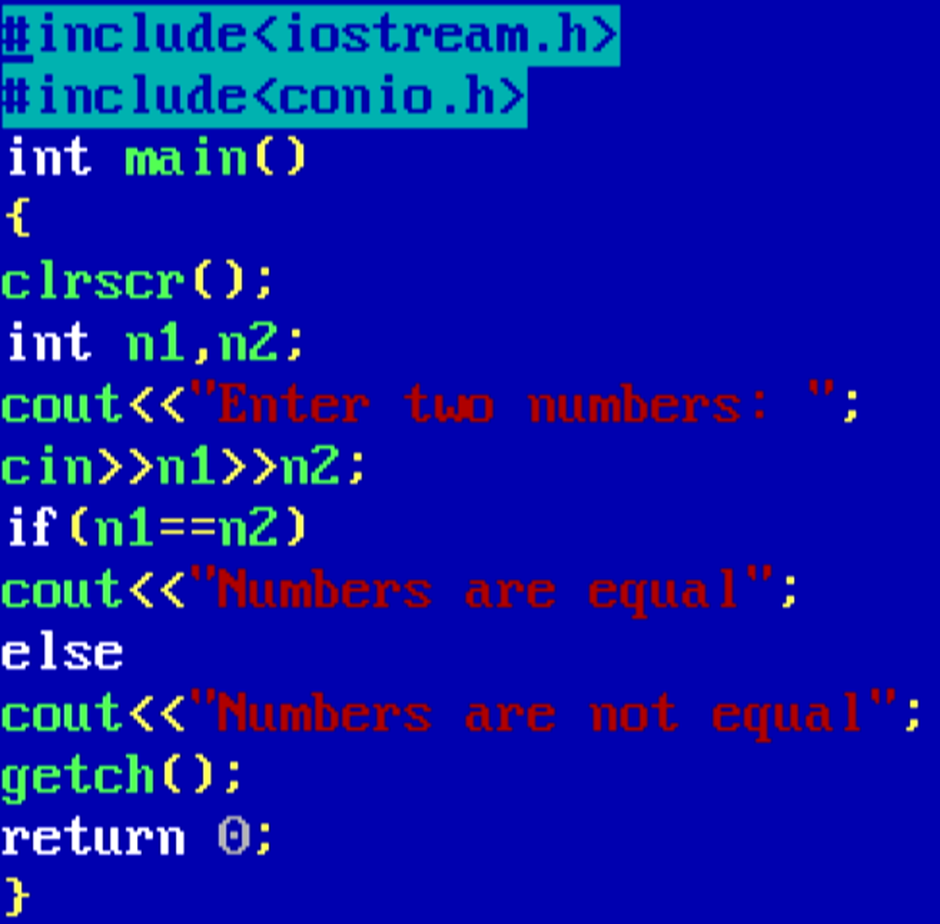
Output:

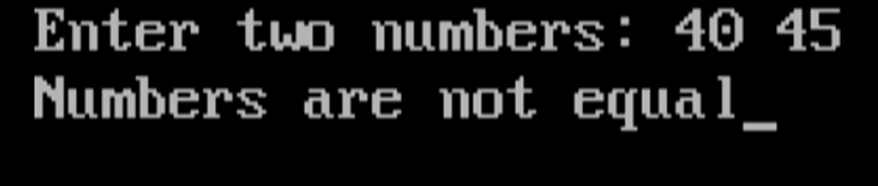
2. Write a program to find the greater number among two numbers.
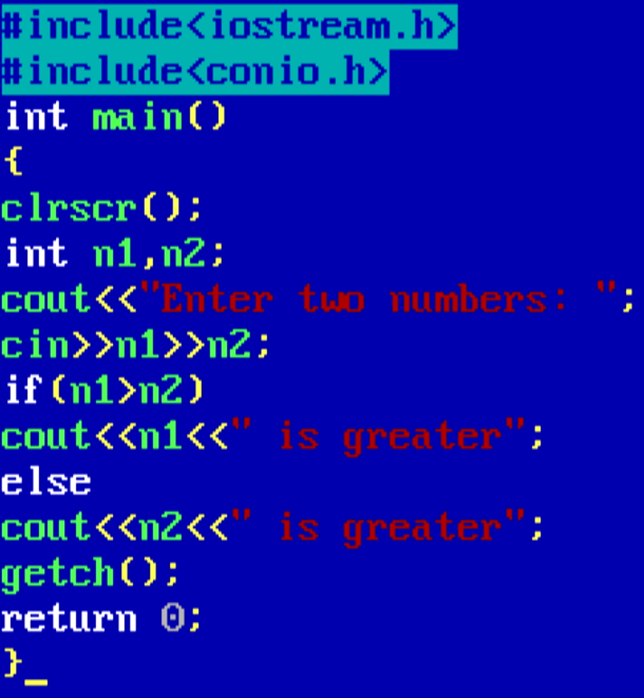
Output:

3. Write a C program to find maximum between two numbers.
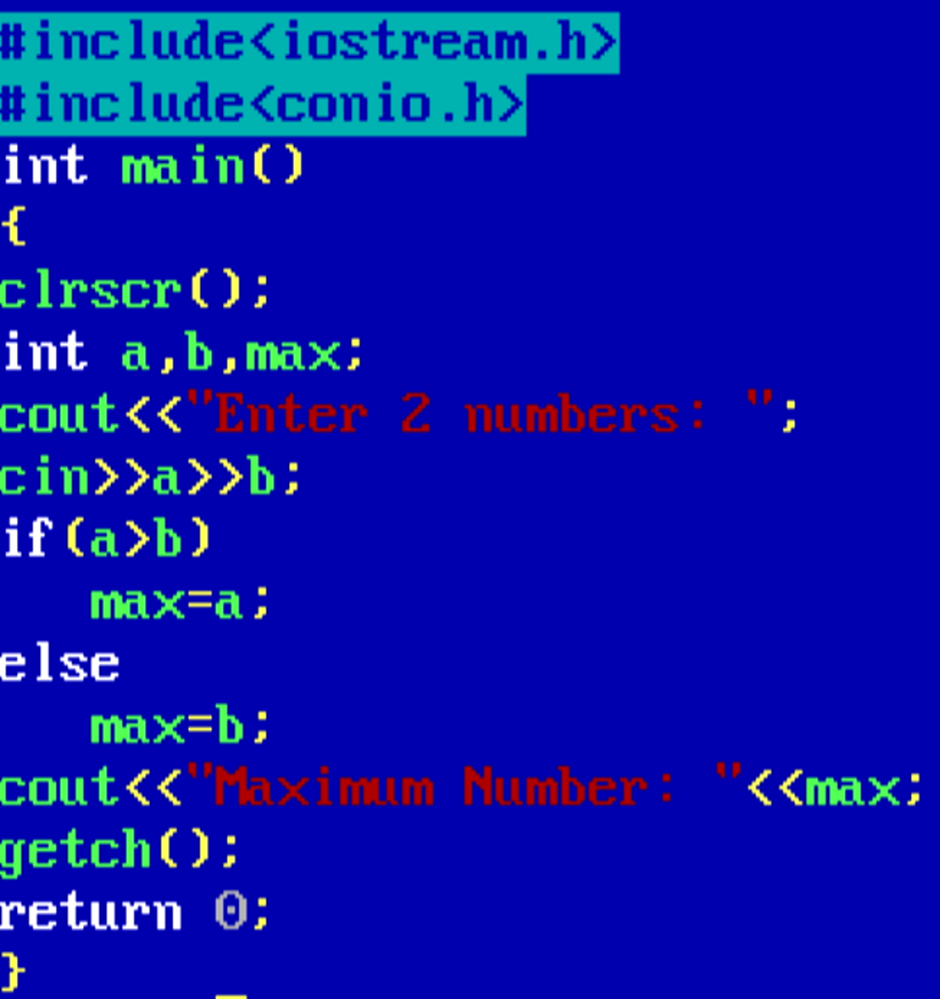
Output:
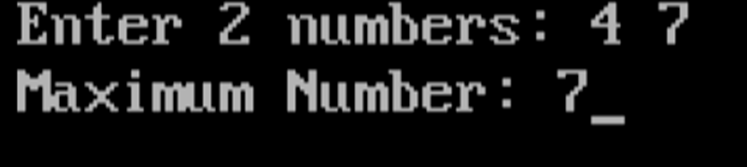
4. Write a C program to find maximum between two numbers using conditional operator.
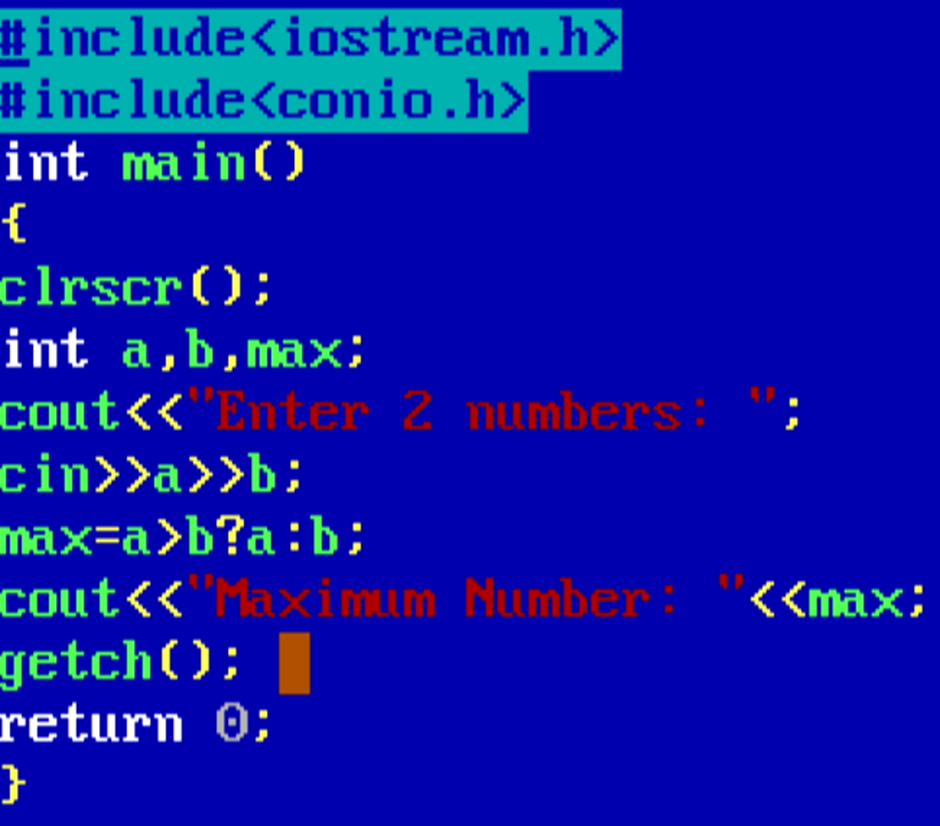
Output:
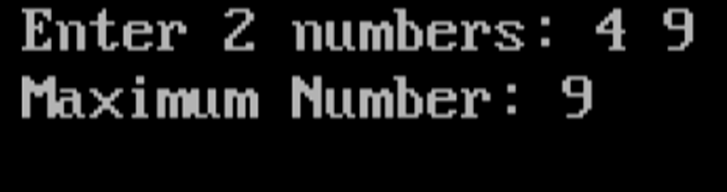
5. Write a program to check whether a number is even or odd.
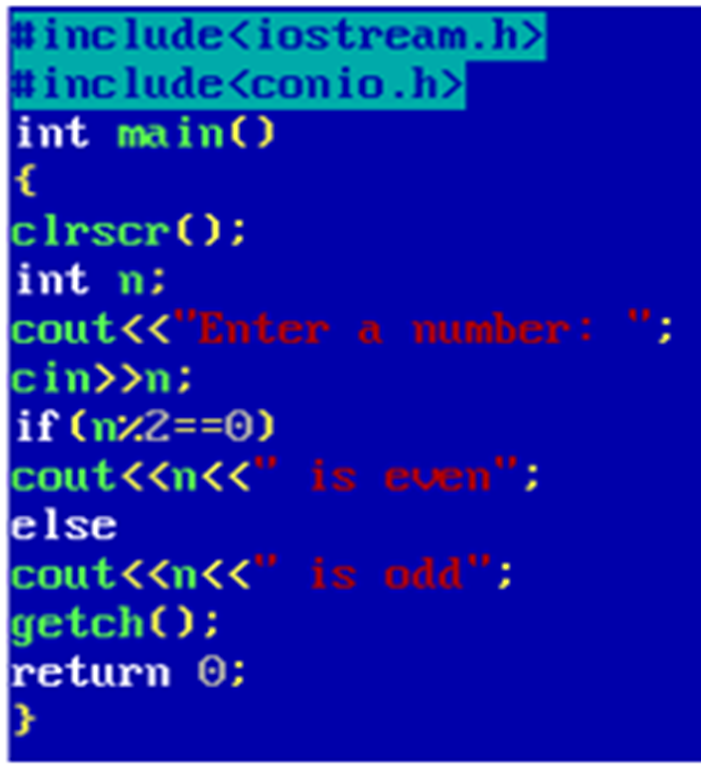
Output:
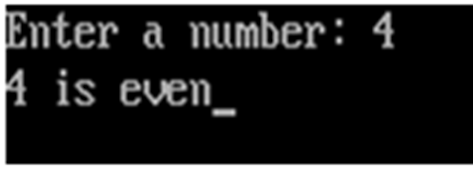
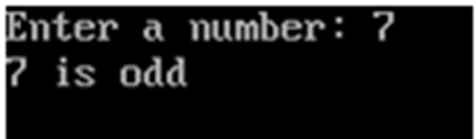
6. Write a program to check whether a year is leap year or not.
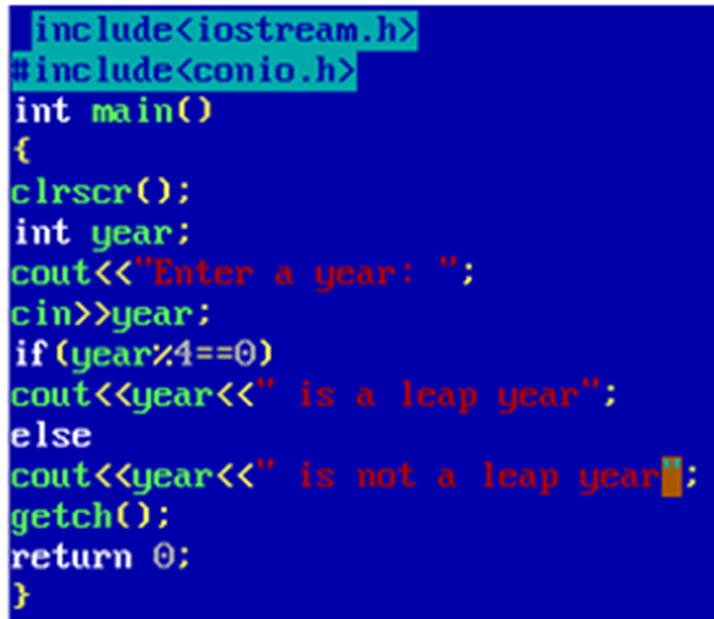
Output:
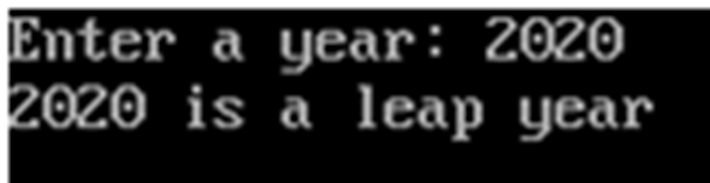
7. Write a C++ program to find the largest number among three numbers.

Output:
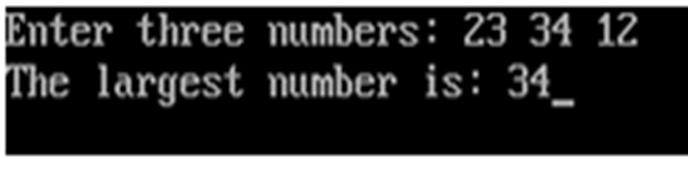
8. Write a C++ program to find the maximum of three numbers using conditional operators.

Output:

9. Write a C++ program to check whether a character is vowel or not using switch case.
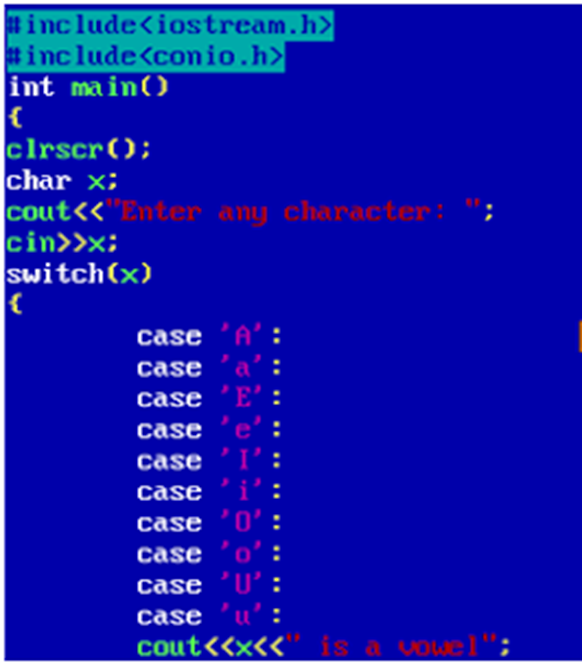
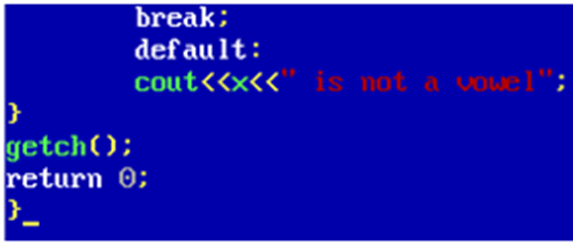
Output:
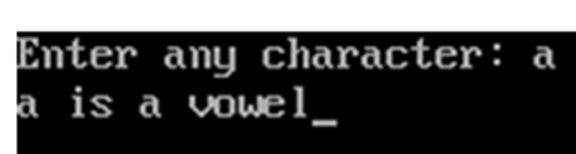
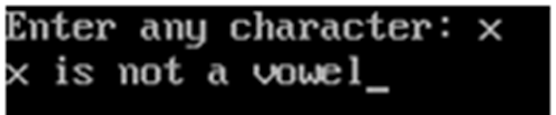
10. Write a program in C++ to display the name of the day in a week.
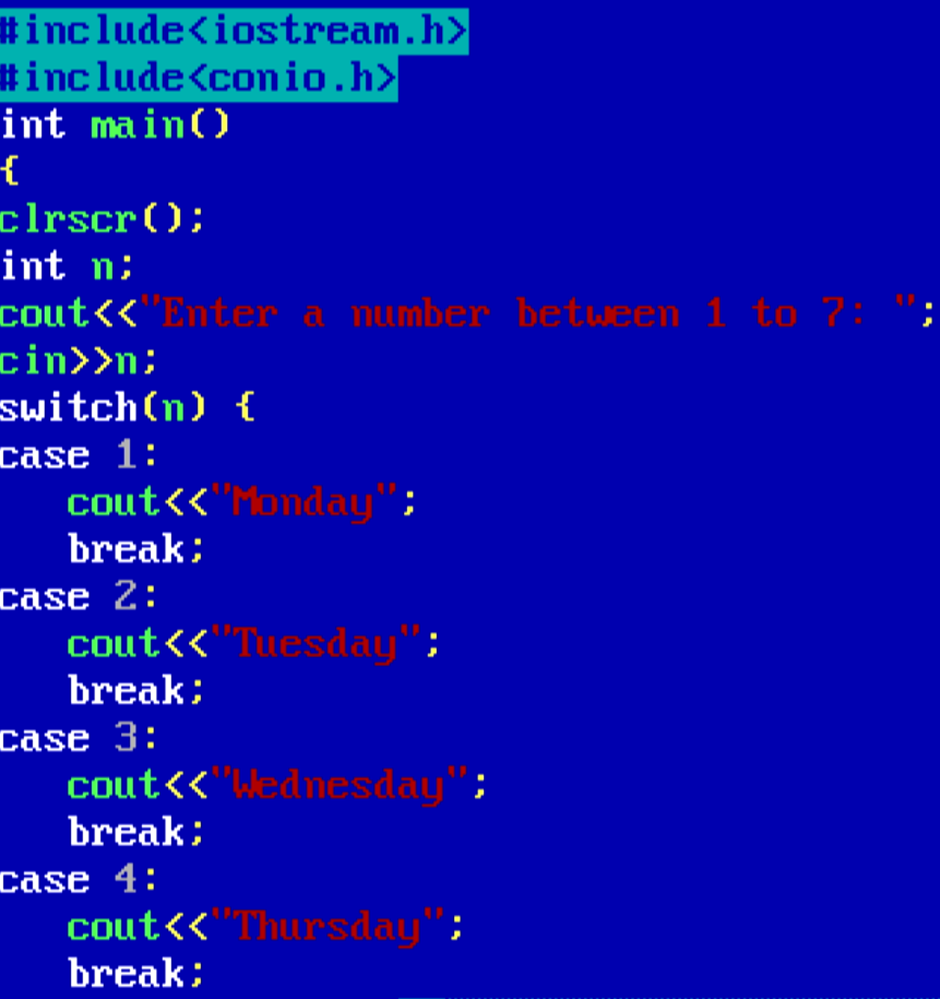
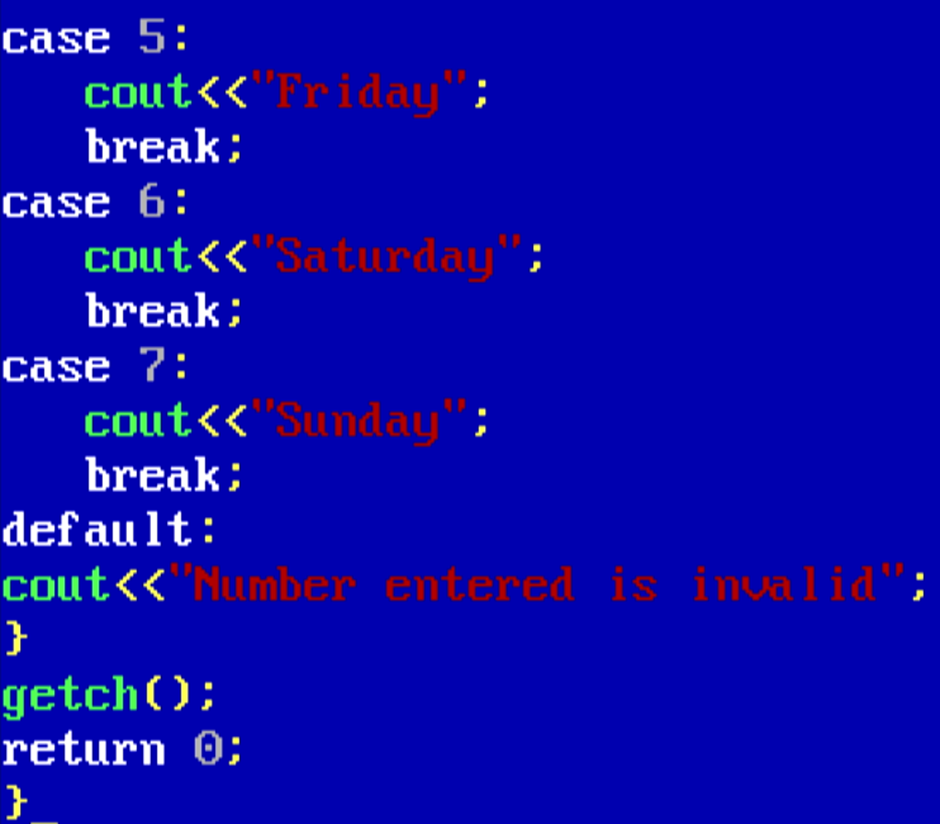
Output:


11. Write a C++ program to make a simple calculator to add, subtract, multiply and divide using switch case.
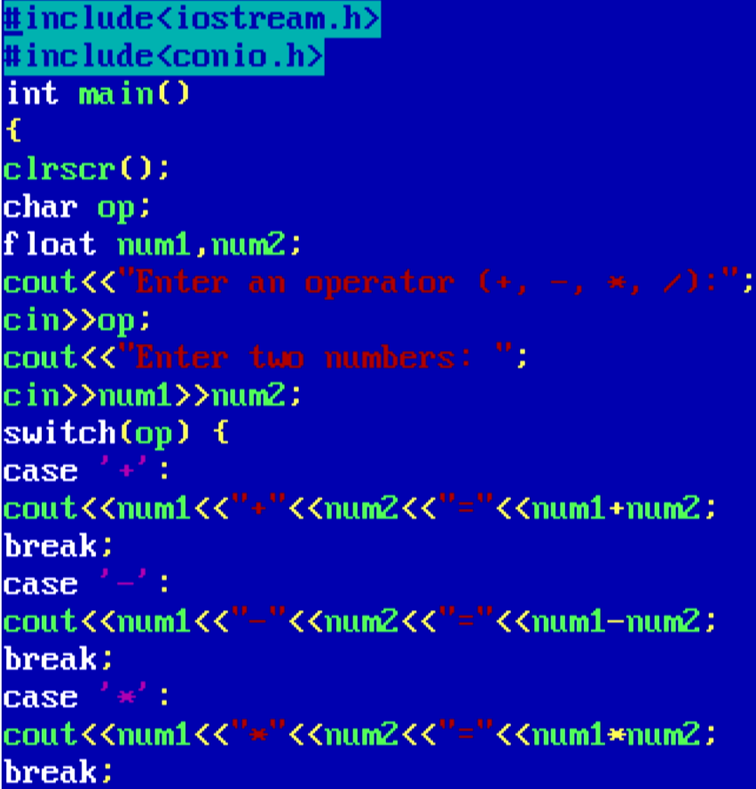
case ‘/’:
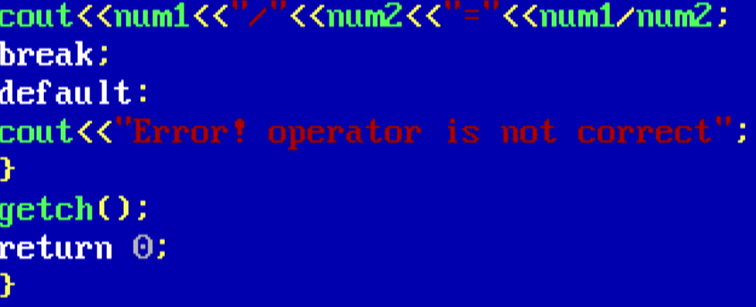
Output:
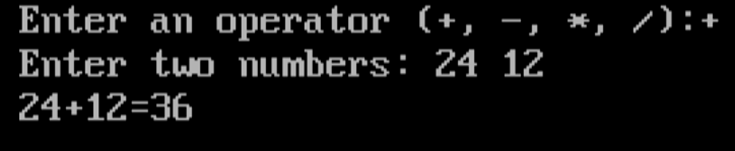
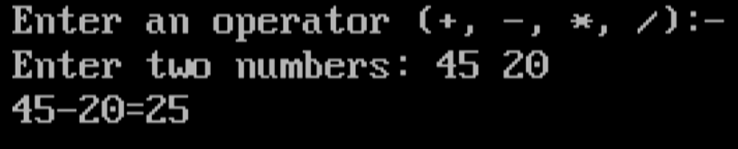


12. Write a C++ program to print 1st 100 natural numbers.
OR
Write a program in C++ to print the number from 1 to 100.
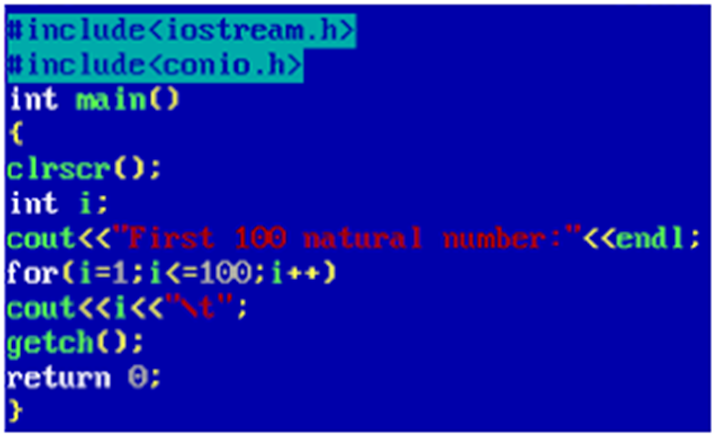
Output:
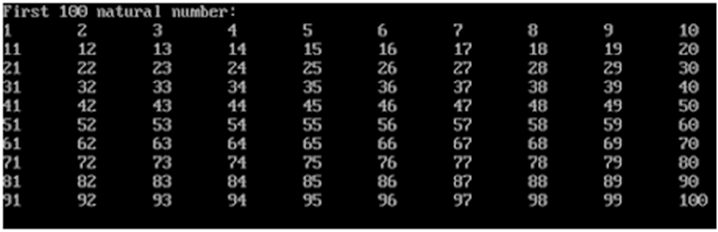
13. Write a C++ program to generate Multiplication Table.
OR
Write a C++ program to find the multiplication table of a number using for loop.
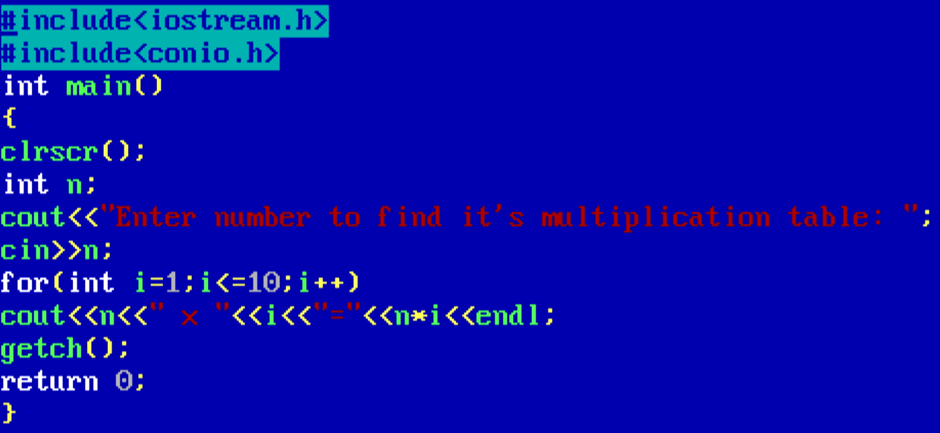
Output:
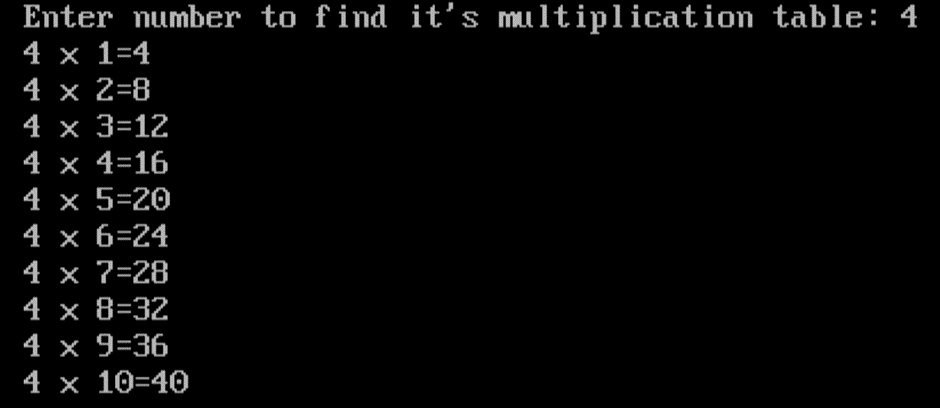
14. Write a C++ program to print multiplication table from 1 to 5.
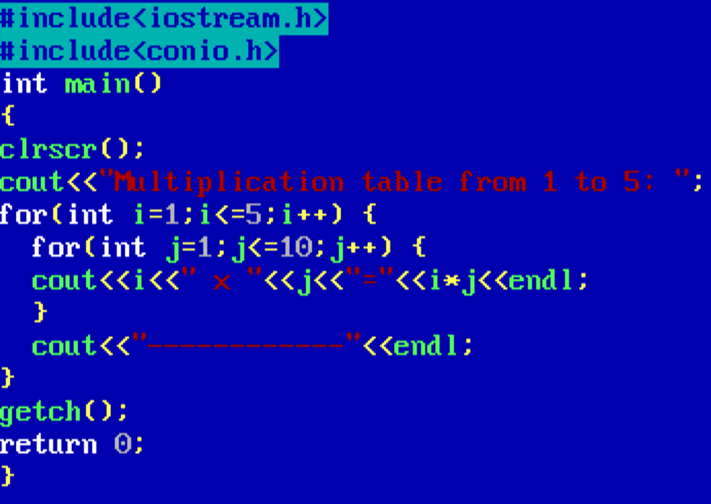
Output:
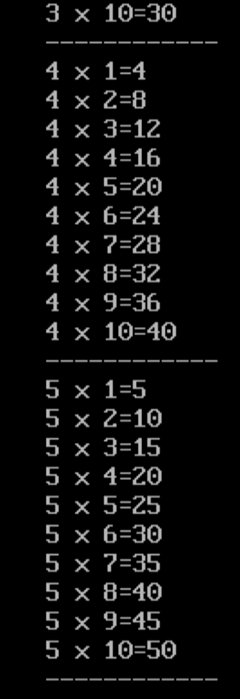
15. Write a program to calculate sum of first n natural numbers.
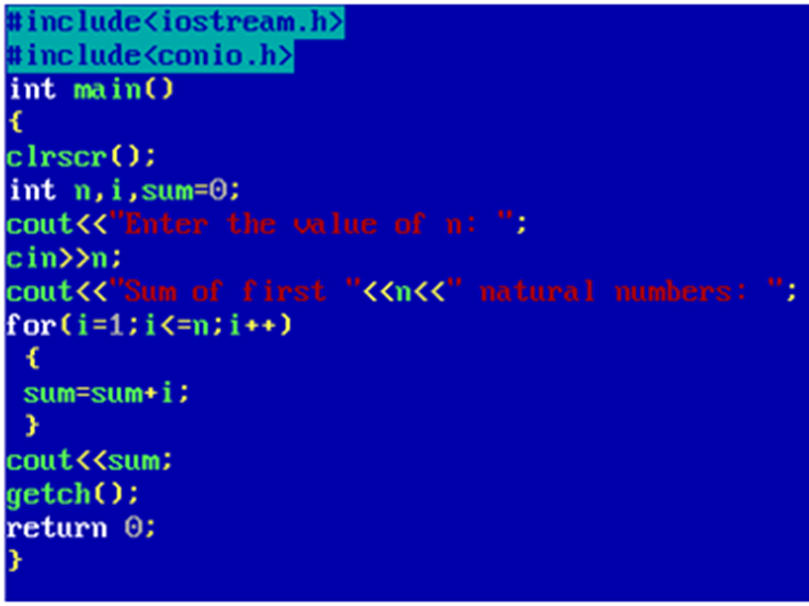
Output:

16. Write a program in C++ to print the following series
1+1/2+1/3+1/4…….+1/n
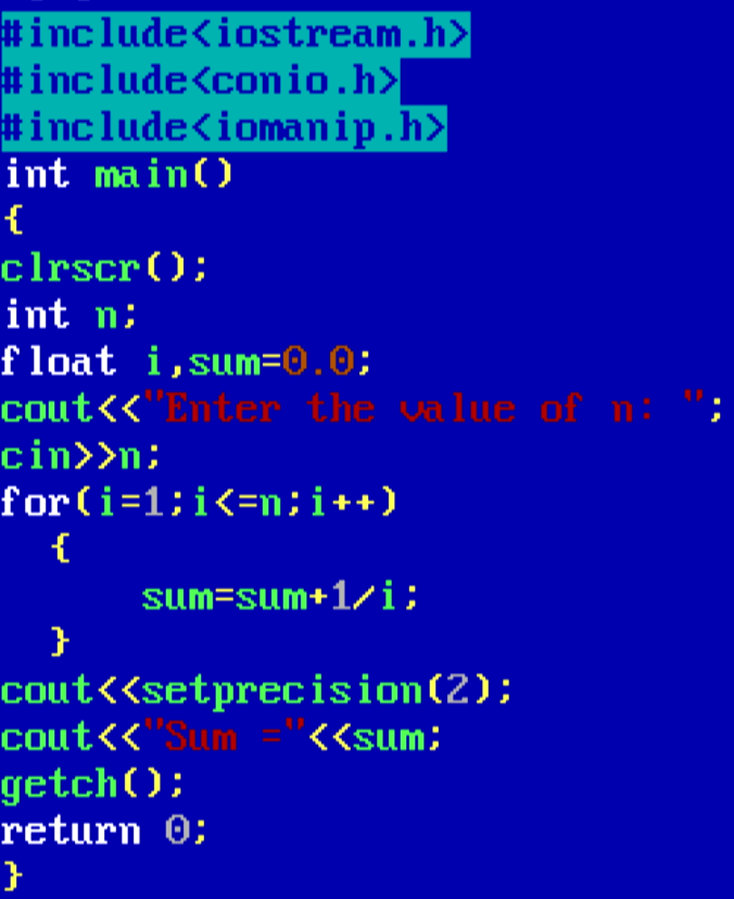
Output:

17. Write a C++ program to print the following pattern using For Loop.
*
* *
* * *
* * * *
* * * * *
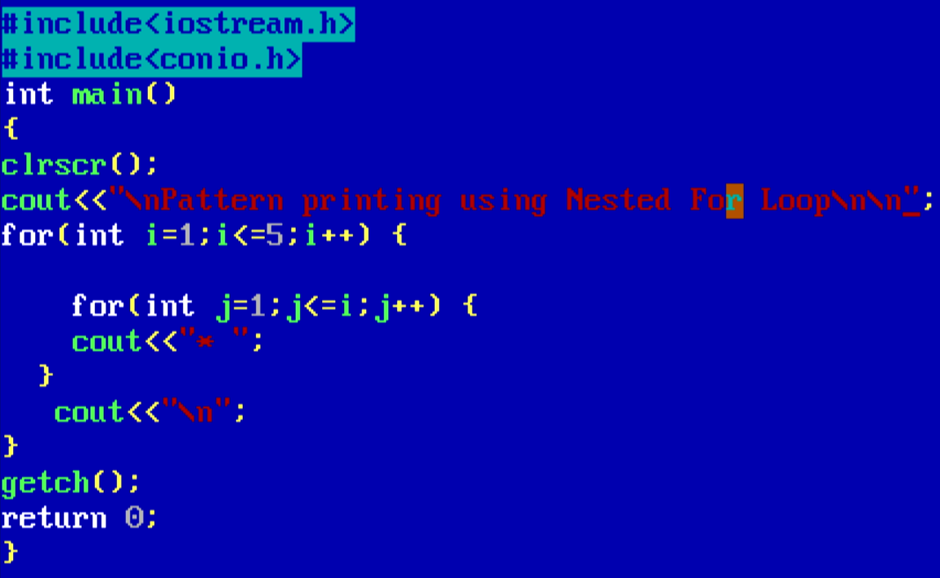
Output:
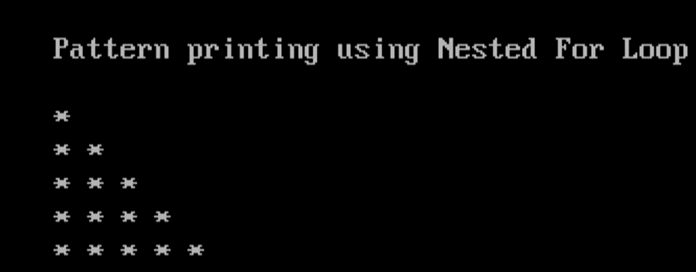
18. Write a program in C++ to print the following pattern using while loop.
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
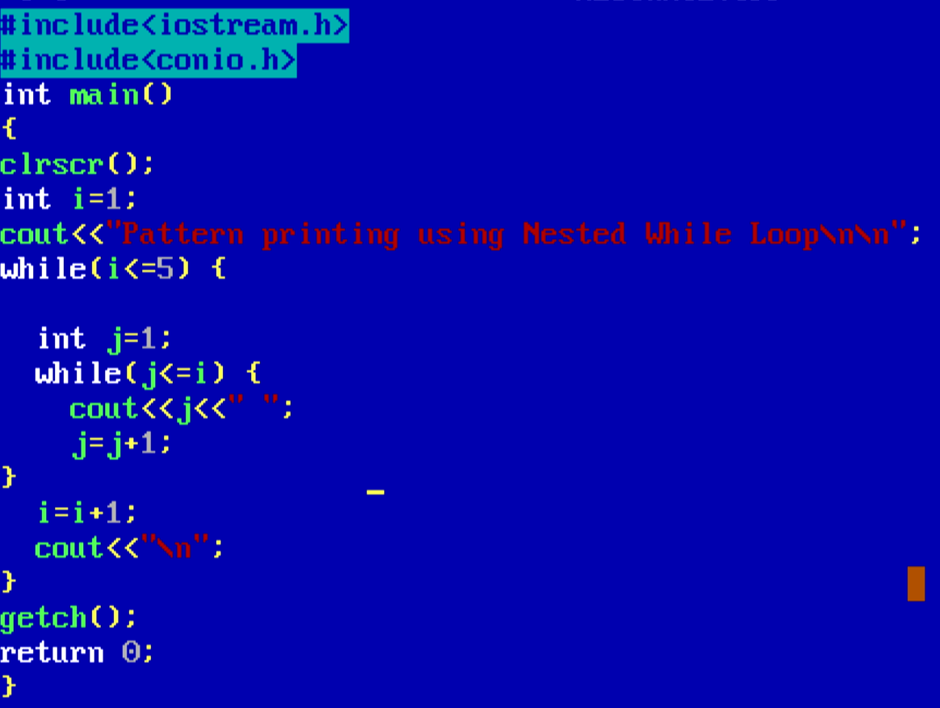
Output:
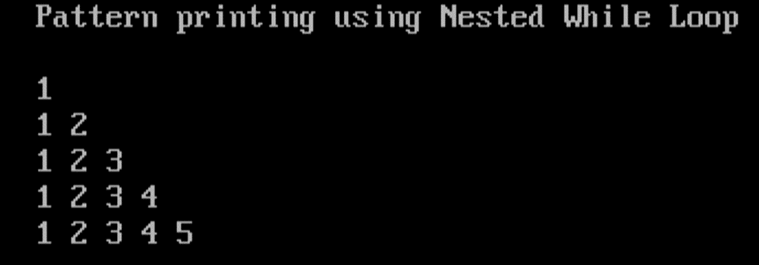
19. Write a C++ program to display Fibonacci Series.
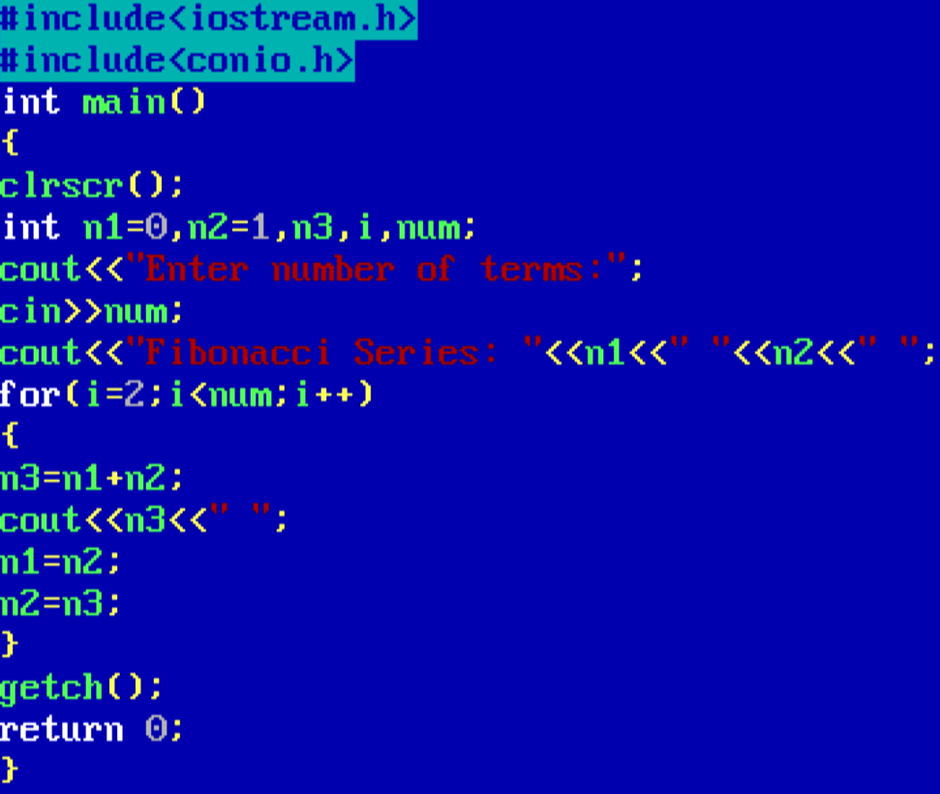
Output:

20. Write a C++ program to print the reverse of a given number.
OR
Write a program in C++ to reverse digits of an integer.
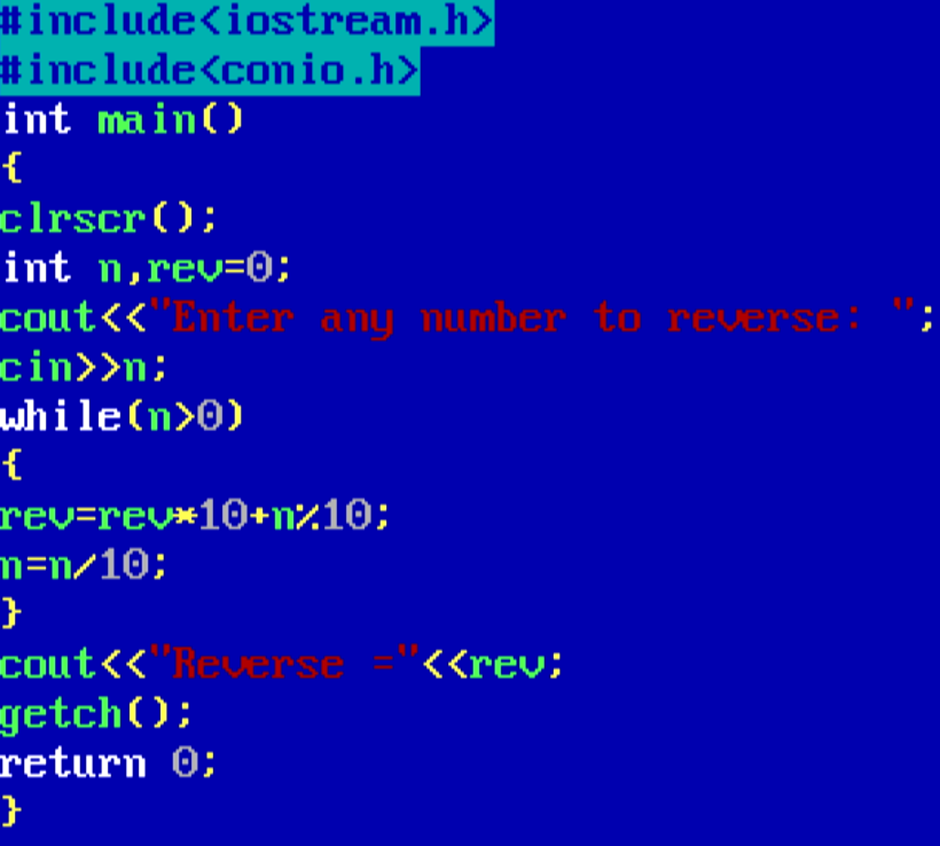
Output:

21. Write a C program to calculate the sum of a 3 digit number.
OR
Write a C program to find the sum of a given integer.
OR
Write a C program to find the sum of digits in a given number.
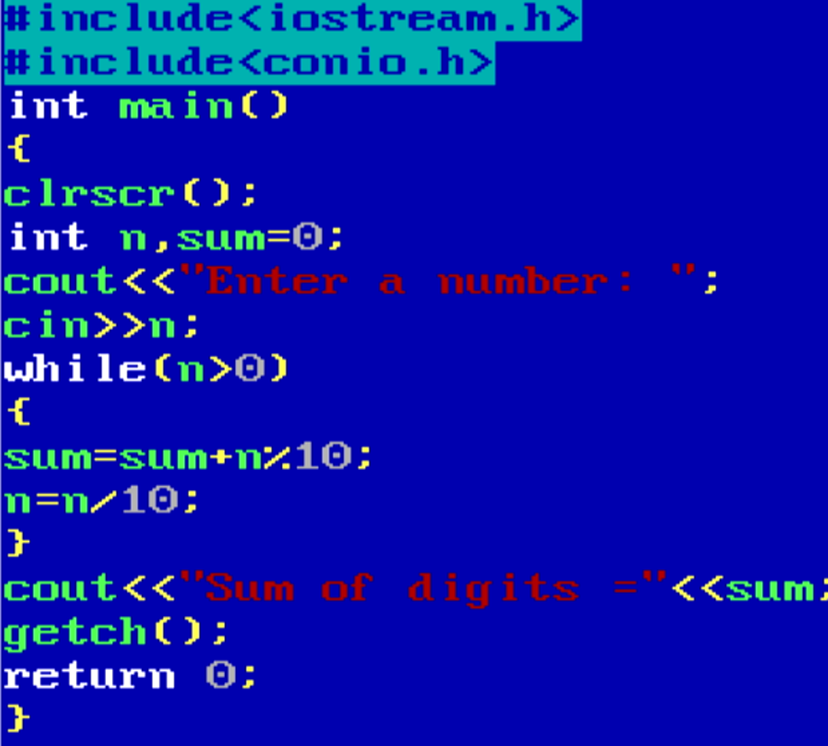
Output:
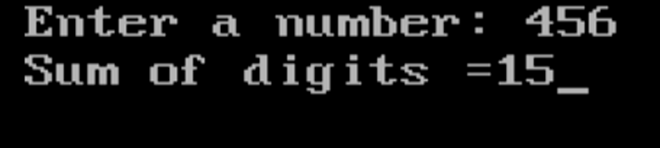
22. Write a program to print elements of a one dimensional (1D) array.
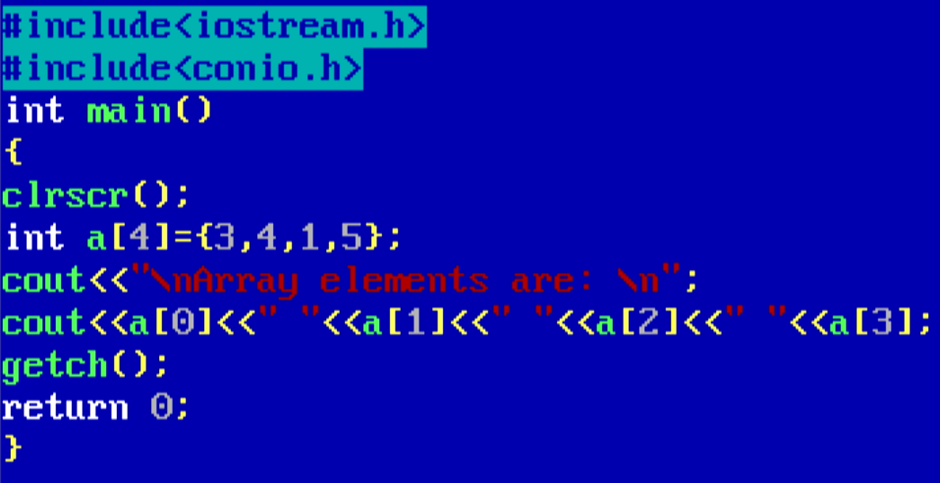
Output:
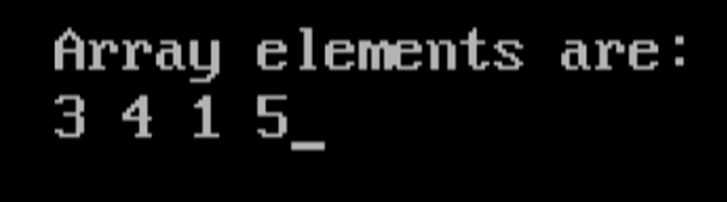
OR
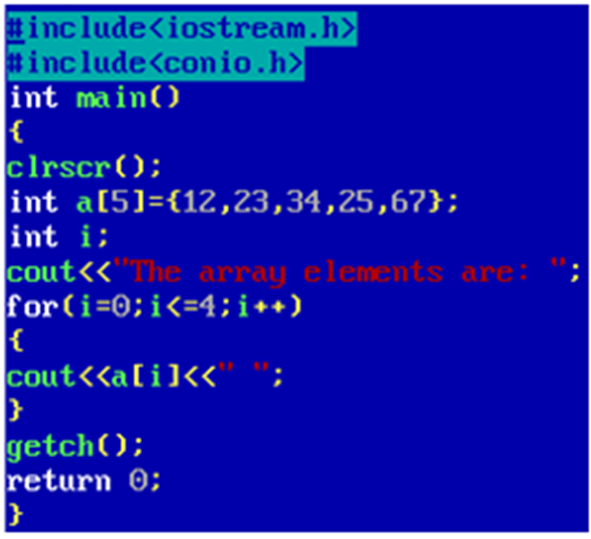
Output:

23. Write a program to print elements of a one dimensional (1D) array taking input from user.
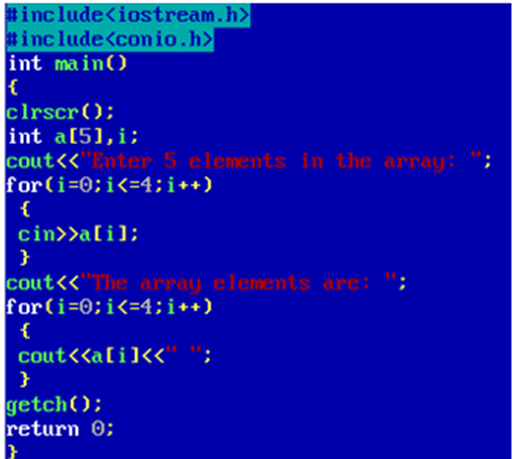
Output:

24. Write a program to print sum and average of array elements.
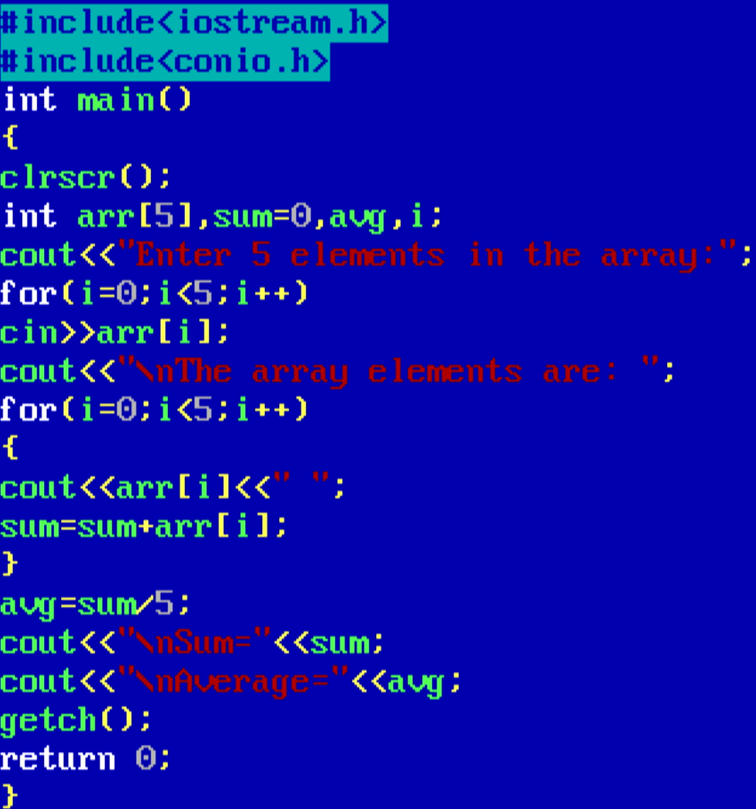
Output:
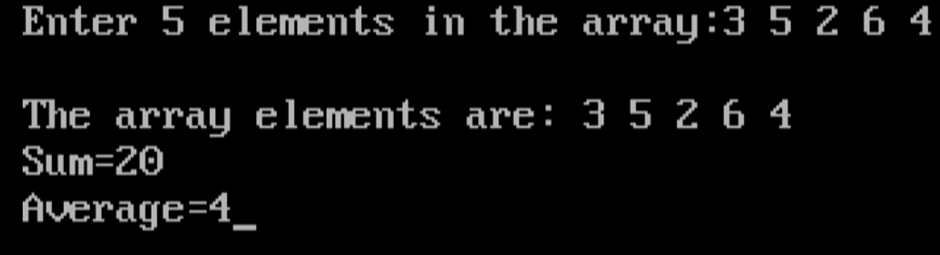
25. Write a program to calculate total and average marks of 5 subjects.
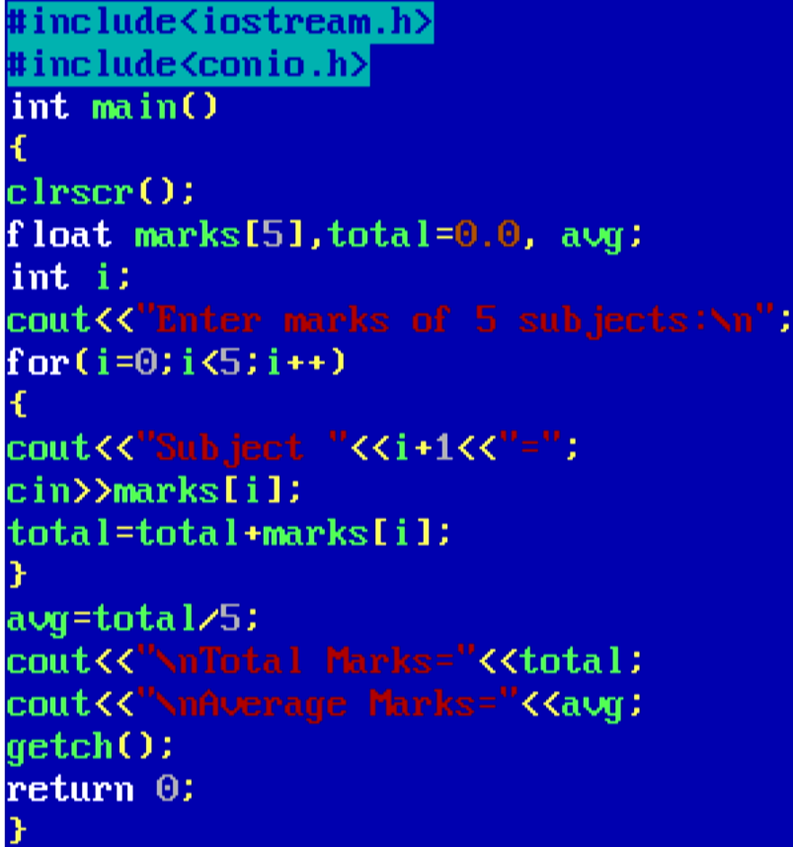
Output:
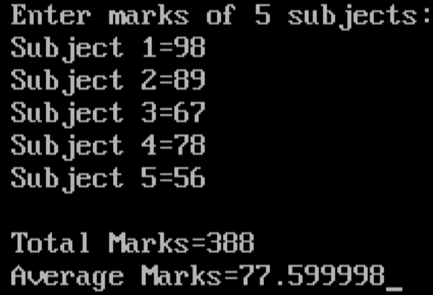
26. Write a program to find the biggest number in an array.
OR
Write a program in C++ to find the largest element of an array.
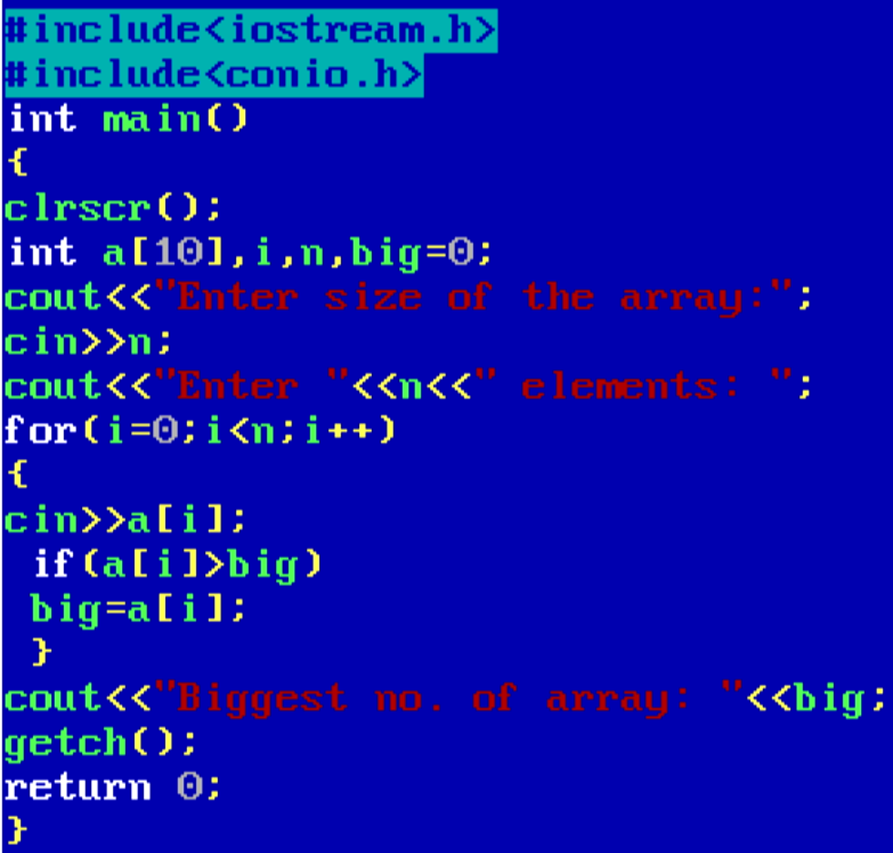
Output:
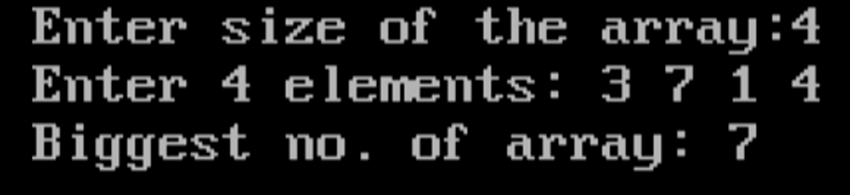
OR
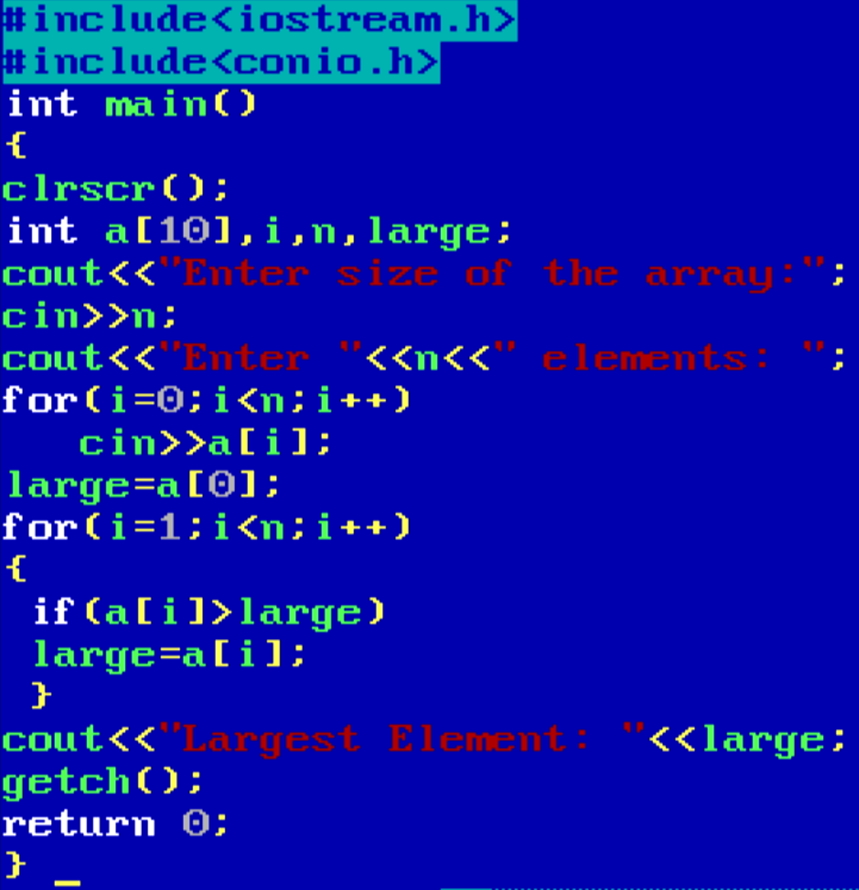
Output:
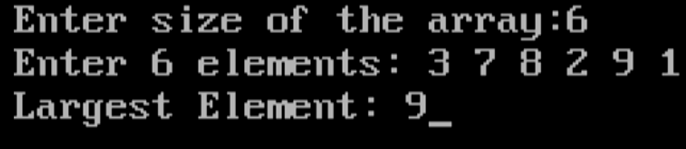
27. Write a program in C to display the largest and smallest element in an integer array.
Or
Write a C program to find maximum and minimum element in array.
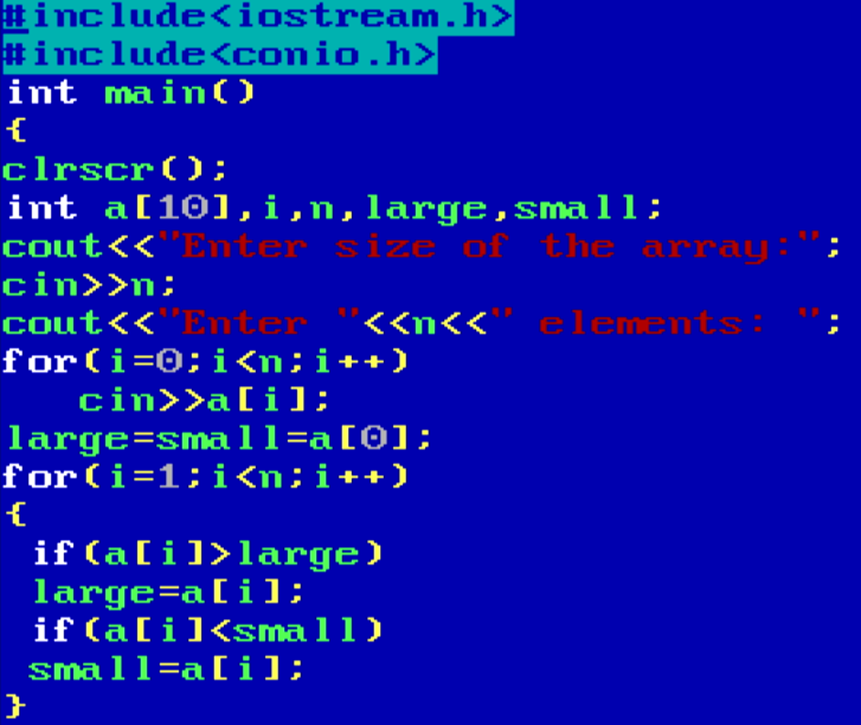
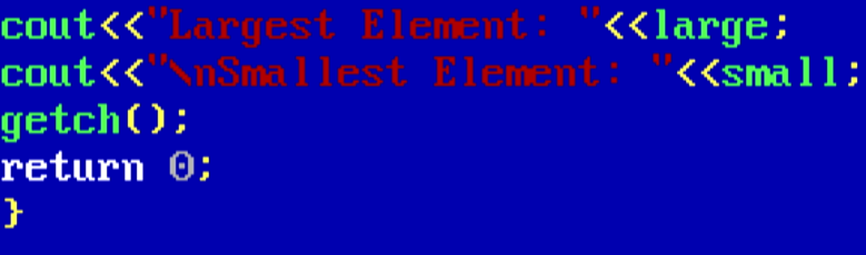
Output:
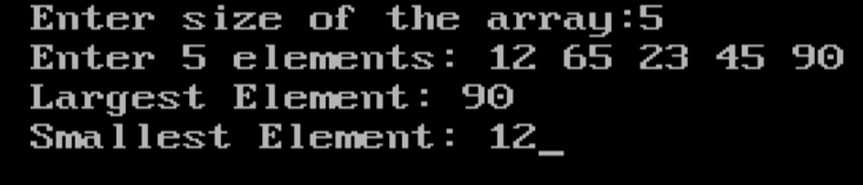
28. Write a program to print elements of a two-dimensional (2D) array.
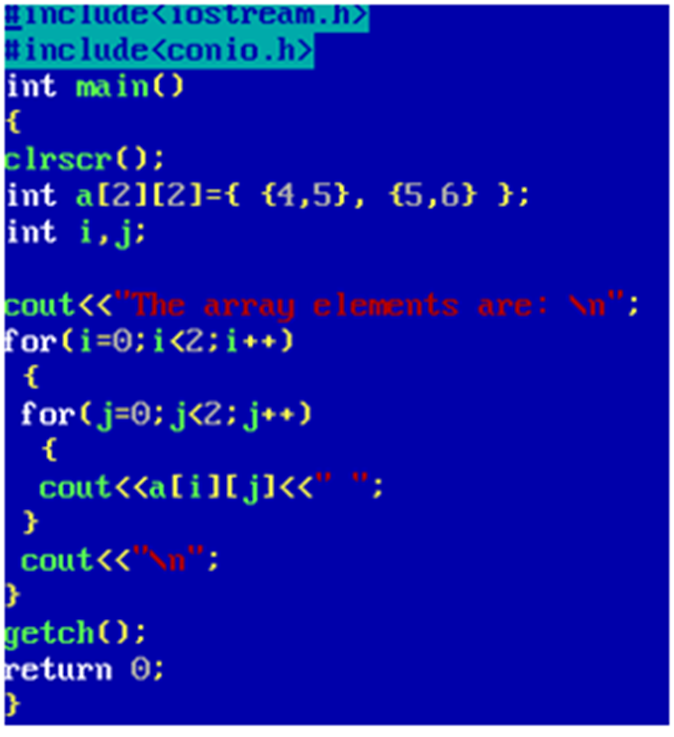
Output:

29. Write a program to read and print elements of a two dimensional (2D) array.
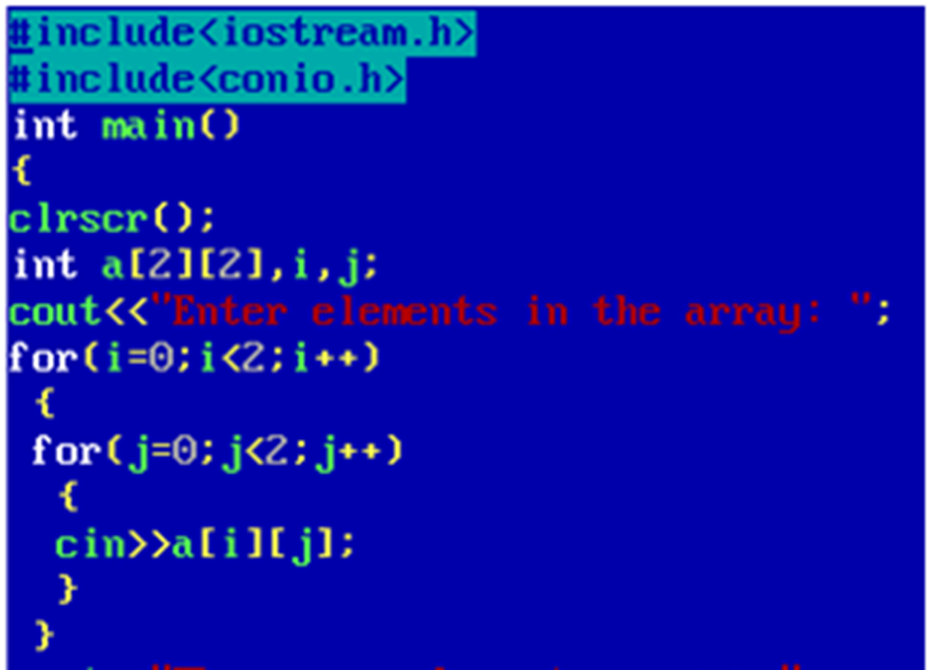
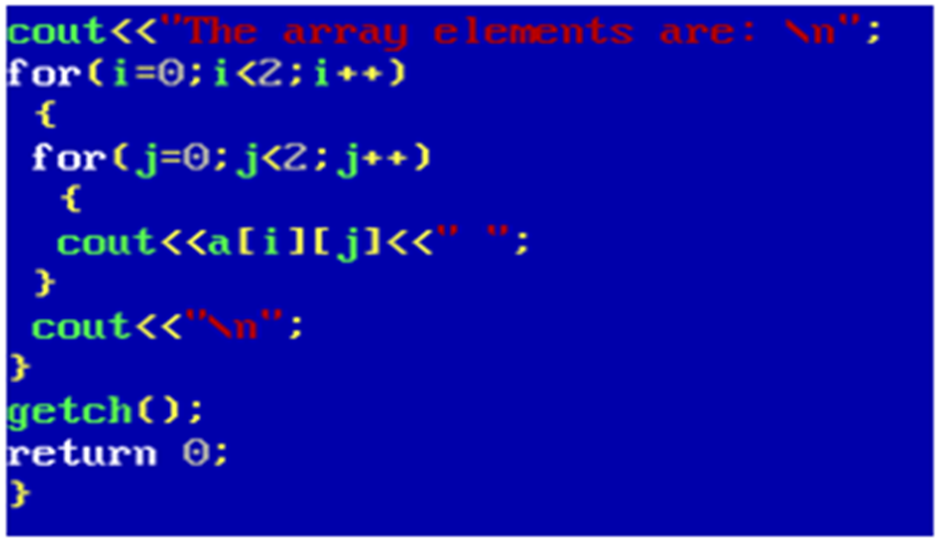
Output:
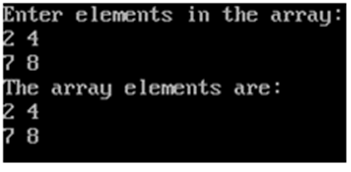
30. Write a C++ program to find maximum and minimum element in a two-dimensional (2D) array.
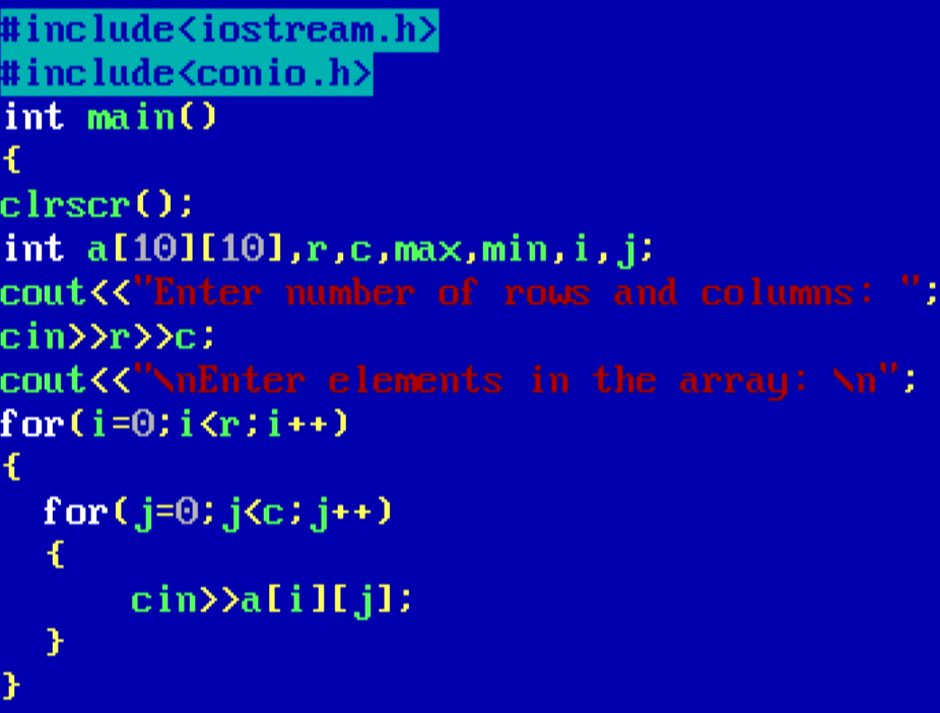
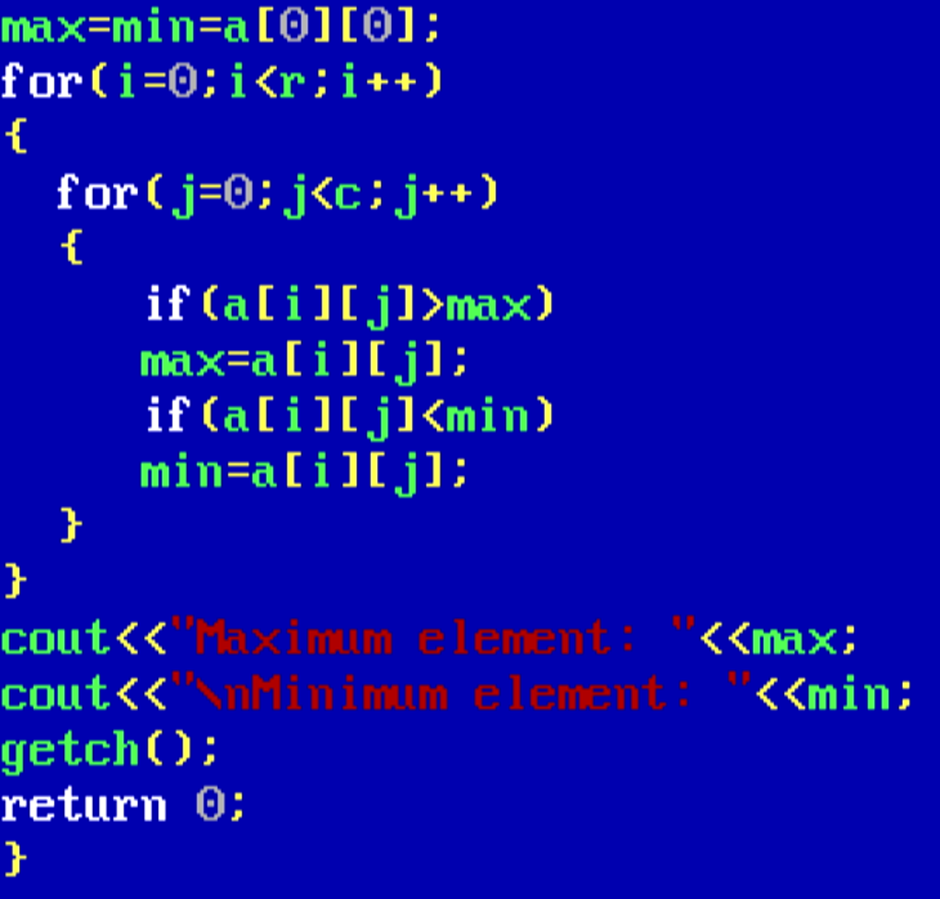
Output:
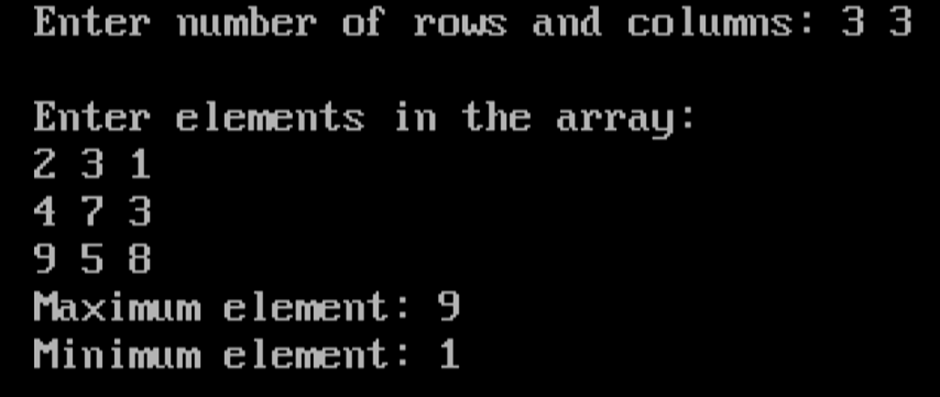
31. Write a C++ program to add two matrices.
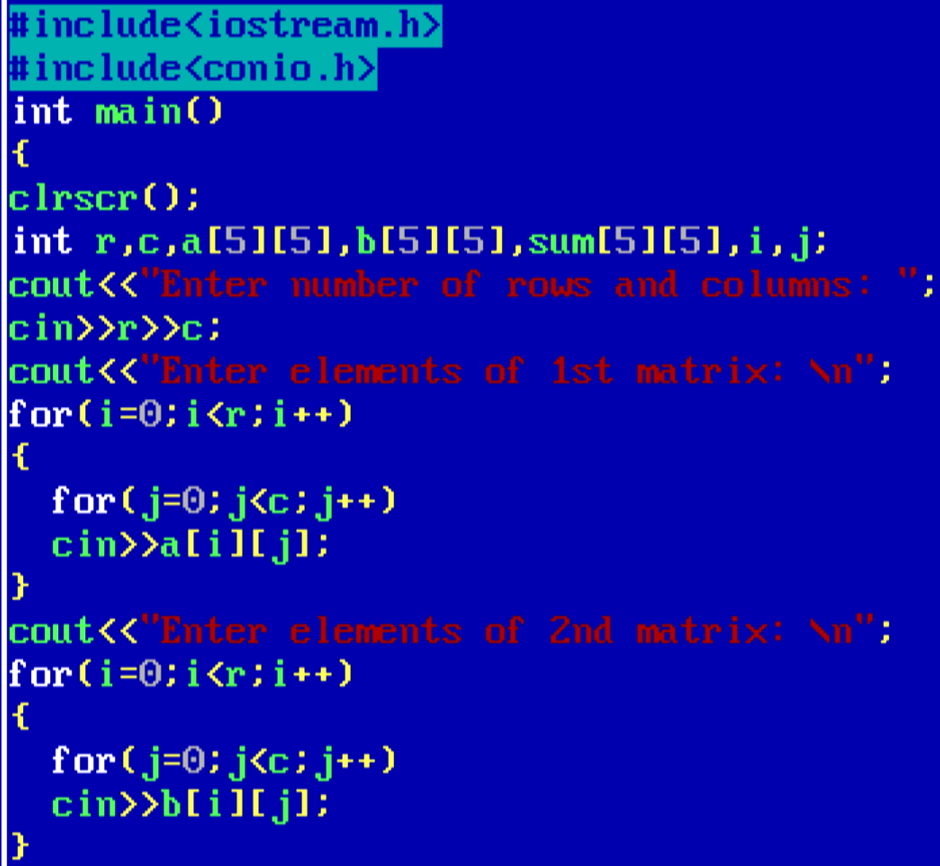
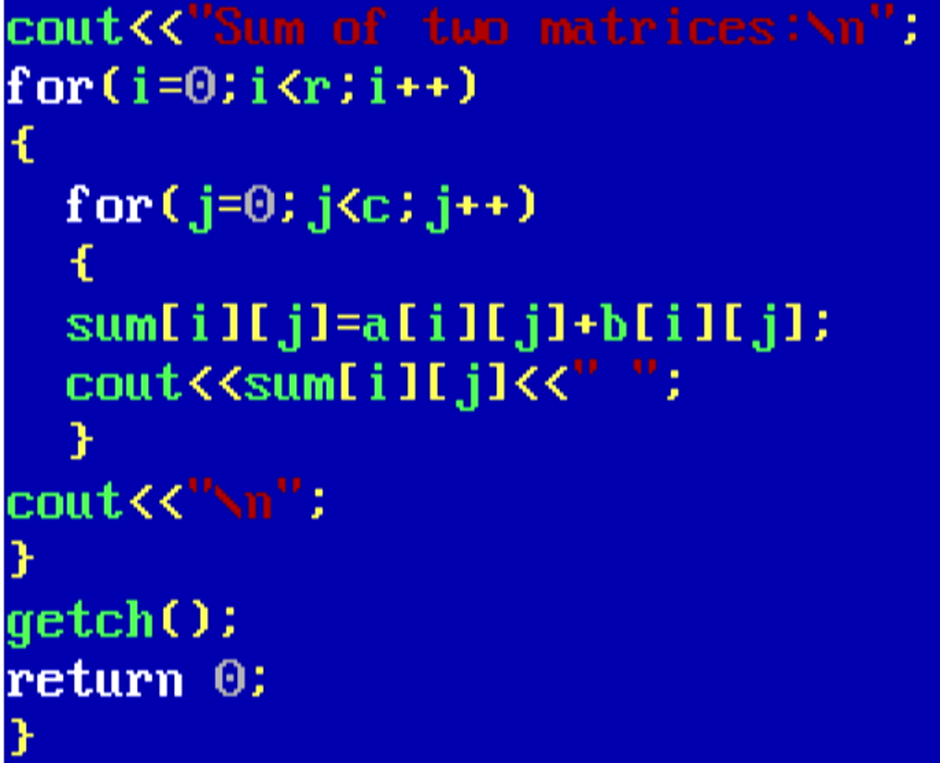
Output:
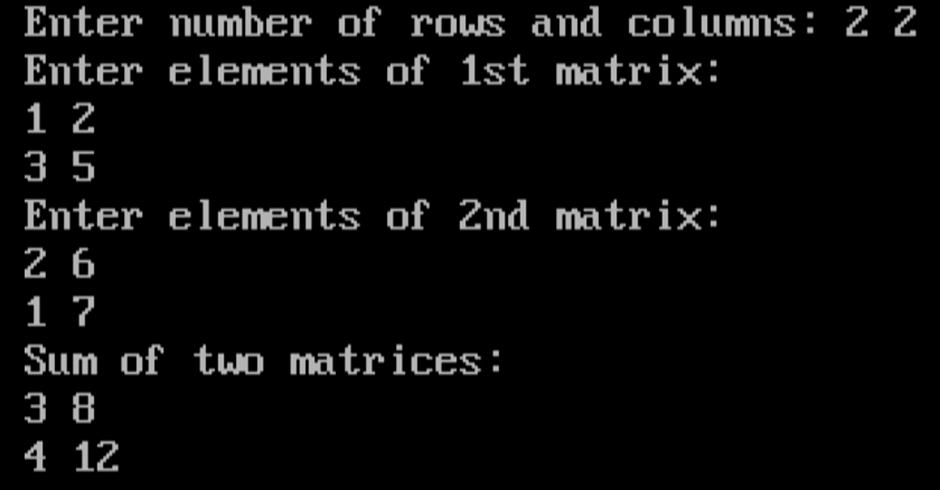
32. Write a C++ program to find the difference between two matrices.
OR
Write a C++ program to subtract two matrices.
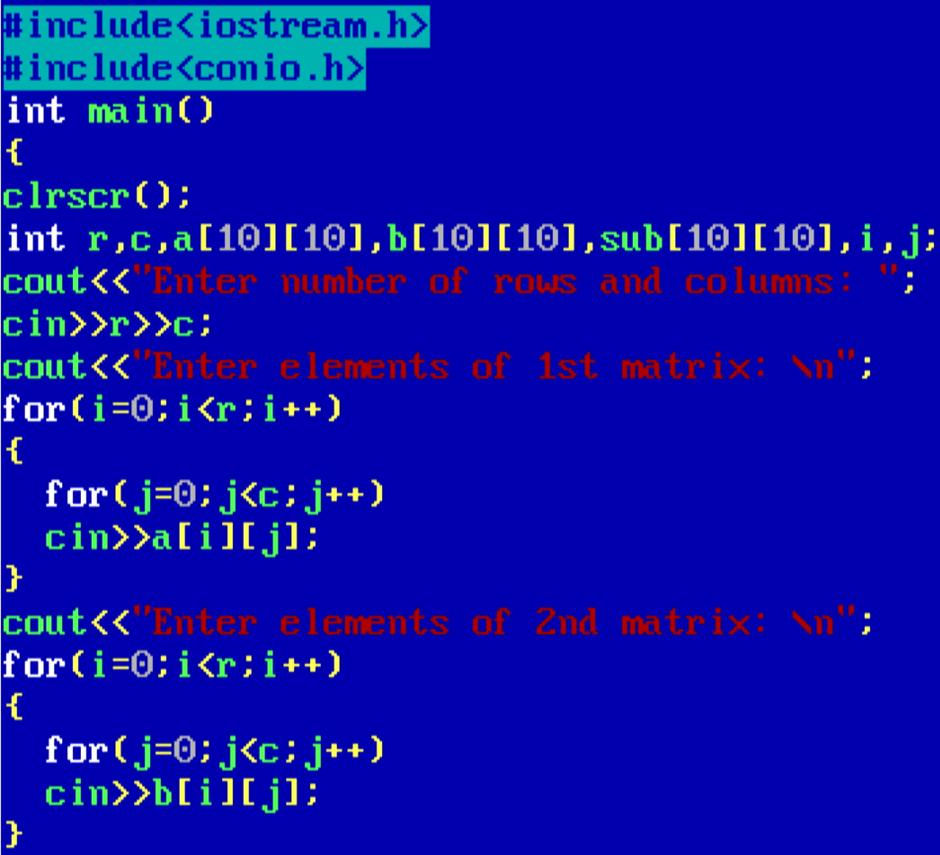
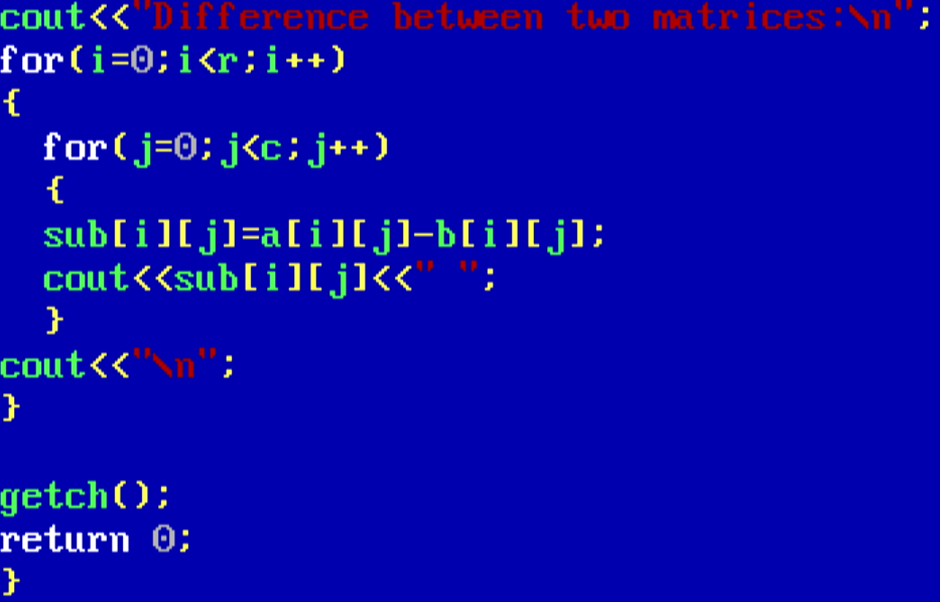
Output:
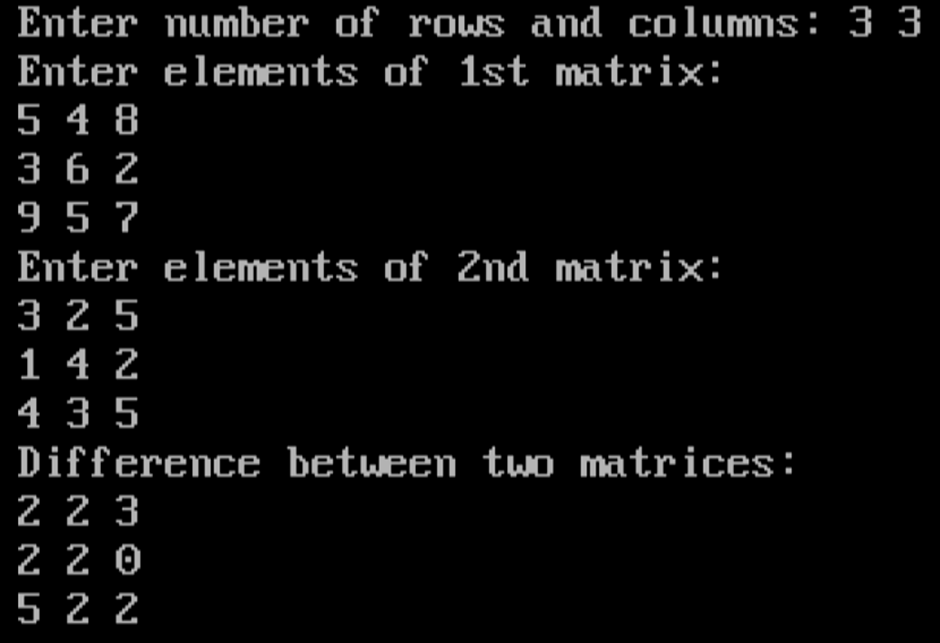
33. Write a C++ program to multiply two matrices.
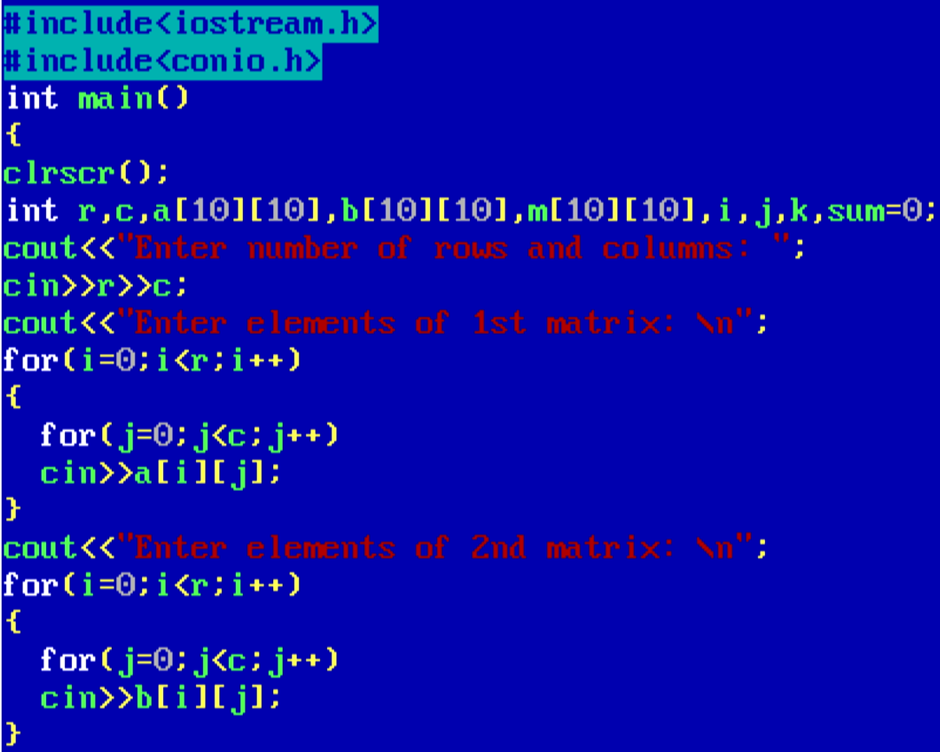
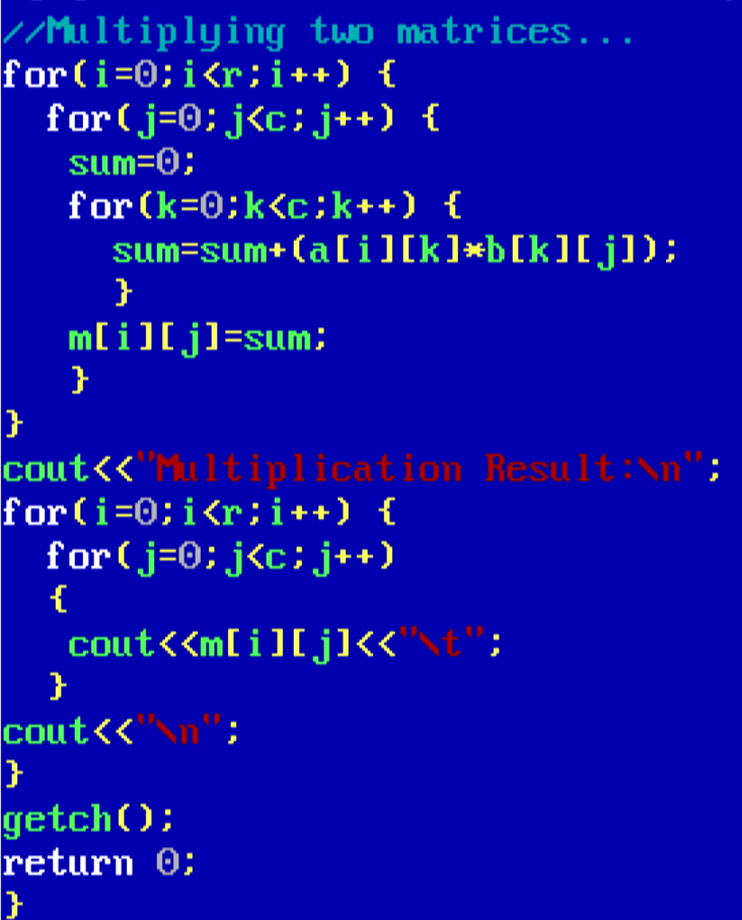
Output:
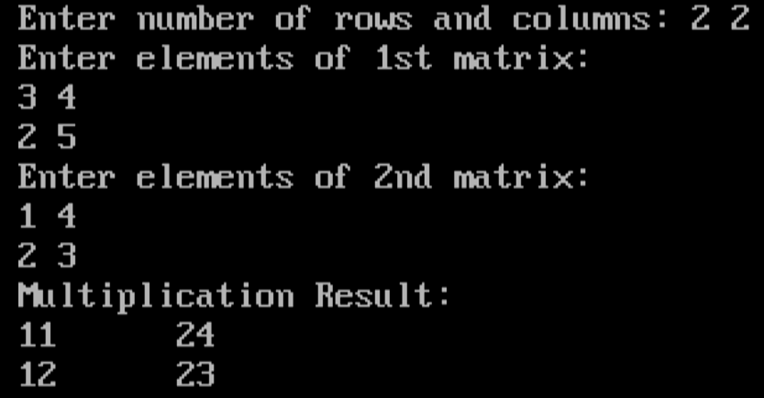
34. Write a C++ program to store information of Students using structure.
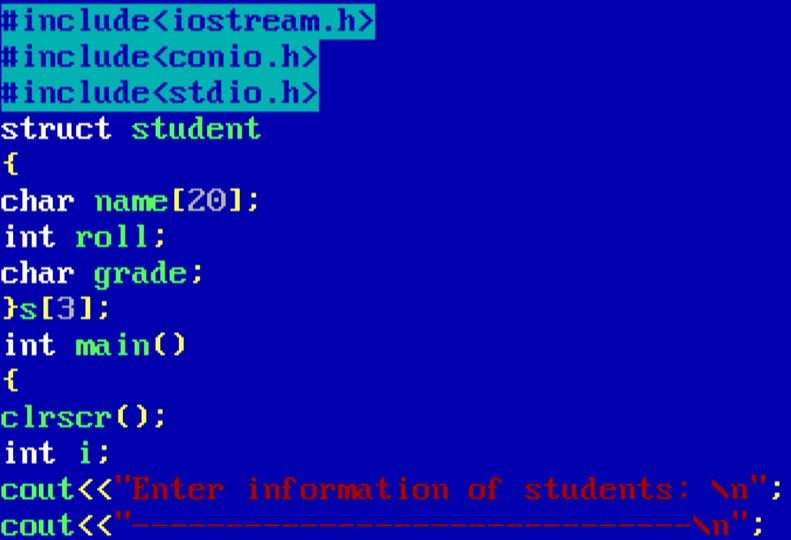
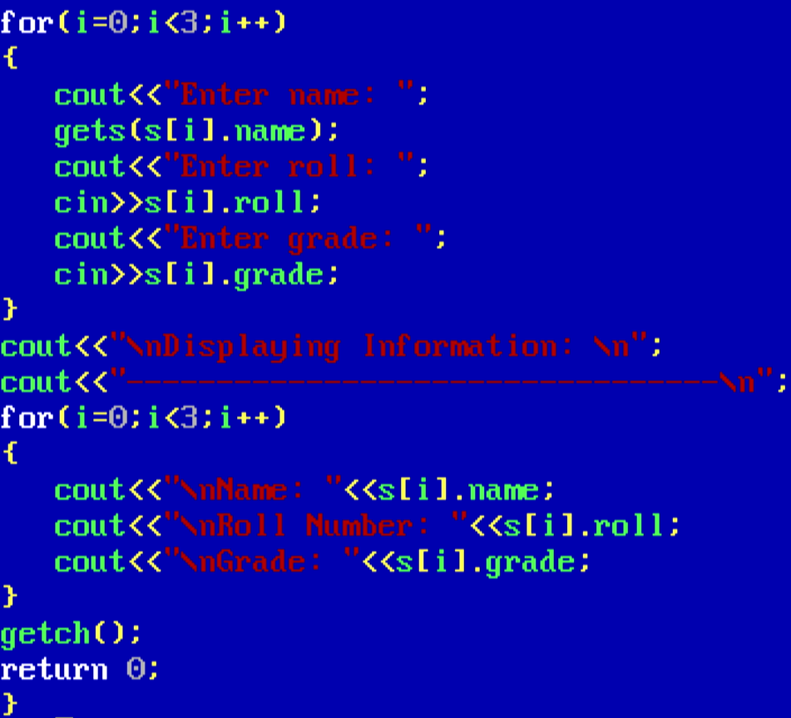
Output:
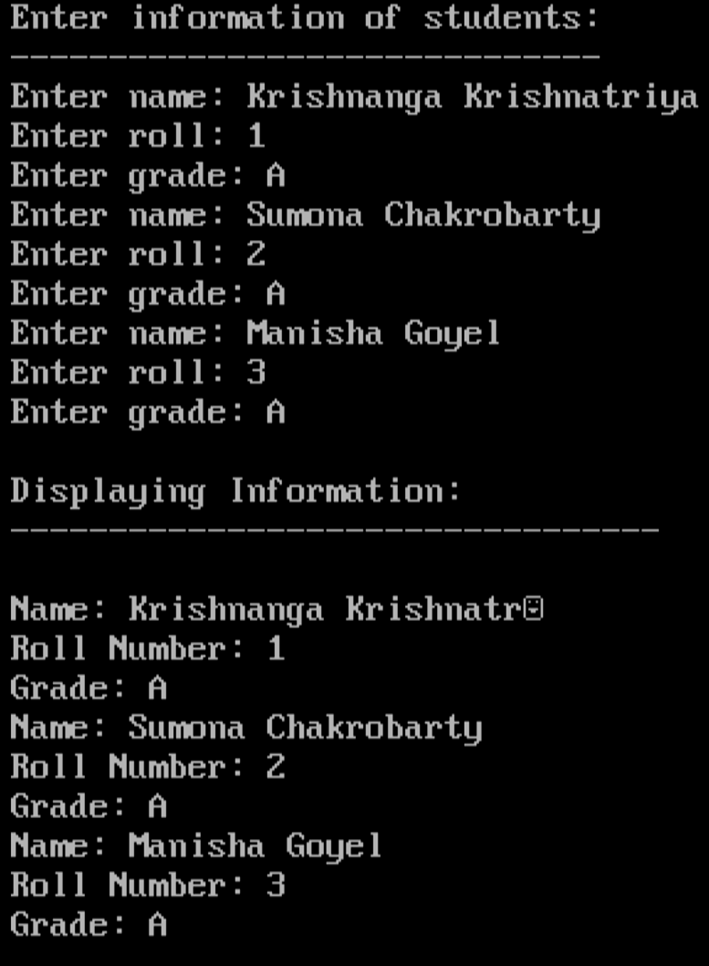
“I agree with your points, very insightful!”