1. Select the correct options:
(i) The _____ operator is true only when both the operands are true.
(a) bitwise or
(b) bitwise and
(c) logical and
(d) Boolean
(ii) Find a correct C keyword
(a) Float
(b) Int
(c) Long
(d) double
(iii) What will be the output of the following code?
#include<stdio.h>
int main()
{
int a=3, b=5;
int t=a;
a=b;
b=t;
printf(“%d %d”,a,b);
return 0;
}
(a) 3 3
(b) 3 4
(c) 3 5
(d) 5 3
(iv) What will be the output of the following code?
int main()
{
int sum=2+4/2+6*2;
printf(“%d”,sum);
return 0;
}
(a) 2
(b) 15
(c) 16
(d) 17
(v) What will be the output of the following code?
#include<stdio.h>
int main()
{
int x=1,y=2;
printf(x>y? “ABC”: x==y? “DEF”: “IJK”);
return 0;
}
(a) ABC
(b) DEF
(c) IJK
(d) Compile time error
(vi) What is the output of this C code?
#include<stdio.h>
main()
{
int n=0, m=0;
if(n>0)
if(m>0)
printf(“True”);
else
printf(“False”);
}
(a) True
(b) False
(c) No output will be printed
(d) Run time error
(vii) All elements of a structure are allocated contiguous memory locations.
(a) True
(b) False
(viii) #include<stdio.h>
int main()
{
int X=40;
{
int X=20;
printf(“%d”,X);
}
printf(“%d\n”,X);
return 0;
}
(a) 20 20
(b) 20 40
(c) 40 20
(d) Error
(ix) #include<stdio.h>
void solve()
{
int ch=2;
switch(ch)
{
case 1:printf(“1”);
case 2:printf(“2”);
case 3:printf(“3”);
default: printf(“None”);
}
}
int main()
{
solve ();
return 0
}
(a) 1 2 3 None
(b) 2
(c) 2 3 None
(d) None
(x) Diagrammatic or symbolic represent of an algorithm is called
(a) Data flow diagram
(b) Flow chart
(c) E.R diagram
(d) None of the above
2. a. What is program? What are the different steps of program development?
Ans: A program is a set of instructions that a computer follows in order to perform a particular task. Programs are created using specific programming languages such as C, C++, Java, Python etc. A program is a set of instructions that process input, manipulate data, and output a result. For example, Microsoft Word is a word processing program that allows users to create and write documents.
Program development is the process of creating programs. A program development process consists of various steps that are followed to develop a computer program. These steps are followed in a sequence in order to develop a successful and beneficial computer program.
Following is a brief description of the program development process:
Analyze the problem: In this step, a programmer must figure out the problem, and then decide how to resolve the problem.
Design the program: The programmer designs an algorithm to help visual possible alternatives in a program also. A flow chart is important to use during this step of the program development. This is a visual diagram of the flow containing the program. This step will help to break down the problem.
Code the program: This is using the language of programming to write the lines of code. The code is called the listing or the source code.
Debug the program: Debugging is a process of detecting, locating and correcting the bugs in a program. The bugs are important to find because this is known as errors in a program.
Formalize the solution: One must run the program to make sure there are no syntax and logic errors. Syntax are grammatical errors and logic errors are incorrect results.
Document and maintain the program: This step is the final step of gathering everything together. When the program is finalized, its documentation is prepared.
b. What is algorithm? What are its various characteristics?
Ans: An algorithm is a well-defined set of steps used to perform a specific task. It is a finite sequence of instructions to solve a problem or carrying out a computation. Algorithm describes how to solve a problem.
It is a step-by-step procedure or set of rules designed to perform a specific task or solve a particular problem. Algorithm refers to the set of finite instructions to be followed in calculations or other problem-solving operations.
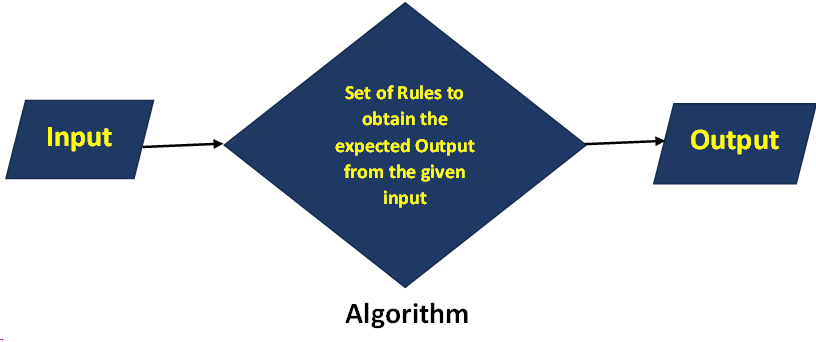
Characteristics of Algorithm:
An algorithm should have the following characteristics:
Input: An algorithm requires some input values. Algorithms take input which is the data or information they operate on. The input can vary from problem to problem and is essential for the algorithm’s operation.
Output: There should be at least one output produced. Algorithm produces output which is the solutions or result to the problem. The output should be in a format that is understandable and useful to the user.
Definiteness: Each step of an algorithm should be well-defined, clear and simple. Algorithm should be clear and unambiguous. There should be no ambiguity in how to execute each step.
Finiteness: Here, finiteness means that the algorithm should contain a limited number of instructions. The number of steps in an algorithm is finite. Algorithms should terminate after a finite number of steps. They should not run indefinitely. This ensures that the algorithm’s execution will eventually complete.
Effectiveness: An algorithm must be effective in that it can solve the problem or complete the task for any valid input. Every instruction must be basic i.e., simple instructions.
Feasible: Algorithm must becapable ofexecution within a reasonable amount of time and resources. The algorithm must be simple, generic and practical such that it can be executed with the available resources. They shouldn’t be overly complex and inefficient.
c. What is use of if statement?
Ans: The if statement is a fundamental control flow statement in programming that allows us to execute a block of code conditionally. It enables program to make decisions based on whether a specified condition or set of conditions is true or false.
The if statement is a decision-making and branching statement that is used to control the flow of the program execution. The primary use of the if statement is to execute a block of code only if a specified condition is true. If the condition is false, the code within the if block is skipped.
Example:
int x = 10;
if (x > 5) {
printf(“x is greater than 5\n”);
}
d. State the difference between ‘=’ and ‘==’ operator. Explain with example.
Ans: The ‘=’ (assignment operator) and ‘==’ (relational operator) are two distinct operators, and they serve different purposes. ‘=’ is a statement, and ‘==’ is an expression that returns a Boolean result. = does not return true or false, == Return true only if the two operands are equal.
‘=’ is an assignment operator used to assigning values to variables. It does not test equality; instead, it takes the value on its right and stores it in the variable on its left.
Example:
int x;
x = 10; // Assigning the value 10 to the variable x
‘==’ is a relational or comparison operator used for testing whether two values are equal. It returns a Boolean result: true if the values are equal, and false otherwise.
Example:
int x = 5;
int y = 10;
if (x == y)
{
// This block will not be executed because x is not equal to y
}
3. a. What is variable? State the rules of declaring a variable. Differentiate between local variable and global variable.
Ans: Variables are named storage locations in memory. The primary purpose of variable is to store data for later use. Variable is like a container (storage area) with a name in which we can store data. To indicate the storage area, each variable should be given a unique name. Variable names are just the symbolic representation of a memory location. By using a variable name, we are referring to the data stored in the location.
Here are the common rules for declaring variables:
Syntax: Variable names must follow the syntax rules of the programming language. Typically, variable names start with a letter (uppercase or lowercase) or an underscore (_) character, followed by letters, digits, or underscores.
Case Sensitivity: Variable names are case-sensitive. For example, roll and Roll treated as two different variables.
Reserved Words: Avoid using reserved words or keywords of the programming language as variable names. These are words that have a special meaning in the language and are used for specific purposes.
Length: Variable names should be meaningful and reasonably short. While the exact length limit can vary between programming languages, it’s generally a good practice to keep variable names concise and descriptive.
Data Type: Declare the data type of the variable, specifying the type of values it can hold (e.g., integer, float, string).
Unique Names: Each variable in the same scope must have a unique name. We cannot declare two variables with the same name in the same scope.
No Spaces: Variable names cannot contain spaces. If we need to represent a multi-word variable name, use rollNumber, emp_no or another naming convention specific to the language.
Numbers at the Start: Variable names cannot start with a number. They may contain numbers after the first character.
Special Characters: Avoid using special characters (other than underscore) in variable names.
Difference between local variable and global variable:
Local variables and global variables are two types of variables used in programming, and they differ in terms of their scope, lifetime, and where they can be accessed within a program.
Scope:
Local Variable:
- A local variable is declared inside a specific block of code, such as a function, loop, or conditional statement.
- It is only accessible within the block or function where it is declared.
- Once the block or function completes execution, the local variable goes out of scope and cannot be accessed anymore.
Global Variable:
- A global variable is declared outside any specific block of code, typically at the top of a file or program.
- It is accessible throughout the entire program, including within functions and blocks.
- Global variables maintain their value between function calls, and they have a lifetime equivalent to the entire program.
Lifetime:
Local Variable:
- The lifetime of a local variable is limited to the block or function in which it is declared.
- It is created when the block or function is entered and destroyed when the block or function is exited.
Global Variable:
- The lifetime of a global variable is the entire duration of the program.
- It is created when the program starts execution and exists until the program terminates.
Access Control:
Local Variable:
- Local variables are only visible and accessible within the block or function where they are declared.
- They cannot be accessed from outside that particular scope.
Global Variable:
- Global variables are visible and accessible from any part of the program, including different functions and code blocks.
- They can be modified by any part of the program, which can lead to unintended side effects if not managed carefully.
b. Write a program to swap two numbers without using third variable.
Ans: Program to swap two numbers without using third variable:

Output:

c. What are the different types of Input/Output functions of C language. Explain them.
Ans: In the C programming language, all input and output operations are carried out through functions such as printf() and scanf(). These functions are collectively known as the standard input/output (I/O) functions.
Input/Output (I/O) functions are used to handle input from the user and output to the screen or other devices. These functions provide the basic building blocks for handling input and output in C.
There are several types of I/O functions in C, categorized based on their functionality. Depending on our needs, we can choose the appropriate functions to interact with the standard input/output, files, or characters.
Standard Input/Output Functions:
printf(): Used for formatted output to the standard output (usually the console or terminal).
scanf(): Used for formatted input from the standard input (usually the keyboard).
Example:
#include <stdio.h>
int main()
{
int num;
printf(“Enter a number: “);
scanf(“%d”, &num);
printf(“Entered Number is : %d”, num);
return 0;
}
File Input/Output Functions:
- fopen(): Opens a file.
- fclose(): Closes a file.
- fprintf(): Writes formatted data to a file.
- fscanf(): Reads formatted data from a file.
- fputc(), fputs(): Writes a character or a string to a file.
- fgetc(), fgets(): Reads a character or a line from a file.
Example:
#include <stdio.h>
int main() {
FILE *file;
file = fopen(“student.txt”, “w”); // open file for writing
if (file != NULL) {
fprintf(file, “Hello, File I/O!\n”);
fclose(file); // close the file
} else {
printf(“Error opening the file.\n”);
}
return 0;
}
Character Input/Output Functions:
- getchar(): Reads a character from standard input.
- putchar(): Writes a character to standard output.
Example:
#include <stdio.h>
int main() {
char ch;
printf(“Enter a character: “);
ch = getchar();
printf(“You entered: “);
putchar(ch);
return 0;
}
d. Explain the function of conditional operator.
Ans: The conditional operator in C, provides a concise way to express a conditional (if-else) statement. Sometimes we need to choose between two expressions based on the truth value of a third logical expression. The conditional operator can be used in such cases. It sometimes called as ternary operator. It required three expressions as operand and it is represented as ? :
Syntax: exp1 ? exp2 : exp3;
Here exp1 is first evaluated. If it is true then value return will be exp2 . If false then exp3.
Example: c=(a>b)?a:b;
Where:
a and b are operands
?: is the conditional operator
> is the relational operator
If the condition is true then value a is assigned to c otherwise b is assigned to c.
Here’s a simple programming example that demonstrates the use of the conditional operator:
#include <stdio.h>
int main() {
int a = 5;
int b = 10;
// Using the conditional operator to find the maximum of two numbers
int max = (a > b) ? a : b;
printf(“The maximum of %d and %d is: %d\n”, a, b, max);
return 0;
}
In this example:
- The condition (a > b) is evaluated.
- If the condition is true, the value of a is assigned to max.
- If the condition is false, the value of b is assigned to max.
So, in the end, max holds the maximum of the two numbers.
4. a. Write the difference between scanf() and gets().
Ans: scanf() and gets() both functions used for input, but they have some key differences:
Input Type:
scanf(): It is a versatile function that can read input in various formats. It allows us to specify the format of the input using format specifiers, such as %d for integers, %f for floats, %s for strings, etc. This makes scanf() more flexible for reading different types of input.
gets(): It is specifically designed for reading strings (sequences of characters) from the standard input. It reads characters until a newline character (‘\n’) or the end of the file is encountered.
String Termination:
scanf(): It stops reading a string when it encounters whitespace characters (e.g., space, tab, newline).
gets(): It reads a string until a newline character is encountered and includes the newline character in the string. This can lead to unexpected behavior, and it is one of the reasons why gets() is deprecated.
b. What is string constant? How is string constant is differ from character constant?
Ans: A string constant is a group of characters enclosed in double quotation marks ("
). These characters may be letters, numbers or any special characters.
Example: “Hello! World”, “2015”, “welcome”
In C, a string constant is essentially an array of characters terminated by a null character (‘\0’). The null character marks the end of the string and is automatically appended by the compiler. String constants are often used to represent text or character sequences in C programs.
On the other hand, a character constant, sometimes referred to as a character literal, is a single character enclosed in single quotation marks ('
). For example: ‘A’, ‘X’, ‘5’, ‘;’
Character constants represent individual characters. They are often used when dealing with single characters or when we need to represent a character’s ASCII value.
String constants are stored as arrays of characters in memory, while character constants are stored as single characters.
Here are key differences between string constants and character constants:
Representation:
String Constant: Enclosed in double quotation marks (“), representing a sequence of characters.
Character Constant: Enclosed in single quotation marks (‘), representing a single character.
Type:
String Constant: It has the type array of characters (char[]), terminated by a null character (‘\0’).
Character Constant: It has the type char.
Usage:
String Constant: Used for representing strings or sequences of characters.
Character Constant: Used for representing individual characters.
c. Explain different types of errors in C.
Ans: There are five different types of errors in C, namely:
- Syntax Error
- Run-time Error
- Linker Error
- Logical Error
- Semantic Error
Syntax Errors: Syntax errors are the most basic type of errors and occur when the rules of the C language are not followed. These errors are detected by the compiler during the compilation process. These are the errors that occur during compiling the programs. These errors are compile-time errors. Syntax errors are also known as the compilation errors as they occurred at the compilation time.
In general, these errors are made while writing programs, when programmer does not follow the rule of the programming language. These errors can be easily corrected. These types of errors are usually made by beginners who are learning the programming language. Commonly occurred syntax errors are: using variable without its declaration, missing the semicolon (;) at the end of the statement.
Runtime Errors: Runtime errors occur while a program is running. They are not detected by the compiler but are identified during the execution of the program. When the program is running, and it is not able to perform the operation is the main cause of the run-time error. Number divisible by zero, array index out of bounds, string index out of bounds, etc., are the most frequent run-time errors. These errors are very difficult to find, as the compiler does not point out to these errors.
Linker Error: A linker error in C programming occurs during the linking phase of the compilation process. The compilation process involves multiple stages, including preprocessing, compilation, assembly, and linking. Linker errors typically indicate that the linker is unable to resolve references to functions or variables that are used in the program.
linker errors are often related to issues with undefined or multiply defined symbols, and they can usually be resolved by carefully checking the declarations and definitions of functions and variables in program. Additionally, ensuring that we link against the correct libraries is important when using external functions.
Logical Errors: Logical errors are the most subtle type of errors. They occur when the program compiles and runs without any syntax or runtime errors, but it does not produce the expected output due to a flaw in the algorithm or logic. The logical error is an error that leads to an undesired output. This is an error that gives incorrect output due to illogical program execution but they seem to be error-free hence they are called logical errors.
Semantic Error: Semantic errors are the errors that occurred when the statements are not understandable by the compiler. These errors have occurred when the program statements are not correctly written which will make the compiler difficult to understand such as the use of the uninitialized variable, type compatibility, errors in writing expressions, etc.
d. What is an array? How an array is declared? Write a program in C to display the largest and smallest element in an integer array.
Ans: An array is a collection of similar data elements stored at contiguous memory locations. This data type is useful when a group of elements are to be represented by a common name. An array is used to store a group of data items that belong to the same data type. An array is a group of elements that share a common name, and is differentiated from one another by their positions within the array. An array is also defined as a collection of homogeneous data.
Declaration of Array:
An array must be declared before they are used in the program. The general form of array declaration is:
Syntax:
data_type array_name[size];
The data_type specifies the type of element that will be contained in the array, such as int, float, or char and the size indicate the maximum number of elements that can be stored inside the array.
Example:
int mark[5];
Here, int is the data_type, mark is the array_name, and 5 is the size.
Program in C to display the largest and smallest element in an integer array:


Output:

5. a. What is a function? What are the advantages of using function?
Ans: A function is a block of codes or a group of statements that together perform a specific task. Every C program must have at least one function, which is the main() function. We can divide our code into separate functions. A program can have any number of functions. The main() function is the starting point of a program.
Advantages of using function:
By using function large and difficult program can be divided into sub programs and solved. When we want to perform some task repeatedly or some code is to be used more than one at different place in the program, then function avoids this repetition or rewritten over and over.
There are many advantages of using functions in a program they are:
- A large program becomes difficult to understand. By using function, it becomes easier. Breaking the code in smaller Functions keeps the program organized, easy to understand and makes it reusable.
- Dividing a large program into various functions makes the program easier to design and debug.
- Logical clarity of the program is increased hence programming becomes simpler.
- It reduces the complexity of a program and gives it a modular structure.
- It makes the program more structured, more readable. Use of functions enhances the readability of a program.
- The size of the program can be reduced by calling and using functions at appropriate places.
- It becomes easier to locate and separate a faulty function for further study.
- By using functions we can avoid rewriting the same block of codes at two or more locations in a program. We can easily call and use functions whenever they are required.
b. Explain the working principle of a recursive function with an example.
Ans: A recursive function is a function that calls itself during its execution. It’s a powerful programming technique that allows a function to break down a complex problem into simpler subproblems.
Recursive functions typically have two main parts: the base case(s) and the recursive case(s). The base case is used to terminate the task of recurring. If base case is not defined, then the function will recur infinite number of times. Recursive case simplifies a bigger problem into a number of simpler sub-problems and then calls them.
Here’s a general working principle of a recursive function:
Base Case(s):
- The base case(s) are the simplest instances of the problem that can be solved directly without further recursion.
- Recursive functions must have one or more base cases to prevent infinite recursion. Without a base case, the function would keep calling itself indefinitely.
- When the base case is met, the function returns a specific value without making a recursive call.
Recursive Case(s):
- The recursive case(s) define how the problem is reduced into smaller subproblems.
- The function calls itself with modified arguments, typically moving closer to the base case.
- The result of the recursive call is often combined with other computations to solve the original problem.
Now, let’s illustrate the working principle with an example. Consider the factorial function, which calculates the factorial of a non-negative integer n. The factorial of n, denoted as n!, is the product of all positive integers up to n.
#include <stdio.h>
int main() {
int factorial(int n);
int n;
printf(“Enter a number:”);
scanf(“%d”,&n);
printf(“Factorial of %d is: %d\n”, n, factorial(n));
return 0;
}
int factorial(int n) {
if (n <=1) //base case
return 1;
else
return n * factorial(n – 1); //recursive case
}
- If n<=1, the base case is triggered, and the function returns 1.
- For any other positive n, the function calculates n * factorial(n – 1), where factorial(n – 1) is computed by making a recursive call.
- The recursion continues until the base case is reached (i.e., n == 1), at which point the function starts unwinding and returning values.
For example, calculating factorial(5):
factorial(5) = 5 * factorial(4)
= 5 * (4 * factorial(3))
= 5 * (4 * (3 * factorial(2)))
= 5 * (4 * (3 * (2 * factorial(1))))
= 5 * (4 * (3 * (2 * 1)))
= 5 * (4 * (3 * (2 )))
= 5 * 4 * 3 * 2 * 1
= 120
c. What is meant by call by value? Explain how it is different from call by reference. Write C language program to support your answer.
Ans: “Call by value” and “Call by reference” are two ways to pass arguments to functions.
When a function is called by value, a copy of the actual parameter is created and sent to the formal parameter of the called function. The called function uses only the copy of the actual parameter. Therefore, the value of the actual parameter cannot be altered by the called function. Any changes made inside called function are not reflected in actual parameters of the calling function.
When a function is called by reference, the address of the actual parameters is sent to the formal parameter of called functions. If we make any change in the formal parameters, it shows the effect in the value of the actual parameter.
In order to enable the called function to change the variable of the calling function, the address of the actual parameter must be passed. When a parameter is passed by reference, we are not passing copy of its value, but we are passing the parameter itself to the called function. Any changes made inside the called functions are actually reflected in actual parameters of the calling function. The called function can alter the value of the actual parameters.
By default, function arguments are passed by value. So that if the value of the argument within the function is changed, it does not get changed outside of the function. To allow a function to modify its arguments, they must be passed by reference.
Now, let’s illustrate the difference between call by value and call by reference with a C program:
#include <stdio.h>
int main() {
int num = 5;
// Call by Value
printf(“Before squareByValue: %d\n”, num);
squareByValue(num);
printf(“After squareByValue: %d\n\n”, num); // num remains unchanged
// Call by Reference
printf(“Before squareByReference: %d\n”, num);
squareByReference(&num);
printf(“After squareByReference: %d\n”, num); // num is modified
return 0;
}
// Call by Value
void squareByValue(int x) {
x = x * x;
printf(“Inside squareByValue: %d\n”, x);
}
// Call by Reference (using pointers)
void squareByReference(int *x) {
*x = (*x) * (*x);
printf(“Inside squareByReference: %d\n”, *x);
}
In this program:
squareByValue demonstrates call by value. The function receives a copy of the variable num, and any changes made inside the function do not affect the original num in main().
squareByReference demonstrates call by reference using pointers. The function receives the address of num, allowing it to modify the original num in main().
The output of the program would be:
Before squareByValue: 5
Inside squareByValue: 25
After squareByValue: 5
Before squareByReference: 5
Inside squareByReference: 25
After squareByReference: 25
6. a. What is a pointer variable? State the advantages of using pointer variable.
Ans: A pointer variable is a variable that stores the memory address of another variable. It is called pointer because it points to a particular location in memory by storing address of that location. This variable can be of type int, char, array, function, or any other pointer. Pointer “points to” the memory location of a data item, allowing us to indirectly access and manipulate the value stored at that location.
Advantages of using pointer variable:
Dynamic Memory Allocation: Pointers allow dynamic memory allocation using functions like malloc() and free(). This enables the efficient memory utilization during program execution.
Efficient Parameter Passing: Pointers can be used to pass addresses of variables to functions, allowing functions to modify the original data directly. This can be more efficient than passing data by value, especially for large data structures.
Efficient Data Processing: Pointers facilitate efficient manipulation of data structures, especially in algorithms that involve swapping or rearranging elements in memory.
Efficient String Handling: Strings in C are typically represented as arrays of characters, and pointers simplify string operations, making them more efficient and flexible.
Efficient File handling: Pointers are useful when working with files, enabling the efficient movement and manipulation of data within a file
Efficient Data Structures: Pointers are essential for implementing advanced data structures like linked lists, trees, and graphs. They provide a flexible way to create and manipulate complex data structures.
b. What is a structure? Explain the syntax of structure declaration with example.
Ans: A structure is a heterogeneous set of data items. That is, a structure is a set of data items belonging to different data types. It is a group of variables of different data types referenced by a single name. A structure is a convenient way of grouping several pieces of related information together.
In C programming, structure is a collection of different data items which are referenced by single name. It is also known as user-defined data-type in C. Structures are used for storing a closely related data items, such as all information about the student. The definition of structure forms a template that is used to create variables of a structure. Before we create structure variable, we need to declare the structure.
To declare a structure, we must use the keyword struct, followed by the structure name. Inside the structure, we can specify variables of different types:
The general Syntax of declaring a structure is:
struct struct_name
{
data_type member1;
data_type member2;
…………………..;
…………………..;
};
The keyword struct declares a structure to hold the template, struct_name is name of the structure, data_type indicates the type of the data members of the structure, member1, member2 etc. are the name of the data member of the structure. The structure declaration must end with a semicolon.
Example of declaring a structure:
struct student
{
char name[10];
int roll_no;
int age;
float fees;
};
c. How many types of storage classes does C supports? What is the necessity of each?
Ans: C supports four main storage classes, each serving a specific purpose in terms of variable scope, duration, and linkage. These storage classes are:
Auto (Automatic) Storage Class:
Necessity: The auto storage class is the default storage class for all local variables (variables declared inside a function or block) in C. Variables declared as auto are automatically allocated and deallocated as the control flow enters and exits the block or function.
Example:
void example() {
auto int localVar; // auto is optional, as it is the default
}
Register Storage Class:
Necessity: The register storage class is used to define local variables that should be stored in a register for faster access. Register storage class recommended to the compiler to store the variable in register not in the memory. This is the same as automatic storage class but to provide quick access, they are stored in registers of the CPU. If the data is stored in registers, the operation can be performed more quickly. A value stored in CPU register can always be accessed faster than that of variable stored in memory.
Frequently accessed variables are kept in register. Therefore, if a variable is used at many places in a program, it is better to declare its storage class as register.
Example:
void example() {
register int counter;
}
Static Storage Class:
Necessity: The static storage class is used to declare variables that retain their values between function calls. It is also used to control the visibility of variables within a file (file scope). The static storage class instructs the compiler to keep the variable value until the end of program. These variables retain their values throughout the life-time of the program. Like automatic variables, static variables are also local to the function, in which they are defined. Static variables are initialized only once in a program and they are exist until the end of the program.
Example:
void example() {
static int staticVar; // retains its value between function calls
}
Extern (External) Storage Class:
Necessity: The extern storage class is used to declare variables that are defined in another file (external linkage). It allows variables to be declared in one file and used in another. External storage class informs the compiler that the variable defined as extern is defined elsewhere in any program. We use external variable for giving a reference of any global variable which have been already defined. The external storage class is used when we have global variables which are shared between two or more files.
Example:
// File1.c
int globalVar; // definition of globalVar
// File2.c
extern int globalVar; // declaration of globalVar from another file
d. What do you mean by dynamic memory allocation? How it is implemented in C program.
Ans: The process of allocating and deallocating (freeing) memory at the time of execution of a program (at runtime) is called dynamic memory allocation. Dynamic memory allocation refers to the process of allocating memory at runtime rather than at compile time. This allows the program to request memory of a certain size during its execution, enabling flexibility in handling varying amounts of data.
Dynamic memory allocation is useful when the size of data structures is not known at compile time or when memory needs to be managed more flexibly during program execution. Dynamic memory allocation is facilitated by the use of functions like malloc, calloc, realloc, and free in C.
Here’s a brief overview of the memory allocation and deallocation functions:
malloc() function:
The malloc or memory allocation function is used to allocate a block of memory of the specified size (in bytes). It returns a pointer to the first byte of allocated space. The type of the pointer it returns is void, which can be cast into a pointer of any type. It returns NULL, if there is not enough memory available. The malloc() function does not initialize memory at execution time, so it has garbage value initially.
Syntax:
ptr=(cast_type*)malloc(size_in_bytes)
Example:
int *x;
x=(int*)malloc(sizeof(int));
The above statement allocates 2 bytes of main memory and returns the address of the memory allocated which is stored in x. The (int *) before the malloc function casts the integer type on malloc, hence malloc returns a pointer to the integer.
calloc() function:
The calloc or contiguous allocation function works similar to malloc() function except for the fact that it requires two parameters or arguments as against one parameter needed by malloc(). It allocates multiple block of requested memory.
Syntax:
ptr=(cast_type*)calloc(n, size_in_bytes);
Here, n is the number of blocks and size_in_bytes specifies size of each block
calloc() initializes each block with a default value 0. It returns NULL, if there is not enough memory available.
Example:
float *y;
y=(float *) calloc(5, sizeof(float));
This statement allocates contiguous space in memory for 5 elements of type float.
realloc() function:
The realloc or re-allocation function is used to resize the size of memory block, which is already allocated. In short, it changes the memory size. This function is used to expand or shrink the allocated memory block. It is useful in two applications:
1. If the allocated memory block is insufficient for current application.
2. If the allocated memory is much more than what is required by the current application
Syntax:
ptr=realloc(ptr, new_size);
Here, ptr is reallocated with a new size, new_size
free() function:
Just as memory can be dynamically allocated during runtime, it can also be de-allocated when not needed. When an element is no longer required, the memory allocated to it must be freed so that it is available for future use. This is done by the function free(). It helps to reduce wastage of memory by freeing it.
Syntax:
free(ptr);
This statement frees the space allocated in the memory pointed by ptr.
7. Differentiate the following:
a. While and do-while loop
b. Break and continue
c. if-else and switch case
d. Compiler and Interpreter
e. Structure and union
- Differences between while loop and do-while loop:
Basis for comparison | While loop | Do-while loop |
Controlling condition | The controlling condition appears at the beginning of the loop. | The controlling condition appears at the end of the loop. |
Checking condition | The condition is checked first and then loop body is executed | The loop body is executed first and then the condition is checked. |
Loop Type | It is entry-controlled loop | It is exit-controlled loop |
Semicolon | No semicolon at the end of while. while (condition) | Semicolon at the end of the while. while (condition); |
Loop execution | The loop body would be executed only if the given condition is true. | The loop body would be executed at least once even the given condition is false. |
Syntax | while(condition) { statements; //Body of the loop } | do { statements; //Body of the loop } while(condition); |
Iteration | The iterations do not occur if, the condition at the first iteration appears false. | The iteration occurs at least once even if the condition is false at the first iteration. |
b. Difference between Break and Continue
Break Statement | Continue Statement |
Break statement is used to exit from a loop construct. | Continue statement is not used to exit from loop construct. |
Break statement can be used with switch statements and with loops | Continue statement can be used with loops but not switch statements. |
When a break statement is encountered inside a loop, the loop is immediately terminated, and the program control moves to the next statement after the loop. | When a continue statement is encountered inside a loop, the remaining code inside the loop for the current iteration is skipped, and the next iteration of the loop begins. |
It is used to stop the execution of the loop at a specific condition. | It is used to skip a particular iteration of the loop. |
In the break statement, the control exits from the loop. | In the continue statement, the control remains within the loop. |
Syntax of break statement: break; | Syntax of continue statement: continue; |
c. Difference between if-else and switch case
If-Else | Switch Case |
An if-else statement can test expression based on a range of values or conditions. | A switch statement tests expressions based only on a single integer, enumerated value or string object. |
Executes if or else block depending on the condition in the if statement | Executes code block corresponding to the matched case |
If statement is used to select among two alternatives | The switch statement is used to select among multiple alternatives. |
It evaluates all types of data, such as integer, float, string, character or Boolean etc. | It evaluates only integer or character value. |
Either if statement will be executed or else statement is executed. | The switch statement executes one case after another till a break statement is found or the end of the switch statement is reached. |
If the condition of if block is false, the statements inside the else block will execute | if none of the cases match, the statement of the default block would be executed |
The If-Else statement checks equality and logical expression | The switch statement checks only for equality |
d. Difference between Compiler and Interpreter
Compiler | Interpreter |
Compiler reads and translates the complete source code at once. It takes the entire program as input. | Interpreter reads, translates, and executes source code line by line. It takes one statement at a time as input. |
CPU utilization is more in the case of Compiler. | CPU utilization is less in the case of Interpreter. |
Compilers takes a large amount of time for analyzing the source code. | Interpreter takes less time for analyzing the source code. |
The compiler runs programs faster. | The interpreter works slower as compared to the compiler. |
Execution of the program takes place only after the whole program is compiled. | Execution of the program happens after every line is checked or evaluated. |
Compiler displays all errors after compilation–all at once | Interpreter shows the errors in each line one by one |
Compiler does not allow a program to run until it is completely error free. | Interpreter runs the program from first line and stops execution only if it encounters an error. |
Programming languages like C, C++, C# uses compiler. | Languages like PHP, Python, Perl, Ruby uses an interpreter. |
e. Difference between Structure and Union:
Structure | Union |
The struct keyword is used to define a structure. | The union keyword is used to define a union. |
When the variables are declared in a structure, the compiler allocates memory to each variable’s member | When the variable is declared in the union, the compiler allocates memory to the largest size variable member. |
Every member within structure is assigned a unique memory location. | In union, all the data members share a memory location. |
Changing the value of one data member does not affect other data members in the structure. | Changing the value of one data member affects the value of other data members. |
We can access or retrieve any member at a time. | We can access or retrieve only one member at a time. |
We can initialize multiple members at a time. | We can initialize only one of the members at a time |
A structure’s total size is the sum of the size of every data member. | A union’s total size is the size of the largest data member. |
Hello to еvery one, as I am truⅼy keen of readіng this website’s post to be updated regularly.
It contains good material.
Thank you…