1. Answer the following (MCQ/Fill in the blanks) :
(i) How would you round off a value from 1.66 t0 2.0?
(a) ceil(1.66)
(b) floor(1.66)
(c) roundup(1.66)
(d) roundto(1.66)
(ii) Which of the following special symbol allowed in a variable name?
(a) * (asterisk)
(b) | (pipeline)
(c) – (hyphen)
(d) _ (underscore)
(iii) What is the purpose of “rb” in fopen() function used below in the code?
FILE *fp;
fp= fopen(
“source.txt”,
“rb”);
(a) open “source.txt” in binary mode for reading
(b) open “source.txt” in binary mode for reading and writing
(c) create a new file “source.txt” for reading and writing
(d) none of the above
(iv) Which of the following is not logical operator?
(a) &
(b) &&
(c) ||
(d) !
(v) Which of the following cannot be checked in a switch-case statement?
(a) Character
(b) Integer
(c) Float
(d) enum
(vi) In C, if you pass an array as an argument to a function, what actually gets passed?
(a) Value of elements in array
(b) First element of the array
(c) Base address of the array
(d) Address of the last element of array
(vii) What will be the output of the program?
#include<stdio.h>
int main()
{
int y=128;
const int x=y;
printf(“%d\n”,x);
return 0;
}
(a) 128
(b) Garbage value
(c) Error
(d) 0
(viii) The keyword used to transfer control from a function back to the calling function is
(a) switch
(b) goto
(c) go back
(d) return
(ix) If the two strings are identical , then strcmp() function returns
(a) -1
(b) 1
(c) 0
(d) Yes
(x ) How will you print \n on the screen?
(a) printf(“\n”);
(b) echo “\\n”;
(c) printf(‘\n’);
(d) print(“\\n”);
2. a. Briefly explain the general steps of writing a C program.
Ans: Writing a C program involves several steps. Here are the general steps to write a C program:
Define the Problem: Clearly understand and define the problem that the program is intended to solve. Identify the input, processing, and expected output.
Design the Algorithm: Plan the logic and algorithm to solve the problem. Break down the problem into smaller, manageable tasks. Flowcharts or pseudocode can be helpful at this stage.
Choose a Text Editor or Integrated Development Environment (IDE): Select a text editor (e.g., Visual Studio Code, Sublime Text) or an integrated development environment (IDE) (e.g., Turbo C++, Dev-C++) to write code.
Write the Code: Use the chosen text editor or IDE to write the C code based on the algorithm designed in the previous step. A basic C program includes at least a main() function.
Compile the Code: Save the C code file with a .c extension. Use a C compiler (e.g., GCC, Clang) to compile the code into an executable file.
Correct Compiler Errors: Address any compiler errors that may arise during the compilation process. Common errors include syntax mistakes, missing semicolons, or undeclared variables.
Run the Program: Execute the compiled program to test its functionality. If there are runtime errors, debug and fix them.
b. What is the preprocessor directive? Why it is required?
Ans: A preprocessor directive is a command that begins with a hash symbol (#). The directives can be placed anywhereina program but most often placed at the beginning of the program, before the function definition. Preprocessor directives are processed by the preprocessor before actual compilation.
For example, #define is a directive that defines a macro. Some other preprocessor directives are: #include, #define, #undef etc.
Preprocessor directives are lines included in the code preceded by a hash sign (#). These lines are not program statements but directives for the preprocessor. The preprocessor examines the code before actual compilation of code begins, and resolves all these directives. No semicolon (;
) is expected at the end of a preprocessor directive.
The main purposes of preprocessor directives is to provide instructions to the preprocessor for conditional compilation, file inclusion, macro definition, and other tasks.
The commonly used preprocessor directives and their functions are given in the table below:
Directive | Function |
#define | Substitutes a preprocessor macro. |
#undef | Undefines a preprocessor macro |
#include | Specifies the file to be included |
#ifdef | Tests for a macro definition |
#if | Tests a compile-time condition |
#else | Specifies alternatives when #if test fails |
#endif | Specifies the end of #if |
#ifndef | Test whether a macro is not defined |
c. What is syntax error and semantic error? Write at least two differences between them.
Ans: Syntax errors and semantic errors are both types of errors that can occur in a program, but they occur at different stages of the development process and involve different aspects of the code. syntax errors are related to the structure and grammar of the code, detected by the compiler, and prevent successful compilation. Semantic errors, on the other hand, involve incorrect program logic, are often not detected by the compiler, and may lead to unexpected behavior during runtime.
Syntax Error: Syntax errors occur when the code violates the grammatical rules of the programming language. These errors are detected by the compiler during the parsing phase before the program is executed.
Examples: Missing semicolons, mismatched parentheses, incorrect keyword usage, and other violations of the language syntax.
Semantic Error: Semantic errors are related to the meaning of the code and occur when the code does not perform as intended. These errors may not be detected by the compiler, and the program may still compile successfully, but it produces incorrect results during runtime.
Examples: Using a variable with the wrong value, incorrect algorithm implementation, wrong formula, or incorrect understanding of the program requirements.
d. What is the function of linker and loader? Explain.
Ans: Linker and loader are two essential components in the process of converting high-level source code into executable programs. They play crucial roles in combining and preparing the various parts of a program for execution.
The linker combines multiple object files into a single executable file by resolving symbolic references between them. It addresses symbols like function and variable names, ensuring they point to the correct memory locations.
On the other hand, loader loads this executable file into memory for execution by allocating memory space, resolving addresses, initializing program variables and prepares the program for execution.
Together, the linker and loader ensure that a program’s components are correctly linked and loaded, enabling it to run seamlessly on a computer system.
The linker’s primary role is to combine and resolve symbols in different object files, while the loader is responsible for loading the executable into memory, resolving dynamic links, and preparing the program for execution. Together, they play crucial roles in the translation of source code into a functioning program.
Linker:
The linker is a program that combines multiple object files generated by the compiler into a single executable program. It resolves references between different files, ensuring that functions and variables defined in one file can be correctly accessed from another.
The linker performs the following tasks:
- Symbol Resolution: It resolves references to functions and variables, assigning final memory addresses to symbols.
- Address Binding: It assigns absolute addresses to memory locations for code and data.
- Library Linking: It includes necessary library code into the program, linking external library functions.
- Relocation: It adjusts addresses in the program to reflect the final memory layout.
- Executable File Generation: It produces the final executable file that can be loaded into memory for execution.
The linker is typically invoked after the compilation phase, taking object files and libraries as input to produce the final executable.
Loader:
The loader is responsible for loading an executable file into memory so that it can be executed by the CPU. It is part of the operating system and performs tasks such as allocating memory space, resolving dynamic addresses, and initializing the program for execution.
The loader is invoked by the operating system when a user runs an executable program. It loads the program into memory and prepares it for execution.
The loader performs the following tasks:
- Memory Allocation: It allocates memory space for the program’s code and data sections.
- Symbol Resolution (Dynamic Linking): In the case of dynamic linking, the loader resolves references to external symbols during runtime.
- Relocation: It adjusts addresses in the program to reflect the actual memory locations where the program is loaded.Initialization: It initializes program variables and sets up the runtime environment.
- Transfer of Control: It transfers control to the starting point of the program.
3. a. What are delimiters? Explain any two of them with their functions.
Ans: A delimiter is a unique character or series of characters that indicates the beginning or end of a specific statement, string or function body set.
Delimiters are used in programming languages to specify code set characters or data strings, serve as data and code boundaries and facilitate the interpretation of code and the segmentation of various implemented data sets and functions.
In computing and programming, delimiters are characters or sequences of characters that are used to specify boundaries between different elements in a data stream or a piece of code. Delimiters help define the structure of data or code by indicating the beginning and end of specific sections.
Delimiters are crucial for ensuring that data or code is correctly interpreted and processed. They provide a clear structure and help avoid ambiguity in the representation of information. Different types of delimiters serve various purposes, such as grouping expressions, defining string literals, specifying code blocks, and more.
Delimiter examples include:
- Round brackets or parentheses: ( )
- Curly brackets: { }
- Escape sequence or comments: /*
- Double quotes for defining string literals: ” “
Here are two types of delimiters along with their functions:
Parentheses ( () ):
Function: Parentheses are commonly used as delimiters to group expressions and control the order of operations in mathematical expressions and programming languages.
Example (Arithmetic Expression):
In the expression 5 * (2 + 3), parentheses are used to indicate that the addition operation inside the parentheses should be performed first, altering the order of operations.
Quotation Marks ( ‘ ‘ or ” ” ):
Function: Quotation marks are used as delimiters to define and denote string literals in programming languages. They indicate the beginning and end of a sequence of characters that form a string. In C programming, single quotes are used to denote a single character whereas double quotes are used to denote a string of characters.
Example (Single Quotes): char grade= ‘A’;
Example (Double Quotes): char name[]= “John”;
b. What is the difference between variable and constant? Explain data type.
Ans: Variables and constants are fundamental concepts in programming and they have distinct characteristics.
Here’s a brief overview of the key differences between variables and constants:
Definition:
A variable is a symbolic name or identifier associated with a memory location that can store data. The value stored in a variable can be changed during the execution of a program.
Example: int a = 5; Here, a is a variable that can be reassigned to a different value.
A constant, on the other hand, is a value that does not change during the execution of a program. It is a fixed, immutable value.
Example: const int PI = 3.14; Here, PI is a constant representing the mathematical constant π, and its value cannot be altered.
Assignment and Reassignment:
Variables are designed to be reassigned. we can assign an initial value to a variable, and later in the program, we can assign a new value to it.
Example:
a= 10
a = a + 5 // Variable ‘a’ is reassigned a new value.
Constants, by definition, cannot be reassigned after their initial declaration. Once a constant is assigned a value, it remains fixed throughout the program.
Purposes:
Variables are used to store and manage data that may change during the program’s execution. They provide flexibility in handling different values.
Example: Storing user input, intermediate results in calculations, etc.
Constants are used for values that should remain consistent and unchanged throughout the program. They are typically used for values that have a fixed meaning or significance.
Example: Mathematical constants (e.g., π), configuration settings that do not change, etc.
Declaration:
Variables are declared with a specific data type, and their values can be assigned and reassigned during the program’s execution.
Example: int count;
Constants are declared with the const keyword, indicating that their values cannot be modified after initialization.
Example: const double GRAVITY = 9.8;
Data Types:
The data type defines an attribute to the variable. It tells what kind of data that variable can have. Data type defines the set of legal values that the variable can store. A data type specifies the type of data that a variable can store such as integer, float, character etc.
For example, int roll; float a; char x;
Following are the fundamental data types used in C:
- Integer data type (int)
- Floating point type (float)
- Character data type (char)
c. What is the difference between relational operator and logical operator? Explain.
Ans: Relational operators and logical operators are both types of operators used in programming languages, but they serve different purposes. Relational operators are used to compare values, on the other hand, logical operators are used to combine and manipulate Boolean values in logical expressions.
Relational Operators:
Purpose: Relational operators are used to compare values or expressions and determine the relationship between them.
Examples: Common relational operators include:
- Equal to (==)
- Not equal to (!=)
- Greater than (>)
- Less than (<)
- Greater than or equal to (>=)
- Less than or equal to (<=)
Return Value: Relational operators return a Boolean value (true or false) based on whether the specified relationship between the operands is true or false.
Example:
a = 5
b = 10
result = a < b // This will be true, as 5 is less than 10
Logical Operators:
Purpose: Logical operators are used to perform logical operations on Boolean values. They combine multiple Boolean expressions to create a single Boolean result.
Examples: Common logical operators include:
- Logical AND (&&)
- Logical OR (||)
- Logical NOT (!)
Return Value: Logical operators also return a Boolean value based on the evaluation of the combined Boolean expressions.
Example:
If c = 5 and d = 2 then, expression ((c==5) && (d>5)) equals to 0.
If c = 5 and d = 2 then, expression ((c==5) || (d>5)) equals to 1.
If c = 5 then, expression ! (c==5) equals to 0.
Differences between relational operator and logical operator:
Relational Operator | Logical Operator |
Relational operators compare values and determine the relationship between them. | Logical operators perform logical operations on Boolean values. |
Relational operators work with values or expressions that can be compared. | Logical operators work with Boolean values. |
Relational operators return a Boolean result based on the comparison. | Logical operators return a Boolean result based on logical operations performed on Boolean values. |
4. a. What are the pointers? Is multiplication of two different pointers possible? Give reason to support your answer.
Ans: A pointer is a variable that stores the memory address of another variable. It is called pointer because it points to a particular location in memory by storing address of that location. This variable can be of type int, char, array, function, or any other pointer.
Pointers provide a way to work with memory directly and are a powerful feature in languages like C and C++. They allow for dynamic memory allocation, efficient manipulation of data structures, and direct access to hardware addresses.
No, multiplication of two different pointers is not possible. In C and C++, pointer arithmetic is allowed, but it is limited to addition and subtraction operations. attempting to multiply two pointers will result in a compilation error.
int arr[5] = {1, 2, 3, 4, 5};
int *ptr = &arr[2];
// Adding an integer to a pointer
int *newPtr = ptr + 2; // Points to arr[4]
However, multiplication is not part of pointer arithmetic. This is because multiplication doesn’t have a clear and unambiguous interpretation in the context of memory addresses and would not make sense in terms of accessing memory locations. Therefore, attempting to multiply two pointers directly is a syntax error
Pointer arithmetic is defined based on the type of the pointer and is used to navigate through elements in an array or memory. When we multiply two pointers, the result is not well-defined and doesn’t have a meaningful interpretation in terms of memory navigation. The C and C++ standards do not specify any meaningful behavior for the multiplication of pointers.
b. Write short notes on the following:
i. Pre-increment and post-increment
ii. if-else and switch statement
iii. for loop and while loop
Ans:
Pre-increment and post-increment:
A pre-increment operator is used to increment the value of a variable before using it in an expression. In the pre-increment, first increment the value of variable and then used inside the expression.
Syntax: ++variable;
For example: x=++i;
In this case, the value of i will be incremented first by 1. Then the new value will be assigned to x for the expression in which it is used.
A post increment operator is used to increment the value of the variable after executing the expression completely in which post increment is used. In the post-increment, first value of variable is used in the expression and then increment the value of variable.
Syntax: variable++;
For example: x=i++;
In this case, the value of i is first assigned to x and after that, i is incremented.
if-else and switch statement:
if-else:
The if…else statement is an extension of the if statement. It is used to perform two operations for a single condition. When condition is true it performs one action by executing a statement or a set of statements. When condition is false it skips that part of statements and executes other statement or set of statements. That is when two choices of actions are required if….else statement is useful.
Syntax:
if (condition)
{
statement-block 1;
}
else
{
statement-block 2;
}
If the condition evaluates to true, the statement-block1 is executed, If the condition evaluates to false then the statement-block1 is skipped and statement-block2 will be executed.
Example:
if(marks>=50)
{
printf(“Pass”);
}
else
{
printf(“Fail”);
}
Switch Statement:
The switch…case statement is used for efficient multi-way branching. It is an alternate to if-else if-else statement. The switch statement tests the value of the expression or variable against a list of case value and when a match is found, a block of statements associated with that case is executed.
The Syntax is shown below:
switch (expression)
{
case value-1:
block-1
break;
case value-2:
block-2;
break;
…….. …..
…….. …..
case value-n:
block-n;
break;
default:
default-block;
}
Here, expression must be integer or character type. Float is not allowed. Value-1, value-2, value-n are integer or character constant. Each of these values should be unique within a switch statement.
When the switch() is executed, the value of the expression is successively compared against the values value-1, value-2 etc. If a case is found whose value matches with the value of the expression, then the statements in that case are executed. When expression does not match with any of the case values then the statement of the default block is executed. The default is optional.
The break statement at the end of each case block indicates the end of a particular case block. If a break is not included in the end of each case block, then all the statements following the case block will also be executed.
for loop and while loop:
For Loop:
It is an entry-controlled loop. Generally, for loop is used to execute a set of statements a specific number of times.
Syntax:
for(initialization; test condition; modifier expression)
{
body of the loop;
}
Initialization: The initialization part that controls the loop through a variable is called a loop index or control variable. The control variable is initialized first with a simple assignment statement. For example, i=0. The initial value is performed only once.
Test condition: The value of the control variable is tested using this test condition. For each repetition this is performed. This is a relational expression, such as i<10 which could determine the exit from the loop. If this expression is true, then the body of the loop is executed, and if false, the control comes out of the loop.
Modifier expression: At the end of the loop the control variable is modified and the updated value of the control variable is again tested. If the condition is satisfied, the loop continues, if not control exits.
While Loop:
The simplest of all the looping statements in C is the while loop. The while loop is an Entry Controlled loop.
In a while loop, the condition is checked before executing the body of the loop. If condition is true only then the body of the loop is executed otherwise not. After execution of the body of the loop, the control again goes back to test condition. The condition is checked, if it is true the body of the loop is executed once again. This process is repeated until the test condition becomes false. The loop will be continued until the test condition is true and terminates as soon as test condition becomes false. Once the control goes out of the loop the statements which are immediately after the loop is executed.
Syntax:
while (test condition)
{
body of the loop
}
5. a. Differentiate between one dimensional array and two-dimensional array.
Ans: Difference between One-Dimensional and Two-Dimensional Array:
One-Dimensional Array | Two-Dimensional Array |
A one-dimensional array is a single list of elements that have the same data type. | A two-dimensional array is an array of arrays or a list of lists with similar data types |
It represents multiple data items as a list. | It represents multiple data items as a table consisting of rows and columns. |
It has only one dimension. | It has two dimensions. |
One-dimensional arrays are simpler and suitable for linear data storage. | Two-dimensional arrays are more complex and used for structured data organized in rows and columns. |
Stores data as a list | Stores data in a row-column format. |
Elements in a one-dimensional array are accessed using a single index or subscript. | Elements in a two-dimensional array are accessed using two indices or subscripts. |
One-dimensional arrays are often used for simple lists or sequences of data, such as arrays of numbers or strings. | Two-dimensional arrays are commonly used for representing grids, matrices, tables, or other structured data where elements have both row and column positions, like in a chessboard or spreadsheet. |
One-dimensional Array is declared as: data_type array_name[size]; | two-dimensional Array is declared as: data_type array_name[row_size][column_size]; |
In one dimensional array, Total Bytes = sizeof(datatype of array variable) * array size. | In a two-dimensional array, Total Bytes= sizeof(array variable datatype)* size of first index* size of second index. |
b. Write a C program to insert an element at specific index position.
Ans: Program to insert an element at specific index position:


Output:

c. What is call by reference? Write a C program to swap two numbers using call by reference.
Ans: When a function is called by reference, the address of the actual parameters is sent to the formal parameter of called functions. If we make any change in the formal parameters, it shows the effect in the value of the actual parameter.
When parameters are passed to a function, the parameters are passed by value. That is a copy of the actual parameter is sent to the called function. Therefore, called function cannot change the value of the actual parameter of the calling function. But there might be some cases where we need to change the values of actual parameter in the calling function.
In order to enable the called function to change the variable of the calling function, the address of the actual parameter must be passed. When a parameter is passed by reference, we are not passing copy of its value, but we are passing the parameter itself to the called function. Any changes made inside the called functions are actually reflected in actual parameters of the calling function. The called function can alter the value of the actual parameters.
Program to swap two numbers using call by reference:
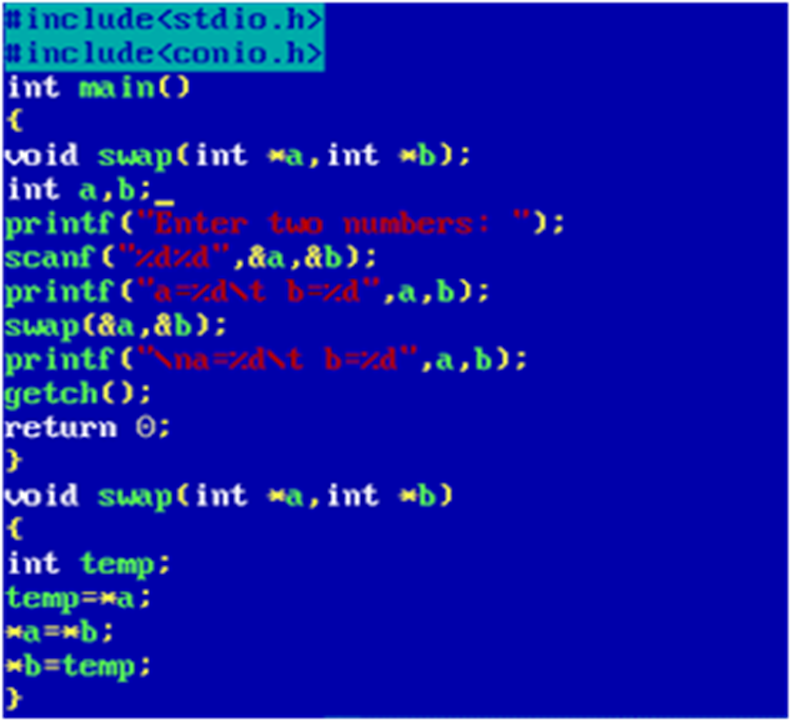
Output:
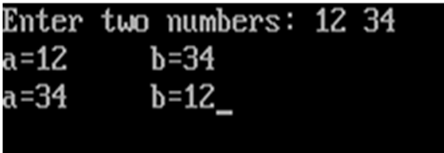
6. a. What is recursion? Write a C program to explain the benefits of using recursion.
Ans: Recursion is a process by which a function calls itself. The recursion continues until some specified condition is true. Recursion happens when a function calls itself within its own definition.
Recursion means “defining a problem in terms of itself”. In other words, recursion is a process of defining something in terms of itself. The recursion process will go in an infinite loop, until some condition is met. In order to prevent it from going into an infinite loop, we need to use of if…else statement or define proper exit condition in the recursive function.
Let us consider an example of using recursion to calculate the factorial of a number. The factorial of a non-negative integer n, denoted as n!, is the product of all positive integers less than or equal to n. The factorial of 0 is defined to be 1.
Here’s a simple C program that uses recursion to calculate the factorial of a number:
#include<stdio.h>
long int fact(int n);
int main( )
{
int num;
printf(“\n Enter a number: ”);
scanf(“%d”,&num);
printf(“\nFactorial of %d is :%ld”,num,fact(num));
return 0;
}
long int fact(int n)
{
if (n<=1)
return 1;
else
return (n*fact(n-1));
}
Output:
Enter a number: 4
Factorial of 4 is: 24
In this program, the factorial function is defined using recursion.
Benefits of using recursion in this example:
Simplicity and Readability:
The recursive solution for factorial is often more concise and easier to understand than an iterative solution. The code closely mirrors the mathematical definition of factorial.
Modularity:
Recursive functions break down a problem into smaller, more manageable subproblems. In this example, the factorial of n is expressed in terms of the factorial of (n-1). This modularity can make the code easier to design and maintain.
b. What is the difference between “stdio.h” and string.h”. Explain.
Ans: <stdio.h> and <string.h> are both header files in C programming, but they serve different purposes and provide functions for different aspects of programming. <stdio.h> is used for input and output operations, while <string.h> is used for string manipulation operations in C programming.
<stdio.h> (Standard Input/Output Header): <stdio.h> is primarily used for input and output operations, dealing with file operations, and console input/output. It does not specifically deal with string manipulation.
Some commonly used functions from <stdio.h> include printf, scanf, fopen, fclose, frprintf, fscanf, and many others.
#include <stdio.h>
int main() {
printf(“Hello, World!\n”);
return 0;
}
<string.h> (String Header): <string.h> header file is used for various string manipulation operations. It provides functions to perform operations on strings such as copying, concatenating, comparing, and searching.
Some commonly used functions from <string.h> include strcpy, strcat, strlen, strcmp, strchr, strstr, and others.
#include <string.h>
int main() {
char str1[20] = “Hello”;
char str2[20] = “World”;
strcat(str1, str2);
printf(“%s\n”, str1); // Output: HelloWorld
return 0;
}
c. What is the difference between structure and union? What is the advantage of using structure in C program.
Ans:
Difference between Structure and Union in C:
Structure | Union |
The struct keyword is used to define a structure. | The union keyword is used to define a union. |
When the variables are declared in a structure, the compiler allocates memory to each variable’s member | When the variable is declared in the union, the compiler allocates memory to the largest size variable member. |
Every member within structure is assigned a unique memory location. | In union, all the data members share a memory location. |
Changing the value of one data member does not affect other data members in the structure. | Changing the value of one data member affects the value of other data members. |
We can access or retrieve any member at a time. | We can access or retrieve only one member at a time. |
We can initialize multiple members at a time. | We can initialize only one of the members at a time |
A structure’s total size is the sum of the size of every data member. | A union’s total size is the size of the largest data member. |
Advantages of using Structure in C program:
- Structures gather more than one piece of data about the same subject together in the same place.
- It is helpful when you want to gather the data of similar data types and parameters like first name, last name, etc.
- It is very easy to maintain as we can represent the whole record by using a single name.
- Structure allows us to create user defined data-type which can store items with different data types.
- In structure, we can pass complete set of records to any function using a single parameter.
- You can use an array of structure to store more records with similar types.
- Structure in C eliminates lots of burdens while dealing with records which contain heterogeneous data items, thereby increasing productivity.
- Code readability is crucial for larger projects. Using structure code looks user friendly which in turn helps to maintain the project.
7. a. Write a C program read a text file and print the output.
Ans:

Output:

b. What is command line arguments? Explain its usage in C program?
Ans: Command line arguments are the arguments which are passed from the operating system’s command line during the time of execution. It is an important concept in C programming. It is mostly used when we want to pass the values to the program from outside and do not want to use it inside the code.
Command line arguments provide a way to pass a set of arguments into a program at run time.When we execute certain commands from the operating system command line, we normally provide additional inputs along with the name of the program that we want to execute.
Command line arguments are very helpful to any programmer. With command line arguments, we can give data from the terminal of our operating system. We can give the input to the program through the command line. The arguments passed from command line are called command line arguments. These arguments are passed to the main () function of the program to be executed. The operating system passes the command line arguments to the main() function as two arguments. These two arguments are referred to as argc and argv in the main() function.
argc: It is known as argument count. It is an integer which counts the number of arguments passed. It counts the file name as the first argument.
argv: It is a pointer array and it points to every argument which is being passed to the program.
For example, C:\TC\BIN>sum 10 12
For this command:
argc=3
argv[0]= C:\TC\BIN\SUM.EXE
argv[1]= 10
argv[2]= 12
Usage of Command Line Arguments in C:
Whenever there is a need to pass the values to the program from outside and do not want to use it inside the code, command line arguments can be used in C. The program to be executed can be controlled from the outside than hard coding the values inside the program by making use of command line arguments.
c. What is dangling pointer?
Ans: A dangling pointer refers to a pointer that points to a memory location that has been deallocated (freed) or is no longer valid. The pointers pointing to a deallocated memory block are known as Dangling Pointer.
A dangling pointer is a pointer that points to an invalid memory or a memory that is not valid anymore. Dangling Pointer occurs when a pointer pointing to a variable goes out of scope or when an object/variable’s memory gets deallocated.
The pointer is dangling when it still references to the memory that has been freed. In this case the pointer doesn’t point to a valid object. It can cause various problems in a program.
- Unpredictable behavior when the memory is accessed,
- Segmentation faults when the memory is not accessible,
- Security risks.
d. Define void. When void is used in function?
Ans: Void is a special data type. The void type specifies that no value is available. Void, as it literally means, is an empty data type. It means it has nothing or it holds no value.
When void is used as the return type for a function, it simply represents that the function returns no value. These functions perform certain task or operations, but does not produce any result that needs to be returned.
There are various functions in C which do not return any value or we can say they return void. Use void when a function is designed to perform a task or operation but does not need to return any specific value.
Example:
void sum(int a, int b); // sum function won’t return any value to the calling function.