1. Answer the following questions:
(i) Which one of the following is not a Java feature?
(a) Object-oriented
(b) Use of pointers
(c) Portable
(d) Dynamic and extensible
(ii) Which of the following is not an OOPs concept in Java?
(a) Polymorphism
(b) Inheritance
(c) Compilation
(d) Encapsulation
(iii) Which of the following is a type of polymorphism in Java Programming?
(a) Multiple polymorphism
(b) Compile time polymorphism
(c) Multilevel polymorphism
(d) Execution time polymorphism
(iv) Which one of the following is not an access modifier?
(a) protected
(b) void
(c) public
(d) private
(v) What is false about constructor?
(a) Constructors cannot be synchronized in Java.
(b) Java does not provide default copy constructor.
(c) Constructor can have a return type.
(d) “this” and “super” can be used in a constructor.
(vi) Which of this keyword can be used in a child class to call the constructor of parent class
(a) super
(b) this
(c) extent
(d) extends
(vii) Which of these keywords can be used to prevent Method overriding?
(a) static
(b) constant
(c) protected
(d) final
(viii) Which feature of OOP indicates code reusability?
(a) Abstraction
(b) Polymorphism
(c) Encapsulation
(d) Inheritance
(ix) The feature by which one object can interact with another object is
(a) Message reading
(b) Message Passing
(c) Data transfer
(d) Data Binding
(x) Which keyword among the following can be used to declare an array of objects in Java?
(a) allocate
(b) arr
(c) new
(d) create
2. Answers the following questions.
(a) “public static void main(String args[])”. Why the static keyword is used with the main function?
Ans: The static keyword is used for the main method to allow it to be called without creating an instance of the class. The main method is the starting point of a Java program, and it needs to be accessible at the class level, not tied to any specific instance. The static keyword ensures that the method is available to be called at the class level, even before any objects of the class are created.
The main must always be declared as static since the interpreter uses this method before any objects are created. The static keyword is used in the main method to make it a class-level method that can be called without creating an instance of the class. This is essential for the JVM to invoke the main method to start the execution of the Java program.
(b) Distinguish between the following:
(i) Object and Class
(ii) Abstraction and Encapsulation
Ans:
Difference between Objects and Class:
Object | Class |
An Object is an instance of a class. | A Class is a blueprint or template of objects. It is used to declare and create objects. |
Object is a real-world entity such as book, pen, etc. | A Class is a group of similar objects. |
An Object is a physical entity. | Class is a logical entity. |
Object represents a specific entity or instance. | Class describes the properties and behaviors of objects |
Object is created through new keyword | Class is declared with the class keyword. |
Memory is allocated as soon as an object is created. | No memory is allocated when a class is declared. |
Each and every object has its own values, which are associated with the fields. | Class doesn’t have any values which are associated with the fields. |
An object cannot be inherited by other objects. | A class can be inherited by other classes. |
A class can be used to create many objects. | We can declare a class only once. |
Objects can be manipulated. | Classes can’t be manipulated as they are not available in memory. |
Difference between Abstraction and Encapsulation:
Abstraction | Encapsulation |
Data abstraction hides the implementation details and shows only the functionality to the user in order to reduce the code complexity. | Encapsulation binds or wraps the data and methods together into a single unit and hides the details for data protection. |
Abstraction is hiding the details and implementation of the code. | Encapsulation is hiding the data and controlling the visibility of the code. |
Abstraction is the process of gaining relevant and selective information from a larger data pool. | Encapsulation is the method of holding up data as a single entity. |
Abstraction is the process of focusing on essential features and ignoring unnecessary details. | Encapsulation, on the other hand, is the process of hiding the internal details of an object from the outside world. |
Abstraction is a design level process. | Encapsulation is an implementation level process. |
Abstraction is concerned about what a class instance can do, instead of the implementation of the class. | Encapsulation helps in data binding and control over maintaining the transparency of the data. |
We can implement abstraction using abstract class and interfaces. | Encapsulation can be implemented using by access modifier i.e. private, protected and public. |
Abstraction simplifies the structure of a system. | Encapsulation protects its integrity. |
(c) What is a byte code in Java? Explain the concept of platform independent in Java?
Ans: In Java, bytecode refers to the intermediate code that is generated by the Java compiler from the source code. Instead of generating native machine code for a specific platform, the Java compiler produces bytecode, which is a set of instructions for a virtual machine called the Java Virtual Machine (JVM). This bytecode is a low-level representation of the source code that is platform-independent.
Java is known as platform-neutral language or platform-independent language because of its design philosophy “Write Once, Run Anywhere”. “Write Once, Run Anywhere” (WORA), is a fundamental concept in Java Programming. This means that once we write a Java program, we can run it on any device or platform that has a Java Virtual Machine (JVM) installed, without modification.
Platform Neutral or Platform Independent means that while writing the program in java, we don’t need to care about on which Machine or platform the program will going to be executed. Java is platform-neutral language because it is designed to run on any operating system or hardware platform. Java’s platform neutrality is achieved through the combination of bytecode and the Java Virtual Machine, allowing Java applications to run on diverse platforms without modification.
Java is considered to be architecturally neutral because it is designed to be platform independent. Architecture neutrality means that the code written in Java is independent of the underlying hardware or operating system. As a result, any code written in Java can be compiled and run on any machine, regardless of what type of processor or operating system it uses. This makes the Java platform independent.
This platform independence is achieved through the use of the Java Virtual Machine (JVM), which acts as an intermediary between the code and the underlying operating system. When we compile a Java program, it is not compiled directly into machine code for a specific computer or operating system. Instead, it is compiled into an intermediate code called byte code. The bytecode is executed by the JVM, which is specific to each platform.
The JVM interprets bytecode, which is the compiled form of the Java code, and translates it into machine code that can be executed by the target platform’s hardware. This means that the same Java code can be run on different platforms without any need for modification or recompilation. Bytecodes are effectively platform-independent. The Java virtual machine takes care of all hardware-related issues so that no code has to be compiled for different hardware. The JVM serves as a layer of abstraction between the Java code and the underlying hardware and operating system.
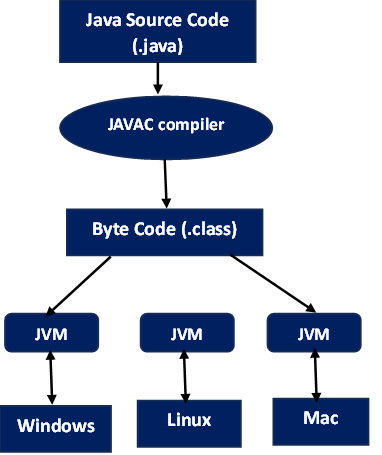
(d) What is the difference between this() and super() in Java?
Ans:
this():
this() can beused to invoke a constructor of the same class. Inside a constructor, we can use this() to call another constructor within the same class. It is often used to avoid code duplication when there are multiple constructors in a class.
- this() is used with constructors only.
- It refers to the constructor of the same class whose parameters matches with the parameters passed to this(parameters).
- No Dot(.) operator is used. Only the matching parameters are passed.
- It is used to refer to the constructor belonging to the same class.
Example:
public class student {
private String name;
private int age;
// Constructor 1 with String as parameter.
public student(String name)
{
// This line of code calls the second constructor.
this(18);
System.out.println(“Name of student : ” + name);
}
// Constructor 2 with int in parameter.
public student(int age)
{
System.out.println(“Age of student = ” + age);
}
// Constructor 3 with no parameters.
public student()
{
// This line calls the first constructor.
this(“John”);
}
public static void main(String[] args)
{
// This calls the third constructor.
student std = new student();
}
Output:
Age of student = 20
Name of student : John
super():
super() is used to invoke a constructor of the immediate parent class (super class). Inside a subclass constructor, we can use super() to call a constructor in the superclass. It is used to initialize the inherited members from the superclass.
The syntax used to call superclass constructor is
super([arguments])
Here, the arguments enclosed in square brackets are optional. The statement super() invokes the constructor with no parameters of its superclass and the statement super (arguments) invokes the superclass constructor that matches the arguments.
Example:
class parent{
parent(){
System.out.println(“Parent constructor called.”);
}
}
class child extends parent{
child(){
super();
System.out.println(“Child constructor called.”);
}
}
class Test {
public static void main( String args[] ) {
child obj = new child();
}
}
Output:
Parent constructor called.
Child constructor called.
3. Answers the following questions.
a. What is a variable? What is the difference between an instance variable and a local variable? Explain with suitable Java program.
Ans: A variable is a named storage location that holds a value, which can be modified during the execution of a program. Variables are used to store and manipulate data in a program. In Java, variables must be declared with a specific data type before they can be used.
A variable is an identifier that denotes a storage location used to store a data value. Unlike constants that remain unchanged during the execution of a program, a variable may take different values at different times during the execution of the program.
Difference between an instance variable and a local variable:
Instance variables are associated with objects and have a separate copy for each object, while local variables are temporary and only exist within the scope in which they are declared.
Instance Variable:
- An instance variable is a variable that is declared within a class but outside any method, constructor, or block.
- Each object of the class has its own copy of the instance variable.
- Instance variables are initialized when an object is created.
- They are used to represent attributes or properties of an object.
Local Variable:
- A local variable is a variable that is declared within a method, constructor, or block.
- Local variables are only accessible within the scope in which they are declared.
- They must be initialized before use.
- Local variables are typically used for temporary storage.
Example:
public class MyClass {
// Instance variable
int instanceVar;
// Method with a local variable
public void myMethod() {
// Local variable
int localVar = 5;
// Accessing instance and local variables
System.out.println(“instanceVar: ” + instanceVar); // Accessing instance variable
System.out.println(“localVar: ” + localVar); // Accessing local variable
}
public static void main(String[] args) {
// Creating an object of MyClass
MyClass obj = new MyClass();
// Modifying the instance variable
obj.instanceVar = 10;
// Calling the method with local variable
obj.myMethod();
}
}
b. What are the characteristics of an object-oriented programming? How is it different from procedure-oriented programming? Give a comparison between the two approaches of programming.
Ans: Object-Oriented Programming (OOP) is a programming paradigm that uses objects, which are instances of classes, for organizing and structuring code. OOP is centered around the concept of “objects,” which encapsulate data and behavior.
Here are some key characteristics of Object-Oriented Programming:
Encapsulation: Encapsulation refers to the bundling of data (attributes) and the methods (functions) that operate on the data into a single unit, known as a class. It helps in hiding the internal implementation details of an object and exposing only what is necessary.
Abstraction: Abstraction is the process of simplifying complex systems by modeling classes based on the essential properties and behaviors they possess. It involves focusing on the relevant characteristics of an object while ignoring the irrelevant details.
Inheritance: Inheritance allows a new class (subclass or derived class) to inherit attributes and methods from an existing class (base class or superclass). It promotes code reuse and helps in creating a hierarchy of classes with shared characteristics.
Polymorphism: Polymorphism allows objects of different classes to be treated as objects of a common base class.It enables a single interface to represent entities of different types and provides a mechanism to override or extend the behavior of methods in derived classes.
Classes and Objects: Classes are blueprints or templates for creating objects. They define the properties and behaviors that objects of the class will have. Objects are instances of classes, and each object has its own unique state (attributes) and behavior (methods).
Message Passing:In OOP, objects communicate with each other by sending and receiving messages. A message is a request for an object to perform one of its methods.
Modularity: OOP promotes modularity by breaking down a complex system into smaller, more manageable parts (classes). Each class represents a module, and changes made to one module do not affect the entire system.
Dynamic Binding: Dynamic binding, also known as late binding, allows the selection of the appropriate method implementation at runtime rather than at compile time. This contributes to flexibility and adaptability in the code.
Difference between Object-Oriented Programming and Procedure-Oriented Programming:
Object-oriented programming (OOP) and procedure-oriented programming (POP) are two different programming paradigms with distinct characteristics. OOP emphasizes the organization of code around objects, promoting encapsulation, inheritance, polymorphism, and abstraction. POP, on the other hand, focuses on procedures and functions, with an emphasis on step-by-step execution of algorithms.
Procedure Oriented Programming | Object Oriented Programming |
Procedure oriented programming is based on a sequence of procedures or instructions. | Object-oriented programming is based on the concept of objects. |
POP follows the Top-Down approach. | OOP follows the Bottom-Up approach |
In POP, data and functions are separate. | In OOP, data and functions are encapsulated into a single unit. |
In POP, the program is divided into small parts called functions. | In OOP, the program is divided into parts called objects. |
POP does not have any access specifier. | OOP has access specifiers named Public, Private, Protected, etc. |
POP does not have any proper way for hiding data so it is less secure. | OOP provides data hiding so it is more secure |
Modifying the existing code is not easy at all | The existing code can easily be modified because of the modular approach in the code. |
In POP overloading is not possible. | Overloading is possible in OOP. |
In POP, there is no concept of data hiding and inheritance. | In OOP, the concept of data hiding and inheritance is used. |
Comparison between object-oriented programming and procedure-oriented programming:
Data and Functionality:
- OOP combines data and functionality into objects, promoting encapsulation.
- POP separates data and functions, focusing on procedures that manipulate the data.
Code Reusability:
- OOP provides code reusability through mechanisms like inheritance.
- POP achieves code reuse through functions, but it may not be as flexible as inheritance.
Ease of Maintenance:
- OOP is often considered more modular and easier to maintain due to encapsulation and abstraction.
- POP may become complex as the program size increases, and changes may require modifications in multiple procedures.
Flexibility and Extensibility:
- OOP is more flexible and extensible through features like polymorphism and inheritance.
- POP may require more effort to adapt to changing requirements, and adding new features might involve modifying existing procedures.
Real-World Modeling:
- OOP allows for real-world modeling by representing entities and their interactions.
- POP is more focused on algorithms and may not provide a natural way to model real-world entities.
c. What is an array? How can you declare and create an array in Java? Explain with suitable Java program.
Ans: An array in Java is a data structure that allows us to store multiple values of the same data type under a single name. Each element in the array is identified by an index, and the index starts from 0. Arrays provide a convenient way to work with collections of data.
Declaration and Creation of an Array in Java:
To declare an array in Java, you specify the type of the elements followed by the array name and square brackets. Memory for the array is allocated using the new
keyword, specifying the size of the array.
We can declare and create an array in Java using the following syntax:
// Declaration
dataType[] arrayName;
// Creation
arrayName = new dataType[arraySize];
Example:
public class ArrayExample {
public static void main(String[] args) {
// Declaration and creation of an array of integers
int[] myArray = new int[5];
// Initialization of array elements
myArray[0] = 10;
myArray[1] = 20;
myArray[2] = 30;
myArray[3] = 40;
myArray[4] = 50;
// Accessing and printing array elements
System.out.println(“Element at index 0: ” + myArray[0]);
System.out.println(“Element at index 1: ” + myArray[1]);
System.out.println(“Element at index 2: ” + myArray[2]);
System.out.println(“Element at index 3: ” + myArray[3]);
System.out.println(“Element at index 4: ” + myArray[4]);
}
}
In this program:
- We declare an array of integers named myArray that can hold 5 elements.
- We initialize the array elements with values 10, 20, 30, 40, and 50.
- We then access and print the elements using their respective indices.
Output:
Element at index 0: 10
Element at index 1: 20
Element at index 2: 30
Element at index 3: 40
Element at index 4: 50
4. Answer the following questions.
a. What is default constructor in Java? How it is different from parameterized constructor?
Ans: In Java, a default constructor is a constructor that is automatically created by the compiler if a class doesn’t explicitly define any constructor. It is a constructor with no parameters or one that has all parameters provided with default values. If a class does not contain any constructor, Java automatically provides a default constructor.
Here’s an example of a class with a default constructor:
public class MyClass {
// Default constructor
public MyClass() {
// Initialization or setup code (if needed)
}
// Other methods and members can be defined here
}
If we don’t provide any constructor in the class, the Java compiler will insert a default constructor during compilation.
On the other hand, a parameterized constructor is a constructor that accepts parameters, allowing us to initialize the object’s state with specific values at the time of creation. Unlike the default constructor, a parameterized constructor explicitly takes one or more parameters.
While the default constructor is automatically provided if not explicitly defined, the parameterized constructor allows us to provide specific values during object creation, offering more flexibility in initializing object state.
Here’s an example of a class with a parameterized constructor:
public class Person {
String name;
int age;
// Parameterized constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Other methods and members can be defined here
}
In this example, the Person class has a parameterized constructor that takes a name and an age as parameters. When an object of the Person class is created using this constructor, we can initialize the object with specific values.
Differences between default constructor and parameterized constructor:
Parameters:
- The default constructor has no parameters.
- The parameterized constructor explicitly takes one or more parameters.
Initialization:
- The default constructor typically initializes the object with default values or performs minimal setup.
- The parameterized constructor allows us to initialize the object with specific values provided as arguments.
Implicit vs. Explicit:
- The default constructor is automatically provided by the compiler if no constructors are defined in the class.
- The parameterized constructor is explicitly defined in the class and can be customized based on the specific requirements of object initialization.
b. What do you mean by polymorphism in Object-Oriented Programming? Explain the concept of method overloading with suitable Java program.
Ans: Polymorphism in object-oriented programming (OOP) refers to the ability of a single entity, such as a method or class, to take on multiple forms. In Java, polymorphism is achieved through two mechanisms: method overloading and method overriding.
Method overloading is a type of polymorphism that allows a class to have multiple methods with the same name but different parameter lists (different in terms of the number or type of parameters). The compiler determines which method to invoke based on the number and types of arguments passed to it. Method overloading enhances code readability and provides flexibility by allowing the same method name to be used for different purposes within the same class. Method overloading is determined at compile time (compile-time polymorphism) based on the method signature, not at runtime.
Example:
public class overloading {
// Method to add two integers
public int add(int a, int b) {
return a + b;
}
// Method to add three integers
public int add(int a, int b, int c) {
return a + b + c;
}
// Method to add two doubles
public double add(double a, double b) {
return a + b;
}
// Method to concatenate two strings
public String add(String a, String b) {
return a + b;
}
public static void main(String[] args) {
overloading obj = new overloading();
System.out.println(obj.add(5, 10)); // Calls first add method
System.out.println(obj.add(5, 10, 15)); // Calls second add method
System.out.println(obj.add(2.5, 3.5)); // Calls third add method
System.out.println(obj.add(“Hi”,”Raj”)); //Calls fourth add method
}
}
Output:
15
30
6.0
HiRaj
In this example, the overloading class has multiple add methods, each with a different parameter list. The compiler decides which method to call based on the arguments provided during the method invocation.
c. What is a constructor? What is the use of a constructor?
Ans: A constructor in Java is a special type of method that is used to initialize objects. It is called when an object of a class is created, and its purpose is to set initial values for the object’s attributes or perform any other setup operations that are necessary for the object to function properly. Constructors play a crucial role in ensuring that objects are properly initialized before they are used in a program.
Constructors in java is as similar to methods in Java. It invokes automatically at the time of initialization or the object creation. It has the same name as of class name. It is called when an instance of the class is created. we don’t need to call it manually or we can say that the constructor is the method that can be invoked using the new operator at the time of object creation.
Key points about constructors:
- Constructors have the same name as the class they belong to.
- They don’t have a return type, not even void.
- The main purpose of a constructor is to initialize the object’s state.
- Constructors are automatically called when an object is created using the new keyword.
- Like regular methods, constructors can be overloaded. This means a class can have multiple constructors with different parameter lists.
Example:
public class student {
String name;
int roll;
// public constructor
public student() {
name = “John”;
roll=1;
}
}
class college {
public static void main(String[] args) {
student s1 = new student();
System.out.println(“Student Name = ” + s1.name);
System.out.println(“Roll Number = ” + s1.roll);
}
}
Output:
Student Name = John
Roll Number = 1
d. Name the default package name in Java. Give at least a class name which belongs to the default package.
Ans: In Java, two packages java.lang package and a no-name package (also called default package) are imported by default in all the classes of Java. Default Package doesn’t have a name but it is present by default and thus, it is named the default package. Java Virtual Machine imports these packages by default in Java internally.
The default package in Java doesn’t have a name and is a collection of classes whose source files do not contain a package declaration. When we don’t mention package declaration and directly create a class it is placed in the default package or no-name package of Java. This default package is also imported along with java.lang every time a java class file is created.
The java.lang package provides fundamental classes of the Java Programming language design. The two important classes Object and Class, instances of which represent classes at runtime are part of this package.
In Java, the default package doesn’t have a name. Classes in the default package don’t belong to any named package. When you don’t specify a package for your classes, they automatically become part of the default package.
Here’s an example of a class belonging to the default package:
// This class belongs to the default package
public class HelloWorld {
public static void main(String[] args) {
System.out.println(“Hello, World!”);
}
}
// This class belongs to the default package
public class HelloWorld {
public static void main(String[] args) {
System.out.println(“Hello, World!”);
}
}
In this example, the HelloWorld class is part of the default package since no package is specified.
5. Answer the following questions.
a. Define an interface in Java. Explain the use of interface using suitable Java program.
Ans: In Java, an interface is a collection of abstract methods. An interface in Java is similar to a class but cannot have any method implementations or instance variables. It provides a way to achieve abstraction in Java, allowing you to define a contract that classes must adhere to without specifying how they implement it. Classes implement interfaces by providing concrete implementations for the methods declared in the interface.
Using interfaces allows us to create a common set of methods that multiple classes can implement, promoting code reuse and supporting a high level of abstraction. It’s a key feature of Java’s support for multiple inheritance and helps achieve a more flexible and modular design in object-oriented programming.
Example:
interface Polygon {
void getArea(int length, int breadth);
}
// implement the Polygon interface
class Rectangle implements Polygon {
// implementation of abstract method
public void getArea(int length, int breadth) {
System.out.println(“The area of the rectangle is: ” + (length * breadth));
}
}
class Main {
public static void main(String[] args) {
Rectangle r1 = new Rectangle();
r1.getArea(5, 6);
}
}
Output:
The area of the rectangle is: 30
In the above example, we have created an interface named Polygon. The interface contains an abstract method getArea().
Here, the Rectangle class implements Polygon. And, provides the implementation of the getArea() method.
b. What is type casting? How can we do type casting in Java. Explain.
Ans: Type casting in Java refers to the process of converting a variable or expression from one data type to another. This conversion can be necessary when you want to assign a value of one data type to a variable of another data type, or when you want to perform operations that involve different data types.
There are two types of type casting in Java: implicit casting (widening) and explicit casting (narrowing). Implicit casting is done automatically by the compiler, while explicit casting requires the programmer to explicitly specify the desired type.
Implicit Casting (Widening):
- Implicit casting occurs when the conversion is done automatically by the compiler.
- It is also known as widening because it involves converting a smaller data type to a larger data type.
- It is generally safe because it does not result in loss of data.
int intValue = 10;
long longValue = intValue; // Implicit casting from int to long
Explicit Casting (Narrowing):
- Explicit casting is performed manually by the programmer.
- It is also known as narrowing because it involves converting a larger data type to a smaller data type.
- It may result in data loss if the value being converted cannot be represented in the target data type.
Explicit casting is done by placing the target data type in parentheses before the value or expression to be cast.
double doubleValue = 10.5;
int intValue = (int) doubleValue; // Explicit casting from double to int
Programming Example:
public class TypeCastingExample {
public static void main(String[] args) {
// Implicit casting (Widening)
int intValue = 100;
long longValue = intValue; // Automatically cast int to long
float floatValue = longValue; // Automatically cast long to float
double doubleValue = floatValue; // Automatically cast float to double
System.out.println(“Implicit Casting:”);
System.out.println(“int to long: ” + longValue);
System.out.println(“long to float: ” + floatValue);
System.out.println(“float to double: ” + doubleValue);
// Explicit casting (Narrowing)
doubleValue = 123.456;
floatValue = (float) doubleValue; // Explicitly cast double to float
longValue = (long) floatValue; // Explicitly cast float to long
intValue = (int) longValue; // Explicitly cast long to int
System.out.println(“\nExplicit Casting:”);
System.out.println(“double to float: ” + floatValue);
System.out.println(“float to long: ” + longValue);
System.out.println(“long to int: ” + intValue);
}
}
c. What is user defined exception? Explain user defined exception with suitable Java program.
Ans: In Java, a user-defined exception is an exception that is created by the programmer to handle specific situations in a program. While Java provides a set of built-in exceptions that cover common scenarios, there are cases where you might want to create your own exception class to represent a custom exception situation that is specific to your application.
Here’s an example of creating a user-defined exception class in Java:
// User-defined exception class
class MyCustomException extends Exception {
// Constructor with a custom error message
public MyCustomException(String message) {
super(message);
}
}
// Example class using the user-defined exception
public class UserDefinedExceptionExample {
// Method that throws the user-defined exception
public static void validateAge(int age) throws MyCustomException {
// Custom validation logic
if (age < 0) {
throw new MyCustomException(“Age cannot be negative”);
}
}
public static void main(String[] args) {
try {
// Call the method that may throw the user-defined exception
validateAge(-5);
} catch (MyCustomException e) {
// Catch and handle the user-defined exception
System.out.println(“Exception caught: ” + e.getMessage());
}
}
}
In this example:
MyCustomException class:
- MyCustomException is a user-defined exception class that extends the built-in Exception class.
- It has a constructor that takes a custom error message as a parameter and passes it to the constructor of the superclass (Exception) using super(message).
validateAge method:
- The validateAge method checks if the provided age is negative.
- If the age is negative, it throws an instance of MyCustomException with a custom error message.
UserDefinedExceptionExample class:
- The main method of this class calls validateAge with a negative age to intentionally trigger the user-defined exception.
- The exception is caught using a try-catch block, and the custom error message is printed.
Output:
Exception caught: Age cannot be negative
6. Answer the following questions.
a. What is a package? Can we create a user defined package in Java? If so, how? Explain with suitable java code.
Ans: A package in Java is a way to organize related classes and interfaces into a single unit. It helps in avoiding naming conflicts, provides a mechanism for access control, and supports modularization of code. Packages are used to group related classes and make the code more manageable.
Creating a User-Defined Package in Java:
Yes, we can create user-defined packages in Java. To create a user-defined package, we have to follow these steps:
Create a Directory Structure: Create a directory with the package name.
Write Java Classes: Write Java classes and place them in the appropriate directory structure.
Package Declaration: Include a package statement at the beginning of each class file to specify the package name.Declare the package at the beginning of a file using the form:
package packagename;
Compilation: Compile the Java files using the ‘javac’ command. The compiler will generate corresponding class files in the package directory.
Use the package: We can use the classes from the user-defined package in other Java files by importing the package. In other Java files, use the ‘import’ statement to access classes from the user-defined package.
Here’s an example to illustrate the creation of a user-defined package and use it:
// File: myPackage/MyClass1.java
package myPackage;
public class MyClass {
public void display() {
System.out.println(“This is MyClass1”);
}
}
// File: myPackage/MyClass2.java
package myPackage;
public class MyClass2 {
public void show() {
System.out.println(“This is MyClass2”);
}
}
//File: PackageTest.java
import myPackage.MyClass1;
import myPackage.MyClass2;
public class PackageTest {
public static void main(String[] args) {
MyClass1 obj1=new MyClass1();
Obj1.display();
MyClass2 obj2=new MyClass2();
Obj2.show();
}
}
b. Explain the concept of method overriding with suitable Java code.
Ans: Method overriding is a feature in object-oriented programming where a subclass provides a specific implementation for a method that is already defined in its superclass. In other words, a subclass can replace or override the implementation of a method that it inherits from its superclass. To perform method overriding, the method in the subclass must have the same signature (name, return type, and parameter types) as the method in the superclass.
Method Overriding Example:
class Animal {
public void displayInfo() {
System.out.println(“I am an animal.”);
}
}
class Dog extends Animal {
@Override
public void displayInfo() {
System.out.println(“I am a dog.”);
}
}
class Override{
public static void main(String[] args) {
Dog d1 = new Dog();
d1.displayInfo();
}
}
Output:
I am a dog.
In the above program, the displayInfo()
method is present in both the Animal superclass and the Dog subclass.
When we call displayInfo() using the d1 object (object of the subclass), the method inside the subclass Dog is called. The displayInfo() method of the subclass overrides the same method of the superclass.
c. Mention the package name to which the following class belong.
(i) DataInputstream class
(ii) Scanner Class
(iii) BufferedReader Class
(iv) System Class
Ans: In Java, classes are organized into packages to provide a logical structure and avoid naming conflicts. Here are the package names for the mentioned classes:
i. DataInputStream class:
- Package Name: java.io
ii. Scanner Class:
- Package Name: java.util
iii. BufferedReader Class:
- Package Name: java.io
iv. System Class:
- Package Name: java.lang
7. Answer the following questions.
a. What is an abstract class? What are the rules of an abstract class?
Ans: An abstract class in object-oriented programming (OOP) is a class that cannot be instantiated on its own and may contain abstract methods, which are methods without a defined implementation. Abstract classes are meant to serve as blueprints for other classes, providing a common interface and structure for their subclasses.
Here are some rules and characteristics of abstract classes:
Abstract classes in Java have certain rules and characteristics that define their behavior.
Cannot be instantiated: We cannot create an instance (object) of an abstract class directly. Abstract classes are meant to be subclassed by other classes.
May contain abstract methods: Abstract classes can have abstract methods, which are declared without providing an implementation. Subclasses must provide concrete implementations for these abstract methods.
May contain concrete methods: Abstract classes can also have regular (concrete) methods with an implementation. Subclasses inherit these methods but can override them if needed.
Can contain constructors: Abstract classes can have constructors. These constructors are typically used to initialize the common properties of the abstract class.
Can contain fields (variables): Abstract classes can have instance variables, just like regular classes. These fields are often used to store common data that may be shared among subclasses. These fields may be private, protected or public, depending on the design.
Cannot be marked as final: An abstract class cannot be marked as final because the purpose of an abstract class is to be subclassed. A final class cannot be subclassed.
May Extend Another Class (Abstract or Concrete): An abstract class can extend another abstract or non-abstract class.
Subclasses Must Implement Abstract Methods: If a subclass of an abstract class does not provide concrete implementations for all the abstract methods defined in its abstract superclass, it must also be declared as abstract.
b. Explain how can we use the class “DataInputStream” for interactive inputs in a Java program.
Ans: The DataInputStream class in Java is part of the java.io package and is used for reading primitive data types from an input stream. While it’s not commonly used for interactive inputs, it can be adapted for this purpose. The more commonly used approach for interactive inputs is to use the Scanner class.
Here’s a simple example of how you can use DataInputStream for interactive inputs:
import java.io.DataInputStream;
import java.io.IOException;
import java.io.InputStream;
public class InteractiveInputExample {
public static void main(String[] args) {
// Create a DataInputStream object for reading from the console
DataInputStream dataInputStream = new DataInputStream(System.in);
try {
// Prompt the user for input
System.out.print(“Enter your name: “);
// Read a line of input as a String
String name = dataInputStream.readLine();
// Display the input
System.out.println(“Hello, ” + name + “!”);
// Close the DataInputStream (not mandatory, but good practice)
dataInputStream.close();
} catch (IOException e) {
System.err.println(“Error reading input: ” + e.getMessage());
}
}
}
Here, we create DataInputStream object (dataInputStream) using System.in as the underlying InputStream. We then use the readLine() method to read a line of input from the user.
using DataInputStream for interactive input is not the most common or recommended approach in modern Java programming. The Scanner class provides a more user-friendly and flexible way to handle interactive inputs.
c. What is inheritance in java? What are the different types of inheritance possible? Explain how we can achieve Hierarchical Inheritance in java.
Ans: Inheritance is a fundamental concept in object-oriented programming (OOP) that allows a new class to inherit properties and behaviors (fields and methods) of an existing class. This promotes code reusability and the creation of a hierarchy of classes. In Java, inheritance is implemented using the extends keyword. The class that is being inherited from is called the superclass or base class or parent class, and the class that inherits from it, is called the subclass or derived class or child class.
There are several types of inheritance in Java:
Single Inheritance: In single inheritance, a subclass can inherit from only one superclass.
class Parent {
// parent class members
}
class Child extends Parent {
// child class members
}
Multilevel Inheritance: In multilevel inheritance, a class can inherit from another class, and then another class can inherit from the second class. This creates a chain of inheritance, where each class inherits from the class preceding it. In simple terms, it involves a chain of inheritance with multiple levels. In multilevel inheritance, a subclass becomes a superclass for another class.
class Grandparent {
// grandparent class members
}
class Parent extends Grandparent {
// parent class members
}
class Child extends Parent {
// child class members
}
Hierarchical Inheritance: In hierarchical inheritance, multiple classes inherit from a single superclass. It forms a tree-like structure. Hierarchical Inheritance allows multiple classes to share common characteristic from a single superclass while allowing each subclass to introduce its own specific behavior.
class Animal {
// animal class members
}
class Dog extends Animal {
// dog class members
}
class Cat extends Animal {
// cat class members
}
Here, both Dog and Cat classes inherit from the common Animal class.
To achieve Hierarchical Inheritance in Java:
class Animal {
void eat() {
System.out.println(“Animal is eating”);
}
}
class Dog extends Animal {
void bark() {
System.out.println(“Dog is barking”);
}
}
class Cat extends Animal {
void meow() {
System.out.println(“Cat is meowing”);
}
}
public class HierarchicalInhertance
{
public static void main(String[] args)
{
Dog myDog=new Dog();
myDog.eat();
myDog.bark();
Cat myCat=new Cat();
myCat.eat();
myCat.meow();
}
}
In this example, the Dog and Cat classes inherit from the common Animal class. They share the eat() method from the Animal class, and each of them has its own specific methods (bark() and meow()). This represents hierarchical inheritance in Java.