JAVA’s History:
Java is a general purpose , Object Oriented programming language. It is a powerful, versatile, and simple programming language. It is one of the most widely used high-level programming languages in the world.
Java developed by James Gosling at Sun Microsystems of USA in 1991. The original name of Java was Oak but it was renamed to Java in 1995 as OAK was a registered trademark of another Tech company.
Sun Microsystems started a project called ‘Green’ in 1991, mot knowing that their project would lead them to develop a programming language called Java. The Green project was born as part of a larger project to develop advanced software for consumer electronic devices like TVs, VCRs, toasters and such other electronic machines. The plan was to develop the project in C++. The project team which included Patrick Naughton discovered that the existing languages like C and C++ had limitations. The developers ran into many problems as they tried to extend the C++ compiler. They needed a new language to develop this project. James Gosling began work on a new language called Oak. Later it was called Java because Oak failed the trade mark search.
Byte Code:
Bytecode in Java is a set of instructions for the Java Virtual Machine. Java Virtual Machine, abbreviated as JVM, enables a computer to run code written in Java. When a Java program is compiled, the bytecode gets generated. Java Compiler produces byte code for JVM.
Bytecode is a machine-independent code. Code that runs on multiple computer architectures is called machine-independent code. It is not dependent on the properties of a specific machine, and can therefore be used on any machine. So basically it can run on any environment or system. It is a code that lies in between low-level language and high-level language. Since we write our code in high-level language, it gets compiled into bytecode and later JVM converts it into machine code which is a low level code hence we call bytecode as a code that lies between low-level language and high-level language. The bytecode can run on any device which makes it independent of the Operating System.
Java Virtual Machine (JVM):
The compiled Java byte code can run on any computer with the Java Runtime Environment (JRE) installed on it. The virtual machine code is not machine specific. The machine specific code (known as machine code) is generated by the Java Interpreter by acting as an intermediary between the virtual machine and the real machine.

The JVM plays an important role in making the Java language portable and platform independent as it hides the details of implementation between the hardware requirement and the operating system. The JVM has two primary functions: to allow Java programs to run on any device or operating system (known as the “Write once, run anywhere” principle), and to manage and optimize program memory.
Features of Java Programming
Java is a versatile, object-oriented programming language that is widely used for developing various types of applications. It comes with a rich set of features that contribute to its popularity and flexibility.
Here are some key features of Java programming:
Simple and Easy to Learn: Java was designed to be easy to use and understand, especially for developers familiar with C++. It eliminates the complexity of C++. Java does not use pointers, preprocessor header files, goto statement and many others. It also eliminate operator overloading and multiple inheritance.
Object-Oriented: Java is a fully object-oriented programming language, emphasizing the use of classes and objects. Almost everything in Java is an object. All concepts of OOP are maintained in Java. It follows the principles of encapsulation, inheritance, abstraction and polymorphism.
Platform Independence (Write Once, Run Anywhere – WORA): Java applications can run on any device that has a Java Virtual Machine (JVM). This platform independence is achieved by compiling Java code into an intermediate form called bytecode, which can be executed on any device with a compatible JVM.
Compiled and Interpreted: Java is both a compiled and an interpreted language. Usually, a computer language is either compiled or interpreted. Java combines both these approaches thus making Java a two-stage system. First Java compiler translates source code into bytecode. Bytecodes are not machine instructions and therefore, in the second stage, java interpreter generates machine code that can be directly executed by the machine that is running the Java program. We can thus say that Java is both a compiled and an interpreted language.
Portable: The portability aspect of Java refers to the ability of Java programs to be executed on different platforms without modification. The most significant contribution of Java over other languages is its portability. Java programs can be easily moved from one computer system to another, anywhere and anytime. Changes and upgrades in operating systems, processors and system resources will not force any changes in Java programs.
Distributed: Java is designed as a distributed language for creating applications on networks. It has the ability to share both data and programs. Java applications can open and access remote objects on Internet as easily as they can do in a local system. This enables multiple programmers at multiple remote locations to collaborate and work together on a single project. Java supports distributed computing through its built-in features like Remote Method Invocation (RMI) and Java Remote Method Protocol (JRMP). These allow objects to invoke methods on remote machines, making it easier to develop distributed applications.
Multithreaded and Interactive: Multithreaded means handling multiple tasks simultaneously. Java supports multithreaded programs. This means that we need not wait for the application to finish one task before beginning another. Java’s multithreading feature allows the execution of multiple threads simultaneously. This is essential for developing applications that require concurrent processing and improved performance. The Java runtime comes with tools that support multiprocess synchronization and construct smoothly running interactive systems.
Dynamic and Extensible: Java is dynamic and extensible, supporting dynamic loading of classes. This feature enables the extension of applications by loading classes dynamically at runtime. Java is capable of dynamically linking in new class libraries, methods and objects.
Automatic Memory Management (Garbage Collection): Java’s automatic garbage collection feature helps in managing memory efficiently, reducing the chances of memory leaks and memory-related errors. Java has an automatic garbage collector that manages memory allocation and deallocation. This helps in preventing memory leaks and makes memory management more efficient.
Robust and Secure: Java is robust and secure programming language due to several built-in features and design principles. It provides many safeguards to ensure reliable code. Java enforces strong type checking at compile-time, reducing the likelihood of type-related errors during runtime. It has a robust exception-handling mechanism that helps developers manage runtime errors effectively. This prevents unexpected crashes and allows for graceful error recovery.
Java includes a Security Manager that allows developers to define and enforce security policies. This provides fine-grained control over the actions that code can perform. The Java Virtual Machine (JVM) verifies bytecode before execution, ensuring that it adheres to Java’s safety rules. This helps prevent the execution of potentially harmful or malformed code.
Rich Standard Library: Java comes with a vast standard library that provides a wide range of pre-built functionality, making it easier for developers to perform common tasks without having to write code from scratch.
High Performance: Java has many features that makes it a high-performance language, including its compiler and runtime system. Java offers good performance through its Just-In-Time (JIT) compilation, which translates bytecode into native machine code at runtime. Java architecture is also designed to reduce overheads during runtime. Further, the incorporation of multithreading enhances the overall execution speed of Java programs.
Data Types of Java:
Each variable in Java has a data type that decides what type of value the variable will hold. Data types specify the size and type of data that can be stored inside variables. Java language is rich in its data types. The varieties of data types available allow the programmer to select the type appropriate to the needs of the application.
Java is a strongly typed language. This means that every variable must have a declared type. A data type is an attribute of a variable which tells the compiler or interpreter how the programmer intends to use the variable. It defines the operations that can be done on the data and what type of values can be stored.
A data type defines the set of values that an expression can produce or a variable can contain. The data type of a variable or expression also defines the operations that can be performed on the variable or expression.
Data types in Java are mainly divided into two categories:
- Primitive Data Types
- Non-Primitive Data Types
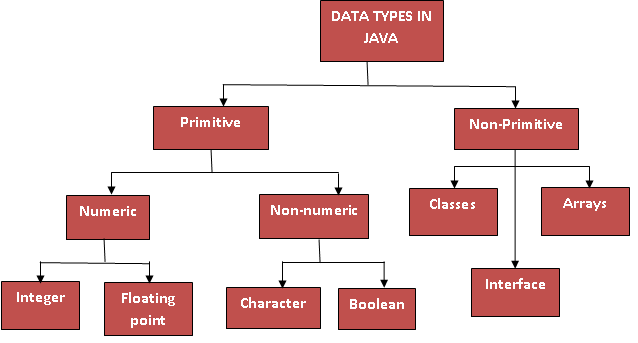
Primitive Data Types:
A primitive data type represents a single value, such as a number, a character, or a Boolean value. A primitive data type is pre-defined by the programming language. The primitive types are self-contained values that can be contained in a variable. The primitive types are comprised of integer types, floating-point types and the boolean type.
Integer Types:
Integer types can hold whole numbers such as 112, -96 and 6535. The size of the values that can be stored depends on the integer data type we choose. Java supports four types of integers as shown in figure below. They are byte, short, int and long.
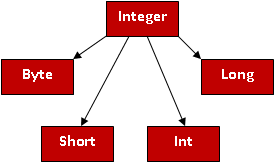
Fig: Integer data types
Floating Point Types:
Floating-point types can hold numbers containing fractional parts such as 25.69 and -2.625. There are two kinds of floating point storage as shown in figure below. The floating-point data types are float and double.

Fig: Floating point data types
Character Type:
In order to store character constants in memory, Java provides a character data type called char. The char type assumes a size of 2 bytes but, basically, it can hold only a single character.
Boolean Type:
In Java, the boolean data type represents two values: true and false. Boolean type is used when we want to test a particular condition during the execution of the program. There are only two values that a Boolean type can take: true or false. Boolean type is denoted by the keyword Boolean and uses only one bit of storage.
All comparison operators return Boolean type values. Boolean values are often used in selection and iteration statements. The words true and false cannot be used as identifiers.
Variables:
Variables are named storage locations in memory. A variable is just a memory location that has been given a name so that it can be easily referred to and used in a program. The programmer only has to worry about the name; it is the compiler’s responsibility to keep track of the memory location.
A variable is an identifier that denotes a storage location used to store a data value. The value stored in a variable can be changed during program execution. A variable’s value is changed by an assignment operator or by a prefix or postfix ++ (increment) or – – (decrement) operator.
Variable is like a container (storage area) with a name in which we can store data. Variable names are just the symbolic representation of a memory location. A variable is a storage location and has an associated type. In Java, every variable has a type. All variables must be declared before use. We declare a variable by placing the type first, followed by the name of the variable.
Here are some examples:
int roll;
long population;
double salary;
char grade;
boolean valid;
Rules for Naming a variable:
There are some rules on choosing variable names. The following rules must be followed while naming variables:
- A variable name consists of letters (both uppercase and lowercase letters), digits, the underscore ( _ ) and dollar character.
- A variable name must begin with a letter, and must be a sequence of letters or digits.
- Variable name must not begin with digit.
- Variable names are case-sensitive. The compiler treats the upper and lower case letters as different.
- No whitespace is allowed within the variable name.
- Special symbols such as period, semicolon, comma, slash etc. are not allowed.
- Any reserved word (keyword) cannot be used as a variable name. e.g. int, float etc.
Declaring (Creating) Variables:
In Java, variables are the names of memory locations. A variable must be declared before it is used in the program. We declare a variable by placing the type first, followed by the name of the variable.
Declaration does the three things:
- It tells the compiler what the variable name is
- It specifies what type of data the variable will hold.
- The place of declaration (in the program) decides the scope of of the variable.
Variable names should be user friendly. The name should be chosen to describe the role that the variable is designed to play e.g., Emp_no for employee number. A meaningful name given to a variable always helps in better understanding of the program.
Variable declaration begins with the data type, followed by the name of one or more variables. All declaration statement must end with a semicolon.
Variable declaration syntax:
data_type variable-name;
Where data_type is data type and variable-name is the name of the variable.
Example:
int age;
float fees;
To declare more than one variable of the specified type, use a comma separated list.
Syntax:
type variable1,variable2,…variableN;
Example:
int x,y,z;
char x1,x2,x3;
Variable Initialization/ Initializations of Variables:
Variable initialization means assigning values to the variables. The process of giving initial values to variables is known as the initialization. The variables that are not initialized are set to zero. Java variables can be initialized with the help of assignment operator.
Java Operators:
An operator can be defined as a symbol that specifies an operation to be performed. For example: +, -, *, / etc.
An operator is a symbol that tells the compiler to perform certain mathematical or logical computations.
Java divides the operators into the following categories:
- Arithmetic operators
- Assignment operators
- Relational (Comparison) operators
- Logical operators
- Increment and decrement operators
- Conditional operators
- Bitwise operators
Arithmetic Operators:
The arithmetic operators are used to perform mathematical calculations such as addition, subtraction etc. Java supports all the basic arithmetic operators.
Operator |
Meaning |
+ |
Addition |
– |
Subtraction |
* |
Multiplication |
/ |
Division |
% |
Modulus (Remainder) |
These operators can operate on any built–in data types such as int, char and float. However, the modulus operator requires an integral operand (must not be float).
Assignment Operator:
An assignment operator is used to assign a value to a variable or to assign the result of an expression to a variable. The = symbol is used as an assignment operator.
Or simply we can say, a data or value can be stored in a variable with the help of assignment operator. The assignment operator is used in assignment statement and assignment expression.
The syntax of an assignment is,
Variable name=expression;
For example, a=5;
Sum=a+b;
In many cases, the assignment operator can be combined with other operators to build a shorter version of the statement called a Compound Statement. For example, instead of a = a+5, we can write a += 5.
Relational Operators:
These operators are used to check for relations like equality, greater than, and less than. They return boolean results after the comparison and are extensively used in looping statements as well as conditional if-else statements.
These are used to compare the values two variable or constants. The relational operators are symbols that are used to test the relationship between variables or between a variable and a constant. For example,
(salary==5000)
Here == is the operator that is used to test equality.
There are six relational operators provided by Java. They are:
Operator |
Meaning |
= = |
equal to |
!= |
not equal to |
> |
greater than |
< |
less than |
>= |
greater than or equal to |
<= |
less than or equal to |
Logical Operators:
Logical operators are symbols that are used to combine two or more expressions containing relational operators. This situations will arise when we want to perform a statement block on a combined condition, like x>5 and x<10. Then we code this using an AND operator. The AND operator is represented as &&.
Example:
((x>5)&&(x<10))
(Salary>=10000&&salary<=20000)
The logical operators are used to combine conditions. These operators are used with one or more operand and return either value zero (for false) or one (for true). The operand may be constant, variables or expressions. And the expression that combines two or more expressions is termed as logical expression.
Java has three logical operators:
Operator |
Meaning |
&& |
AND |
|| |
OR |
! |
NOT |
Increment and decrement Operators:
The increment and decrement operators are two very useful operator used in Java. Both increment and decrement operator are used on a single operand and variable, so it is called as a unary operator.
Increment operator:
Increment operators are used to increase the value of a variable by 1. This operator is represented by ++ symbol. The increment operator can either increase the value of the variable by 1 before assigning it to the variable or can increase the value of the variable by 1 after assigning the variable. Thus it can be classified into two types:
1.Pre-increment operator
2.Post-increment operator
Pre-increment operator (Prefix increment operator):
A pre-increment operator is used to increment the value of a variable before using it in an expression. In the pre-increment, first increment the value of variable and then used inside the expression.
Syntax: ++variable;
For example:
x=++i;
In this case, the value of i will be incremented first by 1. Then the new value will be assigned to x for the expression in which it is used.
Post –increment operator (Postfix increment operator):
A post increment operator is used to increment the value of the variable after executing the expression completely in which post increment is used. In the post-increment, first value of variable is used in the expression and then increment the value of variable.
Syntax: variable++
For example:
x=i++;
In this case, the value of i is first assigned to x and after that, i is incremented.
Decrement Operators:
We use decrement operators in C to decrement the given value of a variable by 1. This operator is represented by – – symbol.
Decrement operator can be classified into two types:
1.Pre-decrement operator
2.Post-decrement operator
Pre-decrement operator (Prefix-decrement operators):
The pre-decrement operator first decrement the value of variable and then used inside the expression.
Syntax: – – variable;
Example:
x=- – i;
Here, value of i will decrement first by 1 and then assign it to the variable x.
Post-decrement operator (Postfix-decrement operators):
In post-decrement first value of variable is used in the expression and then decrement the variable.
Syntax: variable – –
Example: x=i- -;
Here, the value of i is first assigned to x and after that, value of i is decremented.
Conditional Operators:
Sometimes we need to choose between two expressions based on the truth value of a third logical expression. The conditional operator can be used in such cases. It sometimes called as ternary operator. Since it required three expressions as operand and it is represented as (? :).
Syntax: exp1 ? exp2 :exp3;
Here exp1 is first evaluated. It is true then value return will be exp2 . If false then exp3.
Example: c=(a>b)?a:b;
Where, a and b are operands
?: is the conditional operator
> is the relational operator
If the condition is true then value a is assigned to c otherwise b is assigned to c.
Bitwise Operators:
For manipulating data at the bit level C provides us special operators known as bitwise operators. The bitwise operators are the operators used to perform the operations on the data at the bit level. These operators are used to perform bitwise operations such as for testing the bits, shifting the bits to left or right, one’s complement of bits etc.
Various bitwise operators in Java:
Operator |
Meaning |
~ |
one’s complement |
<< |
Shift left |
>> |
Shift right |
& |
Bitwise AND |
| |
Bitwise OR |
^ |
Bitwise XOR (Exclusive OR) |
Arrays:
An array is a collection of similar data elements stored at contiguous memory locations. This data type is useful when a group of elements are to be represented by a common name. An array is used to store a group of data items that belong to the same data type.
An array is a group of elements that share a common name, and is differentiated from one another by their positions within the array. An array is also defined as a collection of homogeneous data.
The array is the simplest data structure where each data element can be randomly accessed by using its index number. Array index always start from zero.
Array is beneficial if we have to store similar elements. For example, 70,78,80,95, 90 and 98 are marks in 6 subjects of a student. To store these 6 marks 6 variables would be required- mark1, mark2, mark3, mark4, mark5 and mark6. For such a representation, arrays are useful. This can be represented as mark[6]. We don’t need to define different variables for the marks in the different subject. Instead of that, we can define an array which can store the marks in each subject at the contiguous memory locations.
Types of Array in java:
There are two types of array.
- Single Dimensional Array
- Multidimensional Array
OOP (Object Oriented Programming) Fundamentals:
OOP is a programming model based on the concept of objects and classes. It is a methodology or paradigm to design a program using classes and objects. The four principles of object-oriented programming are encapsulation, abstraction, inheritance and polymorphism.
Abstraction: Hiding internal details and showing functionality is known as abstraction. For example phone call, we don’t know the internal processing. We all know how to turn the TV on, but we don’t need to know how it works in order to enjoy it.
Encapsulation: Binding (or wrapping) code and data together into a single unit are known as encapsulation. For example, a capsule, it is wrapped with different medicines. Encapsulation is a protective barrier that keeps the data and code safe within the class itself.
Inheritance: A special feature of Object-Oriented Programming in Java. In object-oriented programming, inheritance is a mechanism where programmers can derive a class from another class. Inheritance lets programmers create new classes that share some of the attributes of existing classes. Inheritance also allows programmers to reuse previously written codes. This removes the burden of writing the same codes again, as programmers can make a derived class inherit the property of its parent class.
Polymorphism: If one task is performed in different ways, it is known as polymorphism. Polymorphism allows programmers to use the same word in Java to mean different things in different contexts. One form of polymorphism is method overloading. That’s when the code itself implies different meanings. The other form is method overriding. That’s when the values of the supplied variables imply different meanings.
Class and Objects:
Class: Class is the core of Java language. It can be defined as a template that describes the behaviors and states of a particular entity. According to OOPs concept in Java, a class is the blueprint/template of an object. It contains the similar types of objects having the same states (properties) and behavior.
In other words, a class can also be defined as “a class is a group of objects which are common to all objects of one type”. A class is a blueprint or prototype from which objects are created.
A class is defined as a template of data and methods that manipulate the data. For example, if we consider a template of the fan, then,
Data: Number of wings, type of motor, color.
Methods: on, off, rotate at various speeds.
A class is also defined as a blue print or a pattern that defines the data and the methods that operate on this data. Using this blueprint, objects are created. Using the same blueprint many objects can be created. All these objects are similar in their data content as well as their methods. The data content represents the attributes/properties or state of the object and the methods represent the behavior of the object.
A class is a model to create objects. It means that we write properties and actions of objects in the class.
In Java, to declare a class class keyword is used. A class contains both data and methods that operate on that data. The data or variables defined within a class are called instance variables and the code that operates on this data is known as methods. Thus, the instance variables and methods are known as class members.
Classes are also known as user-defined data types. A class defines new data type. Once defined, this new type can be used to create object of that type.
Suppose we create a class “Student” having probable attributes – student’s name, address, roll number and other related parameters.
So, students like Raja, Rishi, Rohit, etc., will be the objects of a class Student having the same properties like student’s name, address, roll number, etc.
Object: Objects are the building blocks of OOP languages. Object is an instance of a class while class is a blueprint of an object. An object represents the class and consists of properties and behavior.
An object is a basic unit of an object-oriented programming language. It is any real-world thing that has properties and actions. In other words, an entity that has state and behavior is known as object in Java. Here, the state represents properties and behavior represents actions or functionality.
In real world, we can understand object as a cell phone that has its properties like: name, cost, color etc and behavior like calling, chatting etc.
For example, book, pen, pencil, TV, fridge, washing machine, mobile phone, etc.
All objects have three characteristics:
Identity: Any object that exists must have an identity i.e., an object is identified by a name.
Identity represents the unique name of an object. It differentiates one object from the other. The unique name of an object is used to identify the object.
State: State represents properties or attributes of an object. To say that an object has state means that a particular object has properties that can change over its lifetime.
Behavior: What the object can do. Every object knows how to do something. Different objects may have different capabilities. The airplane object can fly, the boat object can sail, the fan object can rotate.
Behavior represents functionality or actions. It is represented by methods in Java.
Method Overloading:
In Java it is possible to define two or more methods within the same class having same name, with different parameter declaration. This is known as method overloading.
Constructor:
A Constructor in Java is a special method, which has the same name as the class. They have no return type, not even void. Constructors are similar to methods, but they are used to initialize an object. The constructor is invoked automatically when a new class object is instantiated.
In Java, a constructor is a block of codes similar to the method. It is called when an instance of the class is created. At the time of calling the constructor, memory for the object is allocated in the memory. It is a special type of method which is used to initialize the object. Every time an object is created using the new() keyword, at least one constructor is called.
Syntax:
Following is the syntax of a constructor:
Class ClassName
{
ClassName()
{
}
}
Types of Constructor:
Primarily there are two types of constructors in java:
· Default constructor
· Parameterized Constructor
Default Constructor: A constructor that has no parameter is known as the default constructor. If we don’t define a constructor in a class, then the compiler creates a default constructor (with no arguments)for the class.
Parameterized Constructor: A constructor that has parameters is known as parameterized constructor. If we want to initialize fields of the class with our own values, then use a parameterized constructor.
Inheritance in Java:
Inheritance is defined as the creation of a new class from an existing class to provide better features. It is a mechanism in which one class acquires the property of another class. The parent class is called a super class and the inherited class is called a subclass. The keyword extends is used by the sub class to inherit the features of super class.
Inheritance is important since it leads to the reusability of code. In Java, when an “Is-A” relationship exists between two classes, we use Inheritance.
Java Inheritance Syntax:
class subClass extends superClass
{
//methods and fields
}
Types of Inheritance:
Single Inheritance:
In Single Inheritance one class extends another class (one class only). It is the simplest form of inheritance where a class inherits only one parent class. Single inheritance enables code reusability and adds new features to the existing class.
Multiple Inheritance:
Multiple Inheritance is one of the inheritance in Java types where one class extending more than one class. When a class inherits more than one parent class, it becomes a multiple inheritance. As the child class inherits properties from different parent classes, it has access to all of its objects. It is different from a single inheritance property, as it allows an object or class to inherit from more than one object or class. Java does not support multiple inheritance.
Multilevel Inheritance:
In Multilevel Inheritance, one class can inherit from a derived class. Hence, the derived class becomes the base class for the new class. When one class inherits properties from a derived class, it is multilevel inheritance. For example, class A extends class B and class B extends class C.
Hierarchical Inheritance:
In Hierarchical Inheritance, one class is inherited by many sub classes. Here, different child classes inherit a single parent class. For example, a parent class C can have three subclasses, D, E and F.
Hybrid Inheritance:
Hybrid inheritance is one of the inheritance types in Java which is a combination of Single and Multiple inheritances.
Super Keyword:
The super keyword is similar to “this” keyword.
The keyword super can be used to access any data member or methods of the parent class.
Super keyword can be used at variable, method and constructor level.
Syntax:
super.<method-name>();